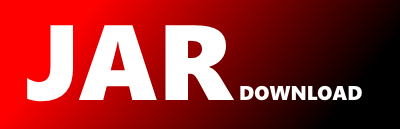
org.catools.etl.model.CEtlExecution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of etl Show documentation
Show all versions of etl Show documentation
The base ETL to be used in other CATools project as a base contract.
package org.catools.etl.model;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.experimental.Accessors;
import org.catools.common.text.CStringUtil;
import javax.persistence.*;
import java.io.Serializable;
import java.util.Date;
@Entity
@Table(name = "execution", schema = "etl")
@Data
@NoArgsConstructor
@AllArgsConstructor
@Accessors(chain = true)
public class CEtlExecution implements Serializable {
private static final long serialVersionUID = 6051874018285613707L;
@Id
@Column(name = "id", length = 20, unique = true, nullable = false)
private String id;
@Column(name = "created", nullable = false)
private Date created;
@Column(name = "executed")
private Date executed;
@Column(name = "execution_defect_count")
private Long executionDefectCount;
@Column(name = "step_defect_count")
private Long stepDefectCount;
@Column(name = "total_defect_count")
private Long totalDefectCount;
@Column(name = "comment", length = 1000)
private String comment;
@ManyToOne(targetEntity = CEtlItem.class)
@JoinColumn(name = "item_id", referencedColumnName = "id", nullable = false, foreignKey = @ForeignKey(name = "FK_EXECUTION_ITEM"))
private CEtlItem item;
@ManyToOne(targetEntity = CEtlCycle.class)
@JoinColumn(name = "cycle_id", referencedColumnName = "id", nullable = false, foreignKey = @ForeignKey(name = "FK_EXECUTION_CYCLE"))
private CEtlCycle cycle;
@ManyToOne(targetEntity = CEtlExecutionStatus.class)
@JoinColumn(name = "status_id", referencedColumnName = "id", nullable = false, foreignKey = @ForeignKey(name = "FK_EXECUTION_STATUS"))
private CEtlExecutionStatus status;
@ManyToOne(targetEntity = CEtlUser.class)
@JoinColumn(name = "executor_id", referencedColumnName = "name", foreignKey = @ForeignKey(name = "FK_EXECUTION_USER"))
private CEtlUser executor;
public CEtlExecution(String id,
CEtlItem item,
CEtlCycle cycle,
Date created,
Date executed,
CEtlUser executor,
CEtlExecutionStatus status,
Long executionDefectCount,
Long stepDefectCount,
Long totalDefectCount,
String comment) {
this.id = id;
this.item = item;
this.cycle = cycle;
this.created = created;
this.executed = executed;
this.executor = executor;
this.status = status;
this.executionDefectCount = executionDefectCount;
this.stepDefectCount = stepDefectCount;
this.totalDefectCount = totalDefectCount;
this.comment = CStringUtil.substring(comment, 0, 1000);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy