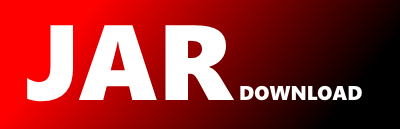
org.canedata.provider.mongodb.MongoResource Maven / Gradle / Ivy
/**
* Copyright 2011 CaneData.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.canedata.provider.mongodb;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.canedata.core.logging.LoggerFactory;
import org.canedata.core.util.StringUtils;
import org.canedata.logging.Logger;
import org.canedata.resource.Resource;
import org.canedata.resource.meta.EntityMeta;
import org.canedata.resource.meta.RepositoryMeta;
import com.mongodb.DB;
import com.mongodb.Mongo;
/**
*
* @author Sun Yat-ton
* @version 1.00.000 2011-8-1
*/
public abstract class MongoResource implements Resource {
final static Logger logger = LoggerFactory.getLogger(MongoResource.class);
private static String NAME = "Resource for mongo-java-driver";
private static String VERSION = "2.6.3";
abstract Mongo getMongo();
abstract MongoResourceProvider getProvider();
public boolean isWrappedFor(Class> iface) {
return iface.isInstance(this);
}
public T unwrap(Class iface) {
return iface.cast(getMongo());
}
public String getName() {
return NAME;
}
public String getVersion() {
return VERSION;
}
public List getRepositories() {
List dbs = getMongo().getDatabaseNames();
List reps = new ArrayList(dbs.size());
for (final String name : dbs) {
reps.add(new RepositoryMeta() {
String label = name;
public String getLabel() {
return label;
}
public Object getAttribute(String name) {
// TODO Auto-generated method stub
return null;
}
public Map getAttributes() {
// TODO Auto-generated method stub
return null;
}
public int sequence() {
// TODO Auto-generated method stub
return 0;
}
public String describe() {
// TODO Auto-generated method stub
return null;
}
public List getEntities() {
// TODO Auto-generated method stub
return null;
}
public EntityMeta getEntity(String name) {
// TODO Auto-generated method stub
return null;
}
public String getName() {
return name;
}
});
}
return reps;
}
public DB take() {
String dbname = getProvider().getDefaultDBName();
if (StringUtils.isBlank(dbname)) {
dbname = getMongo().getDatabaseNames().get(0);
}
return take(dbname);
}
public DB take(Object... args) {
if (args == null || args.length == 0)
throw new IllegalArgumentException(
"Must specify the database name.");
String dbname = (String) args[0];
if(logger.isDebug())
logger.debug("Taking the resource {0}.", dbname);
DB db = getMongo().getDB(dbname);
if (getProvider().getAuthentications().containsKey(dbname)) {
String[] auth = getProvider().getAuthentications().get(dbname);
if (!db.authenticate(auth[0], auth[1].toCharArray())) {
throw new RuntimeException("Authenticate failure!");
}
}
db.requestStart();
db.requestEnsureConnection();
return db;
}
public DB take(boolean exclusive) {
return take();
}
public DB take(boolean exclusive, Object... args) {
return take(args);
}
public DB checkout() {
return take(true);
}
public DB checkout(Object... args) {
return take(true, args);
}
public Resource release(Object target) {
if (!(target instanceof DB))
throw new IllegalArgumentException(
"Specifies the instance of the target is not "
+ DB.class.getName());
if(logger.isDebug())
logger.debug("Release the resource {0}.", ((DB) target).getName());
((DB) target).requestDone();
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy