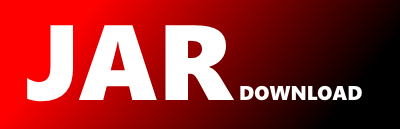
org.camunda.spin.json.SpinJsonNode Maven / Gradle / Ivy
/* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.camunda.spin.json;
import org.camunda.spin.Spin;
import org.camunda.spin.SpinList;
import org.camunda.spin.spi.SpinDataFormatException;
import java.util.List;
import java.util.Map;
/**
* A json node.
*
* @author Thorben Lindhauer
* @author Stefan Hentschel
*/
public abstract class SpinJsonNode extends Spin {
/**
* Fetches the first index of the searched object in an array.
*
* @param searchObject Object for which the index should be searched.
* @return {@link Integer} index of searchObject.
* @throws SpinJsonException if the current node is not an array.
* @throws SpinJsonPropertyException if object is not found.
*/
public abstract Integer indexOf(Object searchObject);
/**
* Fetches the last index of the searched object in an array.
*
* @param searchObject Object for which the index should be searched.
* @return {@link Integer} index of searchObject or -1 if object not found.
* @throws SpinJsonException if the current node is not an array.
*/
public abstract Integer lastIndexOf(Object searchObject);
/**
* Check if this node is an object node.
*
* @return true if the node is an object, false otherwise
*/
public abstract boolean isObject();
/**
* Check if this node has a property with the given name.
*
* @param name the name of the property
* @return true if this node has a property with this name, false otherwise
*/
public abstract boolean hasProp(String name);
/**
* Get the property of this node with the given name.
*
* @param name the name of the property
* @return {@link SpinJsonNode} representation of the property
*/
public abstract SpinJsonNode prop(String name);
/**
* Set a new String property in this node.
*
* @param name the name of the new property
* @param newProperty the new String property
* @return {@link SpinJsonNode} representation of the current node
*/
public abstract SpinJsonNode prop(String name, String newProperty);
/**
* Set a new Number property in this node.
*
* @param name the name of the new property
* @param newProperty the new Number property
* @return {@link SpinJsonNode} representation of the current node
*/
public abstract SpinJsonNode prop(String name, Number newProperty);
/**
* Set a new int property in this node.
*
* @param name the name of the new property
* @param newProperty the new int property
* @return {@link SpinJsonNode} representation of the current node
*/
public abstract SpinJsonNode prop(String name, int newProperty);
/**
* Set a new float property in this node.
*
* @param name the name of the new property
* @param newProperty the new float property
* @return {@link SpinJsonNode} representation of the current node
*/
public abstract SpinJsonNode prop(String name, float newProperty);
/**
* Set a new long property in this node.
*
* @param name the name of the new property
* @param newProperty the new long property
* @return {@link SpinJsonNode} representation of the current node
*/
public abstract SpinJsonNode prop(String name, long newProperty);
/**
* Set a new boolean property in this node.
*
* @param name the name of the new property
* @param newProperty the new boolean property
* @return {@link SpinJsonNode} representation of the current node
*/
public abstract SpinJsonNode prop(String name, boolean newProperty);
/**
* Set a new Boolean property in this node.
*
* @param name the name of the new property
* @param newProperty the new Boolean property
* @return {@link SpinJsonNode} representation of the current node
*/
public abstract SpinJsonNode prop(String name, Boolean newProperty);
/**
* Set a new List property in this node.
*
* @param name the name of the new property
* @param newProperty the new List property
* @return {@link SpinJsonNode} representation of the current node
*/
public abstract SpinJsonNode prop(String name, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy