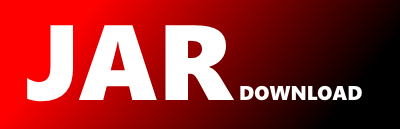
org.catools.common.testng.model.CTestResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
The common objects which use in all other CATools projects
package org.catools.common.testng.model;
import com.fasterxml.jackson.annotation.JsonFormat;
import org.catools.common.annotations.*;
import org.catools.common.collections.CList;
import org.catools.common.date.CDate;
import org.catools.common.exception.CExceptionInfo;
import org.catools.common.text.CStringUtil;
import org.testng.ITestResult;
import org.testng.annotations.Test;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.Date;
import java.util.Objects;
public class CTestResult implements Comparable {
private int testExecutionId;
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd'T'HH:mm:ssZ")
private CDate startTime;
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd'T'HH:mm:ssZ")
private CDate endTime;
private String packageName;
private String className;
private String methodName;
private CExecutionStatus status;
private Object[] annotations;
private Object[] parameters;
private CExceptionInfo exceptionInfo;
private long duration;
private String name;
private String description;
private String host;
private CList methodGroups = new CList<>();
private CList testIds = new CList();
private CList defectIds = new CList();
private CList openDefectIds = new CList();
private CList deferredId = new CList<>();
private String awaiting = CStringUtil.EMPTY;
private boolean configurationMethod = true;
private String project;
private String version;
private Integer severityLevel = null;
private Integer regressionDepth = null;
public CTestResult() {
}
public CTestResult(String project, String version, ITestResult testResult) {
this.project = project;
this.version = version;
this.className = testResult.getMethod().getTestClass().getName();
this.packageName = testResult.getMethod().getRealClass().getPackage().getName();
if (testResult.getMethod().getGroups() != null) {
this.methodGroups.addAll(Arrays.asList(testResult.getMethod().getGroups()));
}
Method method = testResult.getMethod().getConstructorOrMethod().getMethod();
this.methodName = method.getName();
this.annotations = method.getAnnotations();
this.parameters = testResult.getParameters();
this.exceptionInfo = new CExceptionInfo(testResult.getThrowable());
this.duration = testResult.getEndMillis() - testResult.getStartMillis();
this.name = testResult.getName();
this.host = testResult.getHost();
this.description = testResult.getMethod().getDescription();
this.startTime = new CDate(testResult.getStartMillis());
this.endTime = new CDate(testResult.getEndMillis());
boolean ignored = false;
for (Object annotation : this.annotations) {
if (annotation instanceof CTestIds) {
this.testIds.addAll(Arrays.asList(((CTestIds) annotation).ids()));
} else if (annotation instanceof CDeferred) {
this.deferredId.addAll(Arrays.asList(((CDeferred) annotation).ids()));
} else if (annotation instanceof CDefects) {
this.defectIds.addAll(Arrays.asList(((CDefects) annotation).ids()));
} else if (annotation instanceof COpenDefects) {
this.openDefectIds.addAll(Arrays.asList(((COpenDefects) annotation).ids()));
} else if (annotation instanceof CAwaiting) {
this.awaiting = ((CAwaiting) annotation).cause();
} else if (annotation instanceof CIgnored) {
ignored = true;
} else if (annotation instanceof CRegression) {
this.regressionDepth = ((CRegression) annotation).depth();
} else if (annotation instanceof CSeverity) {
this.severityLevel = ((CSeverity) annotation).level();
} else if (annotation instanceof Test) {
this.configurationMethod = false;
}
}
if (testResult.getStatus() == ITestResult.SUCCESS) {
this.status = CExecutionStatus.SUCCESS;
} else if (!awaiting.isEmpty()) {
this.status = CExecutionStatus.AWAITING;
} else {
if (ignored) {
this.status = CExecutionStatus.IGNORED;
} else if (!deferredId.isEmpty()) {
this.status = CExecutionStatus.DEFERRED;
} else if (testResult.getStatus() == ITestResult.FAILURE) {
this.status = CExecutionStatus.FAILURE;
} else {
this.status = CExecutionStatus.SKIP;
}
}
// set it at the end to make sure we have all values we need
this.testExecutionId = hashCode();
}
public Object[] getAnnotations() {
return annotations;
}
public String getAwaiting() {
return awaiting;
}
public String getClassName() {
return className;
}
public CList getDefectIds() {
return defectIds;
}
public CList getDeferredIds() {
return deferredId;
}
public String getDescription() {
return description;
}
public long getDuration() {
return duration;
}
public Date getEndTime() {
return endTime;
}
public CExceptionInfo getExceptionInfo() {
return exceptionInfo;
}
public String getFullName() {
return className + "." + name;
}
public String getHost() {
return host;
}
public CList getTestIds() {
return testIds;
}
public CList getMethodGroups() {
return methodGroups;
}
public String getMethodName() {
return methodName;
}
public String getName() {
return name;
}
public CList getOpenDefectIds() {
return openDefectIds;
}
public String getPackageName() {
return packageName;
}
public Object[] getParameters() {
return parameters;
}
public String getProject() {
return project;
}
public Date getStartTime() {
return startTime;
}
public CExecutionStatus getStatus() {
return status;
}
public int getTestExecutionId() {
return testExecutionId;
}
public String getVersion() {
return version;
}
public boolean hasException() {
return exceptionInfo != null && CStringUtil.isNotBlank(exceptionInfo.getType());
}
public boolean isConfigurationMethod() {
return configurationMethod;
}
public Integer getSeverityLevel() {
return severityLevel;
}
public Integer getRegressionDepth() {
return regressionDepth;
}
public String getTestFullName() {
return getPackageName() + "." + getClassName() + "::" + getMethodName();
}
@Override
public int compareTo(CTestResult o) {
if (o == null) {
return -1;
}
return toString().compareTo(o.toString());
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CTestResult that = (CTestResult) o;
return testExecutionId == that.testExecutionId &&
duration == that.duration &&
configurationMethod == that.configurationMethod &&
Objects.equals(startTime, that.startTime) &&
Objects.equals(endTime, that.endTime) &&
Objects.equals(packageName, that.packageName) &&
Objects.equals(className, that.className) &&
Objects.equals(methodName, that.methodName) &&
status == that.status &&
Arrays.equals(annotations, that.annotations) &&
Arrays.equals(parameters, that.parameters) &&
Objects.equals(exceptionInfo, that.exceptionInfo) &&
Objects.equals(name, that.name) &&
Objects.equals(description, that.description) &&
Objects.equals(host, that.host) &&
Objects.equals(methodGroups, that.methodGroups) &&
Objects.equals(testIds, that.testIds) &&
Objects.equals(defectIds, that.defectIds) &&
Objects.equals(openDefectIds, that.openDefectIds) &&
Objects.equals(deferredId, that.deferredId) &&
Objects.equals(awaiting, that.awaiting) &&
Objects.equals(project, that.project) &&
Objects.equals(version, that.version) &&
Objects.equals(severityLevel, that.severityLevel) &&
Objects.equals(regressionDepth, that.regressionDepth);
}
@Override
public int hashCode() {
int result = Objects.hash(testExecutionId,
startTime,
endTime,
packageName,
className,
methodName,
status,
exceptionInfo,
duration,
name,
description,
host,
methodGroups,
testIds,
defectIds,
openDefectIds,
deferredId,
awaiting,
configurationMethod,
project,
version,
severityLevel,
regressionDepth);
result = 31 * result + Arrays.hashCode(annotations);
result = 31 * result + Arrays.hashCode(parameters);
return result;
}
@Override
public String toString() {
return "CTestResult{" +
"testExecutionId=" + testExecutionId +
", startTime=" + startTime +
", endTime=" + endTime +
", packageName='" + packageName + '\'' +
", className='" + className + '\'' +
", methodName='" + methodName + '\'' +
", status=" + status +
", annotations=" + Arrays.toString(annotations) +
", parameters=" + Arrays.toString(parameters) +
", exceptionInfo=" + exceptionInfo +
", duration=" + duration +
", name='" + name + '\'' +
", description='" + description + '\'' +
", host='" + host + '\'' +
", methodGroups=" + methodGroups +
", testIds=" + testIds +
", defectIds=" + defectIds +
", openDefectIds=" + openDefectIds +
", deferredId=" + deferredId +
", awaiting='" + awaiting + '\'' +
", configurationMethod=" + configurationMethod +
", project='" + project + '\'' +
", version='" + version + '\'' +
", severityLevel=" + severityLevel +
", regressionDepth=" + regressionDepth +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy