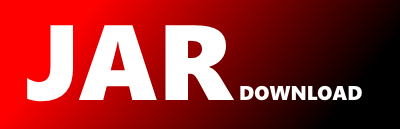
org.camunda.community.rest.client.dto.UserOperationLogEntryDto Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* UserOperationLogEntryDto
*/
@JsonPropertyOrder({
UserOperationLogEntryDto.JSON_PROPERTY_ID,
UserOperationLogEntryDto.JSON_PROPERTY_USER_ID,
UserOperationLogEntryDto.JSON_PROPERTY_TIMESTAMP,
UserOperationLogEntryDto.JSON_PROPERTY_OPERATION_ID,
UserOperationLogEntryDto.JSON_PROPERTY_OPERATION_TYPE,
UserOperationLogEntryDto.JSON_PROPERTY_ENTITY_TYPE,
UserOperationLogEntryDto.JSON_PROPERTY_CATEGORY,
UserOperationLogEntryDto.JSON_PROPERTY_ANNOTATION,
UserOperationLogEntryDto.JSON_PROPERTY_PROPERTY,
UserOperationLogEntryDto.JSON_PROPERTY_ORG_VALUE,
UserOperationLogEntryDto.JSON_PROPERTY_NEW_VALUE,
UserOperationLogEntryDto.JSON_PROPERTY_DEPLOYMENT_ID,
UserOperationLogEntryDto.JSON_PROPERTY_PROCESS_DEFINITION_ID,
UserOperationLogEntryDto.JSON_PROPERTY_PROCESS_DEFINITION_KEY,
UserOperationLogEntryDto.JSON_PROPERTY_PROCESS_INSTANCE_ID,
UserOperationLogEntryDto.JSON_PROPERTY_EXECUTION_ID,
UserOperationLogEntryDto.JSON_PROPERTY_CASE_DEFINITION_ID,
UserOperationLogEntryDto.JSON_PROPERTY_CASE_INSTANCE_ID,
UserOperationLogEntryDto.JSON_PROPERTY_CASE_EXECUTION_ID,
UserOperationLogEntryDto.JSON_PROPERTY_TASK_ID,
UserOperationLogEntryDto.JSON_PROPERTY_EXTERNAL_TASK_ID,
UserOperationLogEntryDto.JSON_PROPERTY_BATCH_ID,
UserOperationLogEntryDto.JSON_PROPERTY_JOB_ID,
UserOperationLogEntryDto.JSON_PROPERTY_JOB_DEFINITION_ID,
UserOperationLogEntryDto.JSON_PROPERTY_REMOVAL_TIME,
UserOperationLogEntryDto.JSON_PROPERTY_ROOT_PROCESS_INSTANCE_ID
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class UserOperationLogEntryDto {
public static final String JSON_PROPERTY_ID = "id";
private JsonNullable id = JsonNullable.undefined();
public static final String JSON_PROPERTY_USER_ID = "userId";
private JsonNullable userId = JsonNullable.undefined();
public static final String JSON_PROPERTY_TIMESTAMP = "timestamp";
private JsonNullable timestamp = JsonNullable.undefined();
public static final String JSON_PROPERTY_OPERATION_ID = "operationId";
private JsonNullable operationId = JsonNullable.undefined();
public static final String JSON_PROPERTY_OPERATION_TYPE = "operationType";
private JsonNullable operationType = JsonNullable.undefined();
public static final String JSON_PROPERTY_ENTITY_TYPE = "entityType";
private JsonNullable entityType = JsonNullable.undefined();
public static final String JSON_PROPERTY_CATEGORY = "category";
private JsonNullable category = JsonNullable.undefined();
public static final String JSON_PROPERTY_ANNOTATION = "annotation";
private JsonNullable annotation = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROPERTY = "property";
private JsonNullable property = JsonNullable.undefined();
public static final String JSON_PROPERTY_ORG_VALUE = "orgValue";
private JsonNullable orgValue = JsonNullable.undefined();
public static final String JSON_PROPERTY_NEW_VALUE = "newValue";
private JsonNullable newValue = JsonNullable.undefined();
public static final String JSON_PROPERTY_DEPLOYMENT_ID = "deploymentId";
private JsonNullable deploymentId = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_ID = "processDefinitionId";
private JsonNullable processDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_DEFINITION_KEY = "processDefinitionKey";
private JsonNullable processDefinitionKey = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_INSTANCE_ID = "processInstanceId";
private JsonNullable processInstanceId = JsonNullable.undefined();
public static final String JSON_PROPERTY_EXECUTION_ID = "executionId";
private JsonNullable executionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_CASE_DEFINITION_ID = "caseDefinitionId";
private JsonNullable caseDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_CASE_INSTANCE_ID = "caseInstanceId";
private JsonNullable caseInstanceId = JsonNullable.undefined();
public static final String JSON_PROPERTY_CASE_EXECUTION_ID = "caseExecutionId";
private JsonNullable caseExecutionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_TASK_ID = "taskId";
private JsonNullable taskId = JsonNullable.undefined();
public static final String JSON_PROPERTY_EXTERNAL_TASK_ID = "externalTaskId";
private JsonNullable externalTaskId = JsonNullable.undefined();
public static final String JSON_PROPERTY_BATCH_ID = "batchId";
private JsonNullable batchId = JsonNullable.undefined();
public static final String JSON_PROPERTY_JOB_ID = "jobId";
private JsonNullable jobId = JsonNullable.undefined();
public static final String JSON_PROPERTY_JOB_DEFINITION_ID = "jobDefinitionId";
private JsonNullable jobDefinitionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_REMOVAL_TIME = "removalTime";
private JsonNullable removalTime = JsonNullable.undefined();
public static final String JSON_PROPERTY_ROOT_PROCESS_INSTANCE_ID = "rootProcessInstanceId";
private JsonNullable rootProcessInstanceId = JsonNullable.undefined();
public UserOperationLogEntryDto() {
}
public UserOperationLogEntryDto id(String id) {
this.id = JsonNullable.of(id);
return this;
}
/**
* The unique identifier of this log entry.
* @return id
**/
@javax.annotation.Nullable
@JsonIgnore
public String getId() {
return id.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getId_JsonNullable() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
public void setId_JsonNullable(JsonNullable id) {
this.id = id;
}
public void setId(String id) {
this.id = JsonNullable.of(id);
}
public UserOperationLogEntryDto userId(String userId) {
this.userId = JsonNullable.of(userId);
return this;
}
/**
* The user who performed this operation.
* @return userId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getUserId() {
return userId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_USER_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getUserId_JsonNullable() {
return userId;
}
@JsonProperty(JSON_PROPERTY_USER_ID)
public void setUserId_JsonNullable(JsonNullable userId) {
this.userId = userId;
}
public void setUserId(String userId) {
this.userId = JsonNullable.of(userId);
}
public UserOperationLogEntryDto timestamp(OffsetDateTime timestamp) {
this.timestamp = JsonNullable.of(timestamp);
return this;
}
/**
* Timestamp of this operation.
* @return timestamp
**/
@javax.annotation.Nullable
@JsonIgnore
public OffsetDateTime getTimestamp() {
return timestamp.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getTimestamp_JsonNullable() {
return timestamp;
}
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
public void setTimestamp_JsonNullable(JsonNullable timestamp) {
this.timestamp = timestamp;
}
public void setTimestamp(OffsetDateTime timestamp) {
this.timestamp = JsonNullable.of(timestamp);
}
public UserOperationLogEntryDto operationId(String operationId) {
this.operationId = JsonNullable.of(operationId);
return this;
}
/**
* The unique identifier of this operation. A composite operation that changes multiple properties has a common `operationId`.
* @return operationId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getOperationId() {
return operationId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_OPERATION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getOperationId_JsonNullable() {
return operationId;
}
@JsonProperty(JSON_PROPERTY_OPERATION_ID)
public void setOperationId_JsonNullable(JsonNullable operationId) {
this.operationId = operationId;
}
public void setOperationId(String operationId) {
this.operationId = JsonNullable.of(operationId);
}
public UserOperationLogEntryDto operationType(String operationType) {
this.operationType = JsonNullable.of(operationType);
return this;
}
/**
* The type of this operation, e.g., `Assign`, `Claim` and so on.
* @return operationType
**/
@javax.annotation.Nullable
@JsonIgnore
public String getOperationType() {
return operationType.orElse(null);
}
@JsonProperty(JSON_PROPERTY_OPERATION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getOperationType_JsonNullable() {
return operationType;
}
@JsonProperty(JSON_PROPERTY_OPERATION_TYPE)
public void setOperationType_JsonNullable(JsonNullable operationType) {
this.operationType = operationType;
}
public void setOperationType(String operationType) {
this.operationType = JsonNullable.of(operationType);
}
public UserOperationLogEntryDto entityType(String entityType) {
this.entityType = JsonNullable.of(entityType);
return this;
}
/**
* The type of the entity on which this operation was executed, e.g., `Task` or `Attachment`.
* @return entityType
**/
@javax.annotation.Nullable
@JsonIgnore
public String getEntityType() {
return entityType.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ENTITY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getEntityType_JsonNullable() {
return entityType;
}
@JsonProperty(JSON_PROPERTY_ENTITY_TYPE)
public void setEntityType_JsonNullable(JsonNullable entityType) {
this.entityType = entityType;
}
public void setEntityType(String entityType) {
this.entityType = JsonNullable.of(entityType);
}
public UserOperationLogEntryDto category(String category) {
this.category = JsonNullable.of(category);
return this;
}
/**
* The name of the category this operation was associated with, e.g., `TaskWorker` or `Admin`.
* @return category
**/
@javax.annotation.Nullable
@JsonIgnore
public String getCategory() {
return category.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CATEGORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCategory_JsonNullable() {
return category;
}
@JsonProperty(JSON_PROPERTY_CATEGORY)
public void setCategory_JsonNullable(JsonNullable category) {
this.category = category;
}
public void setCategory(String category) {
this.category = JsonNullable.of(category);
}
public UserOperationLogEntryDto annotation(String annotation) {
this.annotation = JsonNullable.of(annotation);
return this;
}
/**
* An arbitrary annotation set by a user for auditing reasons.
* @return annotation
**/
@javax.annotation.Nullable
@JsonIgnore
public String getAnnotation() {
return annotation.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ANNOTATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getAnnotation_JsonNullable() {
return annotation;
}
@JsonProperty(JSON_PROPERTY_ANNOTATION)
public void setAnnotation_JsonNullable(JsonNullable annotation) {
this.annotation = annotation;
}
public void setAnnotation(String annotation) {
this.annotation = JsonNullable.of(annotation);
}
public UserOperationLogEntryDto property(String property) {
this.property = JsonNullable.of(property);
return this;
}
/**
* The property changed by this operation.
* @return property
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProperty() {
return property.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROPERTY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProperty_JsonNullable() {
return property;
}
@JsonProperty(JSON_PROPERTY_PROPERTY)
public void setProperty_JsonNullable(JsonNullable property) {
this.property = property;
}
public void setProperty(String property) {
this.property = JsonNullable.of(property);
}
public UserOperationLogEntryDto orgValue(String orgValue) {
this.orgValue = JsonNullable.of(orgValue);
return this;
}
/**
* The original value of the changed property.
* @return orgValue
**/
@javax.annotation.Nullable
@JsonIgnore
public String getOrgValue() {
return orgValue.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ORG_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getOrgValue_JsonNullable() {
return orgValue;
}
@JsonProperty(JSON_PROPERTY_ORG_VALUE)
public void setOrgValue_JsonNullable(JsonNullable orgValue) {
this.orgValue = orgValue;
}
public void setOrgValue(String orgValue) {
this.orgValue = JsonNullable.of(orgValue);
}
public UserOperationLogEntryDto newValue(String newValue) {
this.newValue = JsonNullable.of(newValue);
return this;
}
/**
* The new value of the changed property.
* @return newValue
**/
@javax.annotation.Nullable
@JsonIgnore
public String getNewValue() {
return newValue.orElse(null);
}
@JsonProperty(JSON_PROPERTY_NEW_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getNewValue_JsonNullable() {
return newValue;
}
@JsonProperty(JSON_PROPERTY_NEW_VALUE)
public void setNewValue_JsonNullable(JsonNullable newValue) {
this.newValue = newValue;
}
public void setNewValue(String newValue) {
this.newValue = JsonNullable.of(newValue);
}
public UserOperationLogEntryDto deploymentId(String deploymentId) {
this.deploymentId = JsonNullable.of(deploymentId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this deployment.
* @return deploymentId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getDeploymentId() {
return deploymentId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_DEPLOYMENT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getDeploymentId_JsonNullable() {
return deploymentId;
}
@JsonProperty(JSON_PROPERTY_DEPLOYMENT_ID)
public void setDeploymentId_JsonNullable(JsonNullable deploymentId) {
this.deploymentId = deploymentId;
}
public void setDeploymentId(String deploymentId) {
this.deploymentId = JsonNullable.of(deploymentId);
}
public UserOperationLogEntryDto processDefinitionId(String processDefinitionId) {
this.processDefinitionId = JsonNullable.of(processDefinitionId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this process definition.
* @return processDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessDefinitionId() {
return processDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessDefinitionId_JsonNullable() {
return processDefinitionId;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_ID)
public void setProcessDefinitionId_JsonNullable(JsonNullable processDefinitionId) {
this.processDefinitionId = processDefinitionId;
}
public void setProcessDefinitionId(String processDefinitionId) {
this.processDefinitionId = JsonNullable.of(processDefinitionId);
}
public UserOperationLogEntryDto processDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = JsonNullable.of(processDefinitionKey);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to process definitions with this key.
* @return processDefinitionKey
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessDefinitionKey() {
return processDefinitionKey.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessDefinitionKey_JsonNullable() {
return processDefinitionKey;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY)
public void setProcessDefinitionKey_JsonNullable(JsonNullable processDefinitionKey) {
this.processDefinitionKey = processDefinitionKey;
}
public void setProcessDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = JsonNullable.of(processDefinitionKey);
}
public UserOperationLogEntryDto processInstanceId(String processInstanceId) {
this.processInstanceId = JsonNullable.of(processInstanceId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this process instance.
* @return processInstanceId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getProcessInstanceId() {
return processInstanceId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getProcessInstanceId_JsonNullable() {
return processInstanceId;
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_ID)
public void setProcessInstanceId_JsonNullable(JsonNullable processInstanceId) {
this.processInstanceId = processInstanceId;
}
public void setProcessInstanceId(String processInstanceId) {
this.processInstanceId = JsonNullable.of(processInstanceId);
}
public UserOperationLogEntryDto executionId(String executionId) {
this.executionId = JsonNullable.of(executionId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this execution.
* @return executionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getExecutionId() {
return executionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_EXECUTION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getExecutionId_JsonNullable() {
return executionId;
}
@JsonProperty(JSON_PROPERTY_EXECUTION_ID)
public void setExecutionId_JsonNullable(JsonNullable executionId) {
this.executionId = executionId;
}
public void setExecutionId(String executionId) {
this.executionId = JsonNullable.of(executionId);
}
public UserOperationLogEntryDto caseDefinitionId(String caseDefinitionId) {
this.caseDefinitionId = JsonNullable.of(caseDefinitionId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this case definition.
* @return caseDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getCaseDefinitionId() {
return caseDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CASE_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCaseDefinitionId_JsonNullable() {
return caseDefinitionId;
}
@JsonProperty(JSON_PROPERTY_CASE_DEFINITION_ID)
public void setCaseDefinitionId_JsonNullable(JsonNullable caseDefinitionId) {
this.caseDefinitionId = caseDefinitionId;
}
public void setCaseDefinitionId(String caseDefinitionId) {
this.caseDefinitionId = JsonNullable.of(caseDefinitionId);
}
public UserOperationLogEntryDto caseInstanceId(String caseInstanceId) {
this.caseInstanceId = JsonNullable.of(caseInstanceId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this case instance.
* @return caseInstanceId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getCaseInstanceId() {
return caseInstanceId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CASE_INSTANCE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCaseInstanceId_JsonNullable() {
return caseInstanceId;
}
@JsonProperty(JSON_PROPERTY_CASE_INSTANCE_ID)
public void setCaseInstanceId_JsonNullable(JsonNullable caseInstanceId) {
this.caseInstanceId = caseInstanceId;
}
public void setCaseInstanceId(String caseInstanceId) {
this.caseInstanceId = JsonNullable.of(caseInstanceId);
}
public UserOperationLogEntryDto caseExecutionId(String caseExecutionId) {
this.caseExecutionId = JsonNullable.of(caseExecutionId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this case execution.
* @return caseExecutionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getCaseExecutionId() {
return caseExecutionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CASE_EXECUTION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getCaseExecutionId_JsonNullable() {
return caseExecutionId;
}
@JsonProperty(JSON_PROPERTY_CASE_EXECUTION_ID)
public void setCaseExecutionId_JsonNullable(JsonNullable caseExecutionId) {
this.caseExecutionId = caseExecutionId;
}
public void setCaseExecutionId(String caseExecutionId) {
this.caseExecutionId = JsonNullable.of(caseExecutionId);
}
public UserOperationLogEntryDto taskId(String taskId) {
this.taskId = JsonNullable.of(taskId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this task.
* @return taskId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getTaskId() {
return taskId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TASK_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getTaskId_JsonNullable() {
return taskId;
}
@JsonProperty(JSON_PROPERTY_TASK_ID)
public void setTaskId_JsonNullable(JsonNullable taskId) {
this.taskId = taskId;
}
public void setTaskId(String taskId) {
this.taskId = JsonNullable.of(taskId);
}
public UserOperationLogEntryDto externalTaskId(String externalTaskId) {
this.externalTaskId = JsonNullable.of(externalTaskId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this external task.
* @return externalTaskId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getExternalTaskId() {
return externalTaskId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_TASK_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getExternalTaskId_JsonNullable() {
return externalTaskId;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_TASK_ID)
public void setExternalTaskId_JsonNullable(JsonNullable externalTaskId) {
this.externalTaskId = externalTaskId;
}
public void setExternalTaskId(String externalTaskId) {
this.externalTaskId = JsonNullable.of(externalTaskId);
}
public UserOperationLogEntryDto batchId(String batchId) {
this.batchId = JsonNullable.of(batchId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this batch.
* @return batchId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getBatchId() {
return batchId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_BATCH_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getBatchId_JsonNullable() {
return batchId;
}
@JsonProperty(JSON_PROPERTY_BATCH_ID)
public void setBatchId_JsonNullable(JsonNullable batchId) {
this.batchId = batchId;
}
public void setBatchId(String batchId) {
this.batchId = JsonNullable.of(batchId);
}
public UserOperationLogEntryDto jobId(String jobId) {
this.jobId = JsonNullable.of(jobId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this job.
* @return jobId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getJobId() {
return jobId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_JOB_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getJobId_JsonNullable() {
return jobId;
}
@JsonProperty(JSON_PROPERTY_JOB_ID)
public void setJobId_JsonNullable(JsonNullable jobId) {
this.jobId = jobId;
}
public void setJobId(String jobId) {
this.jobId = JsonNullable.of(jobId);
}
public UserOperationLogEntryDto jobDefinitionId(String jobDefinitionId) {
this.jobDefinitionId = JsonNullable.of(jobDefinitionId);
return this;
}
/**
* If not `null`, the operation is restricted to entities in relation to this job definition.
* @return jobDefinitionId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getJobDefinitionId() {
return jobDefinitionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_JOB_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getJobDefinitionId_JsonNullable() {
return jobDefinitionId;
}
@JsonProperty(JSON_PROPERTY_JOB_DEFINITION_ID)
public void setJobDefinitionId_JsonNullable(JsonNullable jobDefinitionId) {
this.jobDefinitionId = jobDefinitionId;
}
public void setJobDefinitionId(String jobDefinitionId) {
this.jobDefinitionId = JsonNullable.of(jobDefinitionId);
}
public UserOperationLogEntryDto removalTime(OffsetDateTime removalTime) {
this.removalTime = JsonNullable.of(removalTime);
return this;
}
/**
* The time after which the entry should be removed by the History Cleanup job. [Default format](https://docs.camunda.org/manual/7.21/reference/rest/overview/date-format/) `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
* @return removalTime
**/
@javax.annotation.Nullable
@JsonIgnore
public OffsetDateTime getRemovalTime() {
return removalTime.orElse(null);
}
@JsonProperty(JSON_PROPERTY_REMOVAL_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getRemovalTime_JsonNullable() {
return removalTime;
}
@JsonProperty(JSON_PROPERTY_REMOVAL_TIME)
public void setRemovalTime_JsonNullable(JsonNullable removalTime) {
this.removalTime = removalTime;
}
public void setRemovalTime(OffsetDateTime removalTime) {
this.removalTime = JsonNullable.of(removalTime);
}
public UserOperationLogEntryDto rootProcessInstanceId(String rootProcessInstanceId) {
this.rootProcessInstanceId = JsonNullable.of(rootProcessInstanceId);
return this;
}
/**
* The process instance id of the root process instance that initiated the process containing this entry.
* @return rootProcessInstanceId
**/
@javax.annotation.Nullable
@JsonIgnore
public String getRootProcessInstanceId() {
return rootProcessInstanceId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ROOT_PROCESS_INSTANCE_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getRootProcessInstanceId_JsonNullable() {
return rootProcessInstanceId;
}
@JsonProperty(JSON_PROPERTY_ROOT_PROCESS_INSTANCE_ID)
public void setRootProcessInstanceId_JsonNullable(JsonNullable rootProcessInstanceId) {
this.rootProcessInstanceId = rootProcessInstanceId;
}
public void setRootProcessInstanceId(String rootProcessInstanceId) {
this.rootProcessInstanceId = JsonNullable.of(rootProcessInstanceId);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UserOperationLogEntryDto userOperationLogEntryDto = (UserOperationLogEntryDto) o;
return equalsNullable(this.id, userOperationLogEntryDto.id) &&
equalsNullable(this.userId, userOperationLogEntryDto.userId) &&
equalsNullable(this.timestamp, userOperationLogEntryDto.timestamp) &&
equalsNullable(this.operationId, userOperationLogEntryDto.operationId) &&
equalsNullable(this.operationType, userOperationLogEntryDto.operationType) &&
equalsNullable(this.entityType, userOperationLogEntryDto.entityType) &&
equalsNullable(this.category, userOperationLogEntryDto.category) &&
equalsNullable(this.annotation, userOperationLogEntryDto.annotation) &&
equalsNullable(this.property, userOperationLogEntryDto.property) &&
equalsNullable(this.orgValue, userOperationLogEntryDto.orgValue) &&
equalsNullable(this.newValue, userOperationLogEntryDto.newValue) &&
equalsNullable(this.deploymentId, userOperationLogEntryDto.deploymentId) &&
equalsNullable(this.processDefinitionId, userOperationLogEntryDto.processDefinitionId) &&
equalsNullable(this.processDefinitionKey, userOperationLogEntryDto.processDefinitionKey) &&
equalsNullable(this.processInstanceId, userOperationLogEntryDto.processInstanceId) &&
equalsNullable(this.executionId, userOperationLogEntryDto.executionId) &&
equalsNullable(this.caseDefinitionId, userOperationLogEntryDto.caseDefinitionId) &&
equalsNullable(this.caseInstanceId, userOperationLogEntryDto.caseInstanceId) &&
equalsNullable(this.caseExecutionId, userOperationLogEntryDto.caseExecutionId) &&
equalsNullable(this.taskId, userOperationLogEntryDto.taskId) &&
equalsNullable(this.externalTaskId, userOperationLogEntryDto.externalTaskId) &&
equalsNullable(this.batchId, userOperationLogEntryDto.batchId) &&
equalsNullable(this.jobId, userOperationLogEntryDto.jobId) &&
equalsNullable(this.jobDefinitionId, userOperationLogEntryDto.jobDefinitionId) &&
equalsNullable(this.removalTime, userOperationLogEntryDto.removalTime) &&
equalsNullable(this.rootProcessInstanceId, userOperationLogEntryDto.rootProcessInstanceId);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(id), hashCodeNullable(userId), hashCodeNullable(timestamp), hashCodeNullable(operationId), hashCodeNullable(operationType), hashCodeNullable(entityType), hashCodeNullable(category), hashCodeNullable(annotation), hashCodeNullable(property), hashCodeNullable(orgValue), hashCodeNullable(newValue), hashCodeNullable(deploymentId), hashCodeNullable(processDefinitionId), hashCodeNullable(processDefinitionKey), hashCodeNullable(processInstanceId), hashCodeNullable(executionId), hashCodeNullable(caseDefinitionId), hashCodeNullable(caseInstanceId), hashCodeNullable(caseExecutionId), hashCodeNullable(taskId), hashCodeNullable(externalTaskId), hashCodeNullable(batchId), hashCodeNullable(jobId), hashCodeNullable(jobDefinitionId), hashCodeNullable(removalTime), hashCodeNullable(rootProcessInstanceId));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserOperationLogEntryDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" operationId: ").append(toIndentedString(operationId)).append("\n");
sb.append(" operationType: ").append(toIndentedString(operationType)).append("\n");
sb.append(" entityType: ").append(toIndentedString(entityType)).append("\n");
sb.append(" category: ").append(toIndentedString(category)).append("\n");
sb.append(" annotation: ").append(toIndentedString(annotation)).append("\n");
sb.append(" property: ").append(toIndentedString(property)).append("\n");
sb.append(" orgValue: ").append(toIndentedString(orgValue)).append("\n");
sb.append(" newValue: ").append(toIndentedString(newValue)).append("\n");
sb.append(" deploymentId: ").append(toIndentedString(deploymentId)).append("\n");
sb.append(" processDefinitionId: ").append(toIndentedString(processDefinitionId)).append("\n");
sb.append(" processDefinitionKey: ").append(toIndentedString(processDefinitionKey)).append("\n");
sb.append(" processInstanceId: ").append(toIndentedString(processInstanceId)).append("\n");
sb.append(" executionId: ").append(toIndentedString(executionId)).append("\n");
sb.append(" caseDefinitionId: ").append(toIndentedString(caseDefinitionId)).append("\n");
sb.append(" caseInstanceId: ").append(toIndentedString(caseInstanceId)).append("\n");
sb.append(" caseExecutionId: ").append(toIndentedString(caseExecutionId)).append("\n");
sb.append(" taskId: ").append(toIndentedString(taskId)).append("\n");
sb.append(" externalTaskId: ").append(toIndentedString(externalTaskId)).append("\n");
sb.append(" batchId: ").append(toIndentedString(batchId)).append("\n");
sb.append(" jobId: ").append(toIndentedString(jobId)).append("\n");
sb.append(" jobDefinitionId: ").append(toIndentedString(jobDefinitionId)).append("\n");
sb.append(" removalTime: ").append(toIndentedString(removalTime)).append("\n");
sb.append(" rootProcessInstanceId: ").append(toIndentedString(rootProcessInstanceId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
try {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `userId` to the URL query string
if (getUserId() != null) {
try {
joiner.add(String.format("%suserId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getUserId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `timestamp` to the URL query string
if (getTimestamp() != null) {
try {
joiner.add(String.format("%stimestamp%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTimestamp()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `operationId` to the URL query string
if (getOperationId() != null) {
try {
joiner.add(String.format("%soperationId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOperationId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `operationType` to the URL query string
if (getOperationType() != null) {
try {
joiner.add(String.format("%soperationType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOperationType()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `entityType` to the URL query string
if (getEntityType() != null) {
try {
joiner.add(String.format("%sentityType%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getEntityType()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `category` to the URL query string
if (getCategory() != null) {
try {
joiner.add(String.format("%scategory%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCategory()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `annotation` to the URL query string
if (getAnnotation() != null) {
try {
joiner.add(String.format("%sannotation%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAnnotation()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `property` to the URL query string
if (getProperty() != null) {
try {
joiner.add(String.format("%sproperty%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProperty()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `orgValue` to the URL query string
if (getOrgValue() != null) {
try {
joiner.add(String.format("%sorgValue%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getOrgValue()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `newValue` to the URL query string
if (getNewValue() != null) {
try {
joiner.add(String.format("%snewValue%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getNewValue()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `deploymentId` to the URL query string
if (getDeploymentId() != null) {
try {
joiner.add(String.format("%sdeploymentId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDeploymentId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionId` to the URL query string
if (getProcessDefinitionId() != null) {
try {
joiner.add(String.format("%sprocessDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionKey` to the URL query string
if (getProcessDefinitionKey() != null) {
try {
joiner.add(String.format("%sprocessDefinitionKey%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessDefinitionKey()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processInstanceId` to the URL query string
if (getProcessInstanceId() != null) {
try {
joiner.add(String.format("%sprocessInstanceId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessInstanceId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `executionId` to the URL query string
if (getExecutionId() != null) {
try {
joiner.add(String.format("%sexecutionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getExecutionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `caseDefinitionId` to the URL query string
if (getCaseDefinitionId() != null) {
try {
joiner.add(String.format("%scaseDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCaseDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `caseInstanceId` to the URL query string
if (getCaseInstanceId() != null) {
try {
joiner.add(String.format("%scaseInstanceId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCaseInstanceId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `caseExecutionId` to the URL query string
if (getCaseExecutionId() != null) {
try {
joiner.add(String.format("%scaseExecutionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCaseExecutionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `taskId` to the URL query string
if (getTaskId() != null) {
try {
joiner.add(String.format("%staskId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTaskId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `externalTaskId` to the URL query string
if (getExternalTaskId() != null) {
try {
joiner.add(String.format("%sexternalTaskId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getExternalTaskId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `batchId` to the URL query string
if (getBatchId() != null) {
try {
joiner.add(String.format("%sbatchId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getBatchId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `jobId` to the URL query string
if (getJobId() != null) {
try {
joiner.add(String.format("%sjobId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getJobId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `jobDefinitionId` to the URL query string
if (getJobDefinitionId() != null) {
try {
joiner.add(String.format("%sjobDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getJobDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `removalTime` to the URL query string
if (getRemovalTime() != null) {
try {
joiner.add(String.format("%sremovalTime%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getRemovalTime()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `rootProcessInstanceId` to the URL query string
if (getRootProcessInstanceId() != null) {
try {
joiner.add(String.format("%srootProcessInstanceId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getRootProcessInstanceId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy