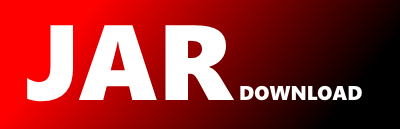
org.carrot2.attrs.AttrObjectArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of carrot2-core Show documentation
Show all versions of carrot2-core Show documentation
Carrot2 Text Clustering Library
/*
* Carrot2 project.
*
* Copyright (C) 2002-2021, Dawid Weiss, Stanisław Osiński.
* All rights reserved.
*
* Refer to the full license file "carrot2.LICENSE"
* in the root folder of the repository checkout or at:
* https://www.carrot2.org/carrot2.LICENSE
*/
package org.carrot2.attrs;
import java.util.List;
import java.util.Objects;
import java.util.function.Consumer;
import java.util.function.Supplier;
public class AttrObjectArray extends Attr> {
private Class clazz;
private Supplier> getter;
private Consumer> setter;
private Supplier extends T> newEntryInstance;
AttrObjectArray(
Class clazz,
List defaultValue,
String label,
List extends Constraint super List>> constraint,
Supplier extends T> newEntryInstance,
Supplier> getter,
Consumer> setter) {
super(null, label, constraint);
this.clazz = clazz;
this.setter = setter != null ? setter : super::set;
this.getter = getter != null ? getter : super::get;
this.newEntryInstance = newEntryInstance;
set(defaultValue);
}
public void set(List value) {
super.set(value);
setter.accept(value);
}
public List get() {
return getter.get();
}
public Class getInterfaceClass() {
return clazz;
}
public boolean isDefaultClass(Object value) {
Objects.requireNonNull(value);
T def = newDefaultEntryValue();
return def != null && Objects.equals(def.getClass(), value.getClass());
}
public T newDefaultEntryValue() {
return this.newEntryInstance.get();
}
public static class Builder extends BuilderScaffold> {
private final Supplier extends T> defaultEntryValue;
private Class clazz;
private Supplier> getter;
private Consumer> setter;
public Builder(Class clazz, Supplier extends T> defaultEntryValue) {
this.clazz = clazz;
this.defaultEntryValue = defaultEntryValue;
}
public Builder getset(Supplier> getter, Consumer> setter) {
this.setter = Objects.requireNonNull(setter);
this.getter = Objects.requireNonNull(getter);
return this;
}
@Override
public Builder label(String label) {
super.label(label);
return this;
}
public AttrObjectArray defaultValue(List defaultValue) {
return new AttrObjectArray(
clazz, defaultValue, label, getConstraint(), defaultEntryValue, getter, setter);
}
}
public static Builder builder(
Class entryClazz, Supplier extends T> defaultEntryValue) {
return new Builder<>(entryClazz, defaultEntryValue);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy