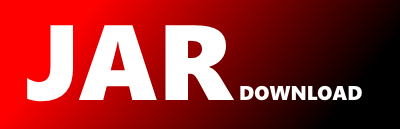
org.catools.common.security.CCipher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
The common objects which use in all other CATools projects
package org.catools.common.security;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Arrays;
import java.util.Base64;
public class CCipher {
public static String decrypt(String strToDecrypt, String secret) {
try {
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5PADDING");
cipher.init(Cipher.DECRYPT_MODE, getEncryptionKey(secret));
return new String(cipher.doFinal(Base64.getDecoder().decode(strToDecrypt)));
} catch (Exception e) {
throw new CCipherException("decrypt", strToDecrypt, e);
}
}
public static String encrypt(String strToEncrypt, String secret) {
try {
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, getEncryptionKey(secret));
return Base64.getEncoder().encodeToString(cipher.doFinal(strToEncrypt.getBytes(StandardCharsets.UTF_8)));
} catch (Exception e) {
throw new CCipherException("encrypt", strToEncrypt, e);
}
}
private static SecretKeySpec getEncryptionKey(String myKey) throws NoSuchAlgorithmException {
MessageDigest sha;
byte[] key = myKey.getBytes(StandardCharsets.UTF_8);
sha = MessageDigest.getInstance("SHA-1");
key = sha.digest(key);
key = Arrays.copyOf(key, 16);
return new SecretKeySpec(key, "AES");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy