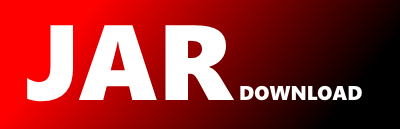
org.carrot2.core.ProcessingComponentSuite Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of carrot2-mini Show documentation
Show all versions of carrot2-mini Show documentation
Carrot2 search results clustering framework. Minimal functional subset
(core algorithms and infrastructure, no document sources).
/*
* Carrot2 project.
*
* Copyright (C) 2002-2016, Dawid Weiss, Stanisław Osiński.
* All rights reserved.
*
* Refer to the full license file "carrot2.LICENSE"
* in the root folder of the repository checkout or at:
* http://www.carrot2.org/carrot2.LICENSE
*/
package org.carrot2.core;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.carrot2.util.CloseableUtils;
import org.carrot2.util.resource.IResource;
import org.carrot2.util.resource.ResourceLookup;
import org.carrot2.util.simplexml.PersisterHelpers;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import org.simpleframework.xml.core.Commit;
import org.simpleframework.xml.core.Persister;
import org.simpleframework.xml.strategy.TreeStrategy;
import org.carrot2.shaded.guava.common.collect.Iterables;
import org.carrot2.shaded.guava.common.collect.Iterators;
import org.carrot2.shaded.guava.common.collect.Lists;
/**
* A set of {@link IProcessingComponent}s used in Carrot2 applications.
*/
@Root(name = "component-suite")
public class ProcessingComponentSuite
{
@ElementList(inline = true, required = false, entry = "include")
ArrayList includes;
@ElementList(name = "sources", entry = "source", required = false)
private ArrayList sources;
@ElementList(name = "algorithms", entry = "algorithm", required = false)
private ArrayList algorithms;
@ElementList(name = "components", entry = "component", required = false)
private ArrayList otherComponents;
public ProcessingComponentSuite()
{
}
public ProcessingComponentSuite(ArrayList sources,
ArrayList algorithms)
{
this.algorithms = algorithms;
this.sources = sources;
this.otherComponents = Lists.newArrayList();
}
/**
* Returns the internal list of document sources. Changes to this list will affect the
* suite.
*/
public List getSources()
{
return sources;
}
/**
* Returns the internal list of algorithms. Changes to this list will affect the
* suite.
*/
public List getAlgorithms()
{
return algorithms;
}
/**
* Return a list of other components (not algorithms, not sources).
*/
public List getOtherComponents()
{
return otherComponents;
}
/**
* Returns all components available in this suite, including data sources, algorithms
* and any other types.
*/
public List getComponents()
{
return Lists.newArrayList(Iterables.concat(sources, algorithms, otherComponents));
}
/**
* Replace missing attributes with empty lists.
*/
@Commit
private void postDeserialize(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy