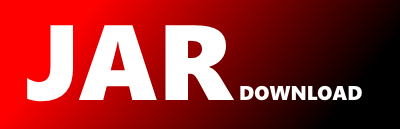
org.camunda.community.rest.client.dto.VariableInstanceQueryDto Maven / Gradle / Ivy
The newest version!
/*
* Camunda Platform REST API
* OpenApi Spec for Camunda Platform REST API.
*
* The version of the OpenAPI document: 7.21.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.camunda.community.rest.client.dto;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.camunda.community.rest.client.dto.VariableInstanceQueryDtoSortingInner;
import org.camunda.community.rest.client.dto.VariableQueryParameterDto;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* A variable instance query which defines a list of variable instances
*/
@JsonPropertyOrder({
VariableInstanceQueryDto.JSON_PROPERTY_VARIABLE_NAME,
VariableInstanceQueryDto.JSON_PROPERTY_VARIABLE_NAME_LIKE,
VariableInstanceQueryDto.JSON_PROPERTY_PROCESS_INSTANCE_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_EXECUTION_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_CASE_INSTANCE_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_CASE_EXECUTION_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_TASK_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_BATCH_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_ACTIVITY_INSTANCE_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_TENANT_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_VARIABLE_VALUES,
VariableInstanceQueryDto.JSON_PROPERTY_VARIABLE_NAMES_IGNORE_CASE,
VariableInstanceQueryDto.JSON_PROPERTY_VARIABLE_VALUES_IGNORE_CASE,
VariableInstanceQueryDto.JSON_PROPERTY_VARIABLE_SCOPE_ID_IN,
VariableInstanceQueryDto.JSON_PROPERTY_SORTING
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-27T13:50:23.655629899Z[Etc/UTC]")
public class VariableInstanceQueryDto {
public static final String JSON_PROPERTY_VARIABLE_NAME = "variableName";
private JsonNullable variableName = JsonNullable.undefined();
public static final String JSON_PROPERTY_VARIABLE_NAME_LIKE = "variableNameLike";
private JsonNullable variableNameLike = JsonNullable.undefined();
public static final String JSON_PROPERTY_PROCESS_INSTANCE_ID_IN = "processInstanceIdIn";
private JsonNullable> processInstanceIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_EXECUTION_ID_IN = "executionIdIn";
private JsonNullable> executionIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_CASE_INSTANCE_ID_IN = "caseInstanceIdIn";
private JsonNullable> caseInstanceIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_CASE_EXECUTION_ID_IN = "caseExecutionIdIn";
private JsonNullable> caseExecutionIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_TASK_ID_IN = "taskIdIn";
private JsonNullable> taskIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_BATCH_ID_IN = "batchIdIn";
private JsonNullable> batchIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_ACTIVITY_INSTANCE_ID_IN = "activityInstanceIdIn";
private JsonNullable> activityInstanceIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_TENANT_ID_IN = "tenantIdIn";
private JsonNullable> tenantIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_VARIABLE_VALUES = "variableValues";
private JsonNullable> variableValues = JsonNullable.>undefined();
public static final String JSON_PROPERTY_VARIABLE_NAMES_IGNORE_CASE = "variableNamesIgnoreCase";
private JsonNullable variableNamesIgnoreCase = JsonNullable.undefined();
public static final String JSON_PROPERTY_VARIABLE_VALUES_IGNORE_CASE = "variableValuesIgnoreCase";
private JsonNullable variableValuesIgnoreCase = JsonNullable.undefined();
public static final String JSON_PROPERTY_VARIABLE_SCOPE_ID_IN = "variableScopeIdIn";
private JsonNullable> variableScopeIdIn = JsonNullable.>undefined();
public static final String JSON_PROPERTY_SORTING = "sorting";
private JsonNullable> sorting = JsonNullable.>undefined();
public VariableInstanceQueryDto() {
}
public VariableInstanceQueryDto variableName(String variableName) {
this.variableName = JsonNullable.of(variableName);
return this;
}
/**
* Filter by variable instance name.
* @return variableName
**/
@javax.annotation.Nullable
@JsonIgnore
public String getVariableName() {
return variableName.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLE_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getVariableName_JsonNullable() {
return variableName;
}
@JsonProperty(JSON_PROPERTY_VARIABLE_NAME)
public void setVariableName_JsonNullable(JsonNullable variableName) {
this.variableName = variableName;
}
public void setVariableName(String variableName) {
this.variableName = JsonNullable.of(variableName);
}
public VariableInstanceQueryDto variableNameLike(String variableNameLike) {
this.variableNameLike = JsonNullable.of(variableNameLike);
return this;
}
/**
* Filter by the variable instance name. The parameter can include the wildcard `%` to express like-strategy such as: starts with (`%`name), ends with (name`%`) or contains (`%`name`%`).
* @return variableNameLike
**/
@javax.annotation.Nullable
@JsonIgnore
public String getVariableNameLike() {
return variableNameLike.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLE_NAME_LIKE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getVariableNameLike_JsonNullable() {
return variableNameLike;
}
@JsonProperty(JSON_PROPERTY_VARIABLE_NAME_LIKE)
public void setVariableNameLike_JsonNullable(JsonNullable variableNameLike) {
this.variableNameLike = variableNameLike;
}
public void setVariableNameLike(String variableNameLike) {
this.variableNameLike = JsonNullable.of(variableNameLike);
}
public VariableInstanceQueryDto processInstanceIdIn(List processInstanceIdIn) {
this.processInstanceIdIn = JsonNullable.>of(processInstanceIdIn);
return this;
}
public VariableInstanceQueryDto addProcessInstanceIdInItem(String processInstanceIdInItem) {
if (this.processInstanceIdIn == null || !this.processInstanceIdIn.isPresent()) {
this.processInstanceIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.processInstanceIdIn.get().add(processInstanceIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of the passed process instance ids.
* @return processInstanceIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getProcessInstanceIdIn() {
return processInstanceIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getProcessInstanceIdIn_JsonNullable() {
return processInstanceIdIn;
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_ID_IN)
public void setProcessInstanceIdIn_JsonNullable(JsonNullable> processInstanceIdIn) {
this.processInstanceIdIn = processInstanceIdIn;
}
public void setProcessInstanceIdIn(List processInstanceIdIn) {
this.processInstanceIdIn = JsonNullable.>of(processInstanceIdIn);
}
public VariableInstanceQueryDto executionIdIn(List executionIdIn) {
this.executionIdIn = JsonNullable.>of(executionIdIn);
return this;
}
public VariableInstanceQueryDto addExecutionIdInItem(String executionIdInItem) {
if (this.executionIdIn == null || !this.executionIdIn.isPresent()) {
this.executionIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.executionIdIn.get().add(executionIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of the passed execution ids.
* @return executionIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getExecutionIdIn() {
return executionIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_EXECUTION_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getExecutionIdIn_JsonNullable() {
return executionIdIn;
}
@JsonProperty(JSON_PROPERTY_EXECUTION_ID_IN)
public void setExecutionIdIn_JsonNullable(JsonNullable> executionIdIn) {
this.executionIdIn = executionIdIn;
}
public void setExecutionIdIn(List executionIdIn) {
this.executionIdIn = JsonNullable.>of(executionIdIn);
}
public VariableInstanceQueryDto caseInstanceIdIn(List caseInstanceIdIn) {
this.caseInstanceIdIn = JsonNullable.>of(caseInstanceIdIn);
return this;
}
public VariableInstanceQueryDto addCaseInstanceIdInItem(String caseInstanceIdInItem) {
if (this.caseInstanceIdIn == null || !this.caseInstanceIdIn.isPresent()) {
this.caseInstanceIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.caseInstanceIdIn.get().add(caseInstanceIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of the passed case instance ids.
* @return caseInstanceIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getCaseInstanceIdIn() {
return caseInstanceIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CASE_INSTANCE_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getCaseInstanceIdIn_JsonNullable() {
return caseInstanceIdIn;
}
@JsonProperty(JSON_PROPERTY_CASE_INSTANCE_ID_IN)
public void setCaseInstanceIdIn_JsonNullable(JsonNullable> caseInstanceIdIn) {
this.caseInstanceIdIn = caseInstanceIdIn;
}
public void setCaseInstanceIdIn(List caseInstanceIdIn) {
this.caseInstanceIdIn = JsonNullable.>of(caseInstanceIdIn);
}
public VariableInstanceQueryDto caseExecutionIdIn(List caseExecutionIdIn) {
this.caseExecutionIdIn = JsonNullable.>of(caseExecutionIdIn);
return this;
}
public VariableInstanceQueryDto addCaseExecutionIdInItem(String caseExecutionIdInItem) {
if (this.caseExecutionIdIn == null || !this.caseExecutionIdIn.isPresent()) {
this.caseExecutionIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.caseExecutionIdIn.get().add(caseExecutionIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of the passed case execution ids.
* @return caseExecutionIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getCaseExecutionIdIn() {
return caseExecutionIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_CASE_EXECUTION_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getCaseExecutionIdIn_JsonNullable() {
return caseExecutionIdIn;
}
@JsonProperty(JSON_PROPERTY_CASE_EXECUTION_ID_IN)
public void setCaseExecutionIdIn_JsonNullable(JsonNullable> caseExecutionIdIn) {
this.caseExecutionIdIn = caseExecutionIdIn;
}
public void setCaseExecutionIdIn(List caseExecutionIdIn) {
this.caseExecutionIdIn = JsonNullable.>of(caseExecutionIdIn);
}
public VariableInstanceQueryDto taskIdIn(List taskIdIn) {
this.taskIdIn = JsonNullable.>of(taskIdIn);
return this;
}
public VariableInstanceQueryDto addTaskIdInItem(String taskIdInItem) {
if (this.taskIdIn == null || !this.taskIdIn.isPresent()) {
this.taskIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.taskIdIn.get().add(taskIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of the passed task ids.
* @return taskIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getTaskIdIn() {
return taskIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TASK_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getTaskIdIn_JsonNullable() {
return taskIdIn;
}
@JsonProperty(JSON_PROPERTY_TASK_ID_IN)
public void setTaskIdIn_JsonNullable(JsonNullable> taskIdIn) {
this.taskIdIn = taskIdIn;
}
public void setTaskIdIn(List taskIdIn) {
this.taskIdIn = JsonNullable.>of(taskIdIn);
}
public VariableInstanceQueryDto batchIdIn(List batchIdIn) {
this.batchIdIn = JsonNullable.>of(batchIdIn);
return this;
}
public VariableInstanceQueryDto addBatchIdInItem(String batchIdInItem) {
if (this.batchIdIn == null || !this.batchIdIn.isPresent()) {
this.batchIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.batchIdIn.get().add(batchIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of the passed batch ids.
* @return batchIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getBatchIdIn() {
return batchIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_BATCH_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getBatchIdIn_JsonNullable() {
return batchIdIn;
}
@JsonProperty(JSON_PROPERTY_BATCH_ID_IN)
public void setBatchIdIn_JsonNullable(JsonNullable> batchIdIn) {
this.batchIdIn = batchIdIn;
}
public void setBatchIdIn(List batchIdIn) {
this.batchIdIn = JsonNullable.>of(batchIdIn);
}
public VariableInstanceQueryDto activityInstanceIdIn(List activityInstanceIdIn) {
this.activityInstanceIdIn = JsonNullable.>of(activityInstanceIdIn);
return this;
}
public VariableInstanceQueryDto addActivityInstanceIdInItem(String activityInstanceIdInItem) {
if (this.activityInstanceIdIn == null || !this.activityInstanceIdIn.isPresent()) {
this.activityInstanceIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.activityInstanceIdIn.get().add(activityInstanceIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of the passed activity instance ids.
* @return activityInstanceIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getActivityInstanceIdIn() {
return activityInstanceIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_INSTANCE_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getActivityInstanceIdIn_JsonNullable() {
return activityInstanceIdIn;
}
@JsonProperty(JSON_PROPERTY_ACTIVITY_INSTANCE_ID_IN)
public void setActivityInstanceIdIn_JsonNullable(JsonNullable> activityInstanceIdIn) {
this.activityInstanceIdIn = activityInstanceIdIn;
}
public void setActivityInstanceIdIn(List activityInstanceIdIn) {
this.activityInstanceIdIn = JsonNullable.>of(activityInstanceIdIn);
}
public VariableInstanceQueryDto tenantIdIn(List tenantIdIn) {
this.tenantIdIn = JsonNullable.>of(tenantIdIn);
return this;
}
public VariableInstanceQueryDto addTenantIdInItem(String tenantIdInItem) {
if (this.tenantIdIn == null || !this.tenantIdIn.isPresent()) {
this.tenantIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.tenantIdIn.get().add(tenantIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of the passed tenant ids.
* @return tenantIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getTenantIdIn() {
return tenantIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_TENANT_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getTenantIdIn_JsonNullable() {
return tenantIdIn;
}
@JsonProperty(JSON_PROPERTY_TENANT_ID_IN)
public void setTenantIdIn_JsonNullable(JsonNullable> tenantIdIn) {
this.tenantIdIn = tenantIdIn;
}
public void setTenantIdIn(List tenantIdIn) {
this.tenantIdIn = JsonNullable.>of(tenantIdIn);
}
public VariableInstanceQueryDto variableValues(List variableValues) {
this.variableValues = JsonNullable.>of(variableValues);
return this;
}
public VariableInstanceQueryDto addVariableValuesItem(VariableQueryParameterDto variableValuesItem) {
if (this.variableValues == null || !this.variableValues.isPresent()) {
this.variableValues = JsonNullable.>of(new ArrayList<>());
}
try {
this.variableValues.get().add(variableValuesItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* An array to only include variable instances that have the certain values. The array consists of objects with the three properties `name`, `operator` and `value`. `name (String)` is the variable name, `operator (String)` is the comparison operator to be used and `value` the variable value. `value` may be `String`, `Number` or `Boolean`. Valid operator values are: `eq` - equal to; `neq` - not equal to; `gt` - greater than; `gteq` - greater than or equal to; `lt` - lower than; `lteq` - lower than or equal to; `like`
* @return variableValues
**/
@javax.annotation.Nullable
@JsonIgnore
public List getVariableValues() {
return variableValues.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLE_VALUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getVariableValues_JsonNullable() {
return variableValues;
}
@JsonProperty(JSON_PROPERTY_VARIABLE_VALUES)
public void setVariableValues_JsonNullable(JsonNullable> variableValues) {
this.variableValues = variableValues;
}
public void setVariableValues(List variableValues) {
this.variableValues = JsonNullable.>of(variableValues);
}
public VariableInstanceQueryDto variableNamesIgnoreCase(Boolean variableNamesIgnoreCase) {
this.variableNamesIgnoreCase = JsonNullable.of(variableNamesIgnoreCase);
return this;
}
/**
* Match all variable names provided in `variableValues` case-insensitively. If set to `true` **variableName** and **variablename** are treated as equal.
* @return variableNamesIgnoreCase
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getVariableNamesIgnoreCase() {
return variableNamesIgnoreCase.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLE_NAMES_IGNORE_CASE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getVariableNamesIgnoreCase_JsonNullable() {
return variableNamesIgnoreCase;
}
@JsonProperty(JSON_PROPERTY_VARIABLE_NAMES_IGNORE_CASE)
public void setVariableNamesIgnoreCase_JsonNullable(JsonNullable variableNamesIgnoreCase) {
this.variableNamesIgnoreCase = variableNamesIgnoreCase;
}
public void setVariableNamesIgnoreCase(Boolean variableNamesIgnoreCase) {
this.variableNamesIgnoreCase = JsonNullable.of(variableNamesIgnoreCase);
}
public VariableInstanceQueryDto variableValuesIgnoreCase(Boolean variableValuesIgnoreCase) {
this.variableValuesIgnoreCase = JsonNullable.of(variableValuesIgnoreCase);
return this;
}
/**
* Match all variable values provided in `variableValues` case-insensitively. If set to `true` **variableValue** and **variablevalue** are treated as equal.
* @return variableValuesIgnoreCase
**/
@javax.annotation.Nullable
@JsonIgnore
public Boolean getVariableValuesIgnoreCase() {
return variableValuesIgnoreCase.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLE_VALUES_IGNORE_CASE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getVariableValuesIgnoreCase_JsonNullable() {
return variableValuesIgnoreCase;
}
@JsonProperty(JSON_PROPERTY_VARIABLE_VALUES_IGNORE_CASE)
public void setVariableValuesIgnoreCase_JsonNullable(JsonNullable variableValuesIgnoreCase) {
this.variableValuesIgnoreCase = variableValuesIgnoreCase;
}
public void setVariableValuesIgnoreCase(Boolean variableValuesIgnoreCase) {
this.variableValuesIgnoreCase = JsonNullable.of(variableValuesIgnoreCase);
}
public VariableInstanceQueryDto variableScopeIdIn(List variableScopeIdIn) {
this.variableScopeIdIn = JsonNullable.>of(variableScopeIdIn);
return this;
}
public VariableInstanceQueryDto addVariableScopeIdInItem(String variableScopeIdInItem) {
if (this.variableScopeIdIn == null || !this.variableScopeIdIn.isPresent()) {
this.variableScopeIdIn = JsonNullable.>of(new ArrayList<>());
}
try {
this.variableScopeIdIn.get().add(variableScopeIdInItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* Only include variable instances which belong to one of passed scope ids.
* @return variableScopeIdIn
**/
@javax.annotation.Nullable
@JsonIgnore
public List getVariableScopeIdIn() {
return variableScopeIdIn.orElse(null);
}
@JsonProperty(JSON_PROPERTY_VARIABLE_SCOPE_ID_IN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getVariableScopeIdIn_JsonNullable() {
return variableScopeIdIn;
}
@JsonProperty(JSON_PROPERTY_VARIABLE_SCOPE_ID_IN)
public void setVariableScopeIdIn_JsonNullable(JsonNullable> variableScopeIdIn) {
this.variableScopeIdIn = variableScopeIdIn;
}
public void setVariableScopeIdIn(List variableScopeIdIn) {
this.variableScopeIdIn = JsonNullable.>of(variableScopeIdIn);
}
public VariableInstanceQueryDto sorting(List sorting) {
this.sorting = JsonNullable.>of(sorting);
return this;
}
public VariableInstanceQueryDto addSortingItem(VariableInstanceQueryDtoSortingInner sortingItem) {
if (this.sorting == null || !this.sorting.isPresent()) {
this.sorting = JsonNullable.>of(new ArrayList<>());
}
try {
this.sorting.get().add(sortingItem);
} catch (java.util.NoSuchElementException e) {
// this can never happen, as we make sure above that the value is present
}
return this;
}
/**
* An array of criteria to sort the result by. Each element of the array is an object that specifies one ordering. The position in the array identifies the rank of an ordering, i.e., whether it is primary, secondary, etc. Sorting has no effect for `count` endpoints
* @return sorting
**/
@javax.annotation.Nullable
@JsonIgnore
public List getSorting() {
return sorting.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SORTING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable> getSorting_JsonNullable() {
return sorting;
}
@JsonProperty(JSON_PROPERTY_SORTING)
public void setSorting_JsonNullable(JsonNullable> sorting) {
this.sorting = sorting;
}
public void setSorting(List sorting) {
this.sorting = JsonNullable.>of(sorting);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
VariableInstanceQueryDto variableInstanceQueryDto = (VariableInstanceQueryDto) o;
return equalsNullable(this.variableName, variableInstanceQueryDto.variableName) &&
equalsNullable(this.variableNameLike, variableInstanceQueryDto.variableNameLike) &&
equalsNullable(this.processInstanceIdIn, variableInstanceQueryDto.processInstanceIdIn) &&
equalsNullable(this.executionIdIn, variableInstanceQueryDto.executionIdIn) &&
equalsNullable(this.caseInstanceIdIn, variableInstanceQueryDto.caseInstanceIdIn) &&
equalsNullable(this.caseExecutionIdIn, variableInstanceQueryDto.caseExecutionIdIn) &&
equalsNullable(this.taskIdIn, variableInstanceQueryDto.taskIdIn) &&
equalsNullable(this.batchIdIn, variableInstanceQueryDto.batchIdIn) &&
equalsNullable(this.activityInstanceIdIn, variableInstanceQueryDto.activityInstanceIdIn) &&
equalsNullable(this.tenantIdIn, variableInstanceQueryDto.tenantIdIn) &&
equalsNullable(this.variableValues, variableInstanceQueryDto.variableValues) &&
equalsNullable(this.variableNamesIgnoreCase, variableInstanceQueryDto.variableNamesIgnoreCase) &&
equalsNullable(this.variableValuesIgnoreCase, variableInstanceQueryDto.variableValuesIgnoreCase) &&
equalsNullable(this.variableScopeIdIn, variableInstanceQueryDto.variableScopeIdIn) &&
equalsNullable(this.sorting, variableInstanceQueryDto.sorting);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(variableName), hashCodeNullable(variableNameLike), hashCodeNullable(processInstanceIdIn), hashCodeNullable(executionIdIn), hashCodeNullable(caseInstanceIdIn), hashCodeNullable(caseExecutionIdIn), hashCodeNullable(taskIdIn), hashCodeNullable(batchIdIn), hashCodeNullable(activityInstanceIdIn), hashCodeNullable(tenantIdIn), hashCodeNullable(variableValues), hashCodeNullable(variableNamesIgnoreCase), hashCodeNullable(variableValuesIgnoreCase), hashCodeNullable(variableScopeIdIn), hashCodeNullable(sorting));
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class VariableInstanceQueryDto {\n");
sb.append(" variableName: ").append(toIndentedString(variableName)).append("\n");
sb.append(" variableNameLike: ").append(toIndentedString(variableNameLike)).append("\n");
sb.append(" processInstanceIdIn: ").append(toIndentedString(processInstanceIdIn)).append("\n");
sb.append(" executionIdIn: ").append(toIndentedString(executionIdIn)).append("\n");
sb.append(" caseInstanceIdIn: ").append(toIndentedString(caseInstanceIdIn)).append("\n");
sb.append(" caseExecutionIdIn: ").append(toIndentedString(caseExecutionIdIn)).append("\n");
sb.append(" taskIdIn: ").append(toIndentedString(taskIdIn)).append("\n");
sb.append(" batchIdIn: ").append(toIndentedString(batchIdIn)).append("\n");
sb.append(" activityInstanceIdIn: ").append(toIndentedString(activityInstanceIdIn)).append("\n");
sb.append(" tenantIdIn: ").append(toIndentedString(tenantIdIn)).append("\n");
sb.append(" variableValues: ").append(toIndentedString(variableValues)).append("\n");
sb.append(" variableNamesIgnoreCase: ").append(toIndentedString(variableNamesIgnoreCase)).append("\n");
sb.append(" variableValuesIgnoreCase: ").append(toIndentedString(variableValuesIgnoreCase)).append("\n");
sb.append(" variableScopeIdIn: ").append(toIndentedString(variableScopeIdIn)).append("\n");
sb.append(" sorting: ").append(toIndentedString(sorting)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `variableName` to the URL query string
if (getVariableName() != null) {
try {
joiner.add(String.format("%svariableName%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getVariableName()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `variableNameLike` to the URL query string
if (getVariableNameLike() != null) {
try {
joiner.add(String.format("%svariableNameLike%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getVariableNameLike()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processInstanceIdIn` to the URL query string
if (getProcessInstanceIdIn() != null) {
for (int i = 0; i < getProcessInstanceIdIn().size(); i++) {
try {
joiner.add(String.format("%sprocessInstanceIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getProcessInstanceIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `executionIdIn` to the URL query string
if (getExecutionIdIn() != null) {
for (int i = 0; i < getExecutionIdIn().size(); i++) {
try {
joiner.add(String.format("%sexecutionIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getExecutionIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `caseInstanceIdIn` to the URL query string
if (getCaseInstanceIdIn() != null) {
for (int i = 0; i < getCaseInstanceIdIn().size(); i++) {
try {
joiner.add(String.format("%scaseInstanceIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getCaseInstanceIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `caseExecutionIdIn` to the URL query string
if (getCaseExecutionIdIn() != null) {
for (int i = 0; i < getCaseExecutionIdIn().size(); i++) {
try {
joiner.add(String.format("%scaseExecutionIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getCaseExecutionIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `taskIdIn` to the URL query string
if (getTaskIdIn() != null) {
for (int i = 0; i < getTaskIdIn().size(); i++) {
try {
joiner.add(String.format("%staskIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getTaskIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `batchIdIn` to the URL query string
if (getBatchIdIn() != null) {
for (int i = 0; i < getBatchIdIn().size(); i++) {
try {
joiner.add(String.format("%sbatchIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getBatchIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `activityInstanceIdIn` to the URL query string
if (getActivityInstanceIdIn() != null) {
for (int i = 0; i < getActivityInstanceIdIn().size(); i++) {
try {
joiner.add(String.format("%sactivityInstanceIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getActivityInstanceIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `tenantIdIn` to the URL query string
if (getTenantIdIn() != null) {
for (int i = 0; i < getTenantIdIn().size(); i++) {
try {
joiner.add(String.format("%stenantIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getTenantIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `variableValues` to the URL query string
if (getVariableValues() != null) {
for (int i = 0; i < getVariableValues().size(); i++) {
if (getVariableValues().get(i) != null) {
joiner.add(getVariableValues().get(i).toUrlQueryString(String.format("%svariableValues%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
// add `variableNamesIgnoreCase` to the URL query string
if (getVariableNamesIgnoreCase() != null) {
try {
joiner.add(String.format("%svariableNamesIgnoreCase%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getVariableNamesIgnoreCase()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `variableValuesIgnoreCase` to the URL query string
if (getVariableValuesIgnoreCase() != null) {
try {
joiner.add(String.format("%svariableValuesIgnoreCase%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getVariableValuesIgnoreCase()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `variableScopeIdIn` to the URL query string
if (getVariableScopeIdIn() != null) {
for (int i = 0; i < getVariableScopeIdIn().size(); i++) {
try {
joiner.add(String.format("%svariableScopeIdIn%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getVariableScopeIdIn().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `sorting` to the URL query string
if (getSorting() != null) {
for (int i = 0; i < getSorting().size(); i++) {
if (getSorting().get(i) != null) {
joiner.add(getSorting().get(i).toUrlQueryString(String.format("%ssorting%s%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix))));
}
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy