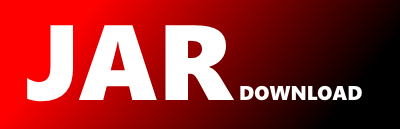
org.catools.common.extensions.verify.CMapVerification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
The common objects which use in all other CATools projects
package org.catools.common.extensions.verify;
import org.catools.common.extensions.verify.interfaces.CMapVerifier;
import org.catools.common.logger.CLogger;
import java.util.Map;
/**
* Map verification class contains all verification method which is related to Map
*
* @param represent any classes which extent {@link CVerificationBuilder}.
*/
public class CMapVerification extends CBaseVerification {
public CMapVerification(T verifier, CLogger logger) {
super(verifier, logger);
}
/**
* Verify that actual map contains the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void contains(Map actual, Map.Entry expected, final String message, final Object... params) {
toVerifier(actual).verifyContains(verifier, expected, message, params);
}
/**
* Verify that actual map contains the expected key and value.
*
* @param actual map to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void contains(Map actual, K expectedKey, V expectedValue, final String message, final Object... params) {
toVerifier(actual).verifyContains(verifier, expectedKey, expectedValue, message, params);
}
/**
* Verify that actual map contains the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void contains(Map actual, Map.Entry expected, final int waitInSeconds, final String message, final Object... params) {
toVerifier(actual).verifyContains(verifier, expected, waitInSeconds, message, params);
}
/**
* Verify that actual map contains the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void contains(Map actual,
Map.Entry expected,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyContains(verifier, expected, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map contains the expected key and value.
*
* @param actual map to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void contains(Map actual, K expectedKey, V expectedValue, final int waitInSeconds,
final String message, final Object... params) {
toVerifier(actual).verifyContains(verifier, expectedKey, expectedValue, waitInSeconds, message, params);
}
/**
* Verify that actual map contains the expected key and value.
*
* @param actual map to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void contains(Map actual,
K expectedKey,
V expectedValue,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyContains(verifier, expectedKey, expectedValue, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map contains all entries from the expected map.
* Please note that actual map might have more entries.
*
* @param actual value to compare
* @param expected value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void containsAll(Map actual, Map expected, final String message, final Object... params) {
toVerifier(actual).verifyContainsAll(verifier, expected, message, params);
}
/**
* Verify that actual map contains all entries from the expected map.
* Please note that actual map might have more entries.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void containsAll(Map actual, Map expected, final int waitInSeconds, final String message, final Object... params) {
toVerifier(actual).verifyContainsAll(verifier, expected, waitInSeconds, message, params);
}
/**
* Verify that actual map contains all entries from the expected map.
* Please note that actual map might have more entries.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void containsAll(Map actual, Map expected, final int waitInSeconds, final int intervalInMilliSeconds, final String message, final Object... params) {
toVerifier(actual).verifyContainsAll(verifier, expected, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map contains none of entries from the expected map.
*
* @param actual value to compare
* @param expected value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void containsNone(Map actual, Map expected, final String message, final Object... params) {
toVerifier(actual).verifyContainsNone(verifier, expected, message, params);
}
/**
* Verify that actual map contains none of entries from the expected map.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void containsNone(Map actual, Map expected, final int waitInSeconds, final String message, final Object... params) {
toVerifier(actual).verifyContainsNone(verifier, expected, waitInSeconds, message, params);
}
/**
* Verify that actual map contains none of entries from the expected map.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void containsNone(Map actual, Map expected, final int waitInSeconds, final int intervalInMilliSeconds, final String message, final Object... params) {
toVerifier(actual).verifyContainsNone(verifier, expected, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map either is empty or contains the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrContains(Map actual, K expectedKey, V expectedValue, final String message, final Object... params) {
toVerifier(actual).verifyEmptyOrContains(verifier, expectedKey, expectedValue, message, params);
}
/**
* Verify that actual map either is empty or contains the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrContains(Map actual, Map.Entry expected, final String message, final Object... params) {
toVerifier(actual).verifyEmptyOrContains(verifier, expected, message, params);
}
/**
* Verify that actual map either is empty or contains the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrContains(Map actual, K expectedKey, V expectedValue, final int waitInSeconds,
final String message, final Object... params) {
toVerifier(actual).verifyEmptyOrContains(verifier, expectedKey, expectedValue, waitInSeconds, message, params);
}
/**
* Verify that actual map either is empty or contains the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrContains(Map actual,
K expectedKey,
V expectedValue,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyEmptyOrContains(verifier, expectedKey, expectedValue, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map either is empty or contains the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrContains(Map actual, Map.Entry expected, final int waitInSeconds,
final String message, final Object... params) {
toVerifier(actual).verifyEmptyOrContains(verifier, expected, waitInSeconds, message, params);
}
/**
* Verify that actual map either is empty or contains the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrContains(Map actual,
Map.Entry expected,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyEmptyOrContains(verifier, expected, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map either is empty or does not contains the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrNotContains(Map actual, K expectedKey, V expectedValue, final String message, final Object... params) {
toVerifier(actual).verifyEmptyOrNotContains(verifier, expectedKey, expectedValue, message, params);
}
/**
* Verify that actual map either is empty or does not contain the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrNotContains(Map actual, Map.Entry expected, final String message, final Object... params) {
toVerifier(actual).verifyEmptyOrNotContains(verifier, expected, message, params);
}
/**
* Verify that actual map either is empty or does not contains the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrNotContains(Map actual, K expectedKey, V expectedValue, final int waitInSeconds,
final String message, final Object... params) {
toVerifier(actual).verifyEmptyOrNotContains(verifier, expectedKey, expectedValue, waitInSeconds, message, params);
}
/**
* Verify that actual map either is empty or does not contains the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrNotContains(Map actual,
K expectedKey,
V expectedValue,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyEmptyOrNotContains(verifier, expectedKey, expectedValue, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map either is empty or does not contain the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrNotContains(Map actual, Map.Entry expected, final int waitInSeconds,
final String message, final Object... params) {
toVerifier(actual).verifyEmptyOrNotContains(verifier, expected, waitInSeconds, message, params);
}
/**
* Verify that actual map either is empty or does not contain the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void emptyOrNotContains(Map actual,
Map.Entry expected,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyEmptyOrNotContains(verifier, expected, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual and expected maps have the exact same entries. (Ignore entry order)
* First we compare that actual map contains all expected map entries and then we verify that expected has all entries from actual.
*
* @param actual map to compare
* @param expected map to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void equals(Map actual, Map expected, final String message, final Object... params) {
toVerifier(actual).verifyEquals(verifier, expected, message, params);
}
/**
* Verify that actual and expected maps have the exact same entries. (Ignore entry order)
* First we compare that actual map contains all expected map entries and then we verify that expected has all entries from actual.
*
* @param actual map to compare
* @param expected map to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void equals(Map actual, Map expected, final int waitInSeconds, final String message, final Object... params) {
toVerifier(actual).verifyEquals(verifier, expected, waitInSeconds, message, params);
}
/**
* Verify that actual and expected maps have the exact same entries. (Ignore entry order)
* First we compare that actual map contains all expected map entries and then we verify that expected has all entries from actual.
*
* @param actual map to compare
* @param expected map to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void equals(Map actual, Map expected, final int waitInSeconds, final int intervalInMilliSeconds, final String message, final Object... params) {
toVerifier(actual).verifyEquals(verifier, expected, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map is empty.
*
* @param actual value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void isEmpty(Map actual, final String message, final Object... params) {
toVerifier(actual).verifyIsEmpty(verifier, message, params);
}
/**
* Verify that actual map is empty.
*
* @param actual value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void isEmpty(Map actual, final int waitInSeconds, final String message, final Object... params) {
toVerifier(actual).verifyIsEmpty(verifier, waitInSeconds, message, params);
}
/**
* Verify that actual map is empty.
*
* @param actual value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void isEmpty(Map actual, final int waitInSeconds, final int intervalInMilliSeconds, final String message, final Object... params) {
toVerifier(actual).verifyIsEmpty(verifier, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map is not empty. (might contains null values)
*
* @param actual value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void isNotEmpty(Map actual, final String message, final Object... params) {
toVerifier(actual).verifyIsNotEmpty(verifier, message, params);
}
/**
* Verify that actual map is not empty. (might contains null values)
*
* @param actual value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void isNotEmpty(Map actual, final int waitInSeconds, final String message, final Object... params) {
toVerifier(actual).verifyIsNotEmpty(verifier, waitInSeconds, message, params);
}
/**
* Verify that actual map is not empty. (might contains null values)
*
* @param actual value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void isNotEmpty(Map actual, final int waitInSeconds, final int intervalInMilliSeconds, final String message, final Object... params) {
toVerifier(actual).verifyIsNotEmpty(verifier, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map does not contain the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContains(Map actual, Map.Entry expected, final String message, final Object... params) {
toVerifier(actual).verifyNotContains(verifier, expected, message, params);
}
/**
* Verify that actual map does not contain the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContains(Map actual, K expectedKey, V expectedValue, final String message, final Object... params) {
toVerifier(actual).verifyNotContains(verifier, expectedKey, expectedValue, message, params);
}
/**
* Verify that actual map does not contain the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContains(Map actual, Map.Entry expected, final int waitInSeconds,
final String message, final Object... params) {
toVerifier(actual).verifyNotContains(verifier, expected, waitInSeconds, message, params);
}
/**
* Verify that actual map does not contain the expected entry.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContains(Map actual,
Map.Entry expected,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyNotContains(verifier, expected, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map does not contain the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContains(Map actual, K expectedKey, V expectedValue, final int waitInSeconds,
final String message, final Object... params) {
toVerifier(actual).verifyNotContains(verifier, expectedKey, expectedValue, waitInSeconds, message, params);
}
/**
* Verify that actual map does not contain the expected entry.
*
* @param actual value to compare
* @param expectedKey key to compare
* @param expectedValue value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContains(Map actual,
K expectedKey,
V expectedValue,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyNotContains(verifier, expectedKey, expectedValue, waitInSeconds, intervalInMilliSeconds, message, params);
}
/**
* Verify that actual map does not contain all entries from the expected map.
* Please note that actual map might have some of entries but the point is to ensure that not all expected entries are exist in it.
*
* @param actual value to compare
* @param expected value to compare
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContainsAll(Map actual, Map expected, final String message, final Object... params) {
toVerifier(actual).verifyNotContainsAll(verifier, expected, message, params);
}
/**
* Verify that actual map does not contain all entries from the expected map.
* Please note that actual map might have some of entries but the point is to ensure that not all expected entries are exist in it.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContainsAll(Map actual, Map expected, final int waitInSeconds, final String message, final Object... params) {
toVerifier(actual).verifyNotContainsAll(verifier, expected, waitInSeconds, message, params);
}
/**
* Verify that actual map does not contain all entries from the expected map.
* Please note that actual map might have some of entries but the point is to ensure that not all expected entries are exist in it.
*
* @param actual value to compare
* @param expected value to compare
* @param waitInSeconds maximum wait time
* @param intervalInMilliSeconds interval between retries in milliseconds
* @param message information about the propose of this verification
* @param params parameters in case if message is a format {@link String#format}
* @param type of map key
* @param type of map value
*/
public void notContainsAll(Map actual,
Map expected,
final int waitInSeconds,
final int intervalInMilliSeconds,
final String message,
final Object... params) {
toVerifier(actual).verifyNotContainsAll(verifier, expected, waitInSeconds, intervalInMilliSeconds, message, params);
}
private CMapVerifier toVerifier(Map actual) {
return () -> actual;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy