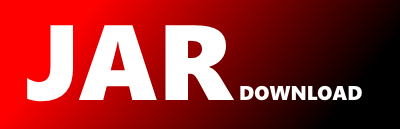
org.cdecode.firebase.api.auth.FirebaseAuth Maven / Gradle / Ivy
/*
* Copyright 2017 Google Inc. Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License
* for the specific language governing permissions and limitations under the License.
*/
package org.cdecode.firebase.api.auth;
import java.util.Map;
import org.cdecode.firebase.api.auth.UserRecord.CreateRequest;
import org.cdecode.firebase.api.auth.UserRecord.UpdateRequest;
import org.cdecode.firebase.api.tasks.Task;
import org.osgi.annotation.versioning.ProviderType;
/**
* This class is the entry point for all server-side Firebase Authentication actions.
*/
@ProviderType
public interface FirebaseAuth {
/**
* Creates a Firebase Custom Token associated with the given UID. This token can then be provided back to a client application for use with the
* signInWithCustomToken authentication API.
*
* @param uid The UID to store in the token. This identifies the user to other Firebase services (Firebase Database, Firebase Auth, etc.)
* @return A {@link Task} which will complete successfully with the created Firebase Custom Token, or unsuccessfully with the failure Exception.
*/
public Task createCustomToken(String uid);
/**
* Creates a Firebase Custom Token associated with the given UID and additionally containing the specified developerClaims. This token can then be provided
* back to a client application for use with the signInWithCustomToken authentication API.
*
* @param uid The UID to store in the token. This identifies the user to other Firebase services (Realtime Database, Storage, etc.). Should be less than 128
* characters.
* @param developerClaims Additional claims to be stored in the token (and made available to security rules in Database, Storage, etc.). These must be able
* to be serialized to JSON (e.g. contain only Maps, Arrays, Strings, Booleans, Numbers, etc.)
* @return A {@link Task} which will complete successfully with the created Firebase Custom Token, or unsuccessfully with the failure Exception.
*/
public Task createCustomToken(final String uid, final Map developerClaims);
/**
* Parses and verifies a Firebase ID Token.
*
* A Firebase application can identify itself to a trusted backend server by sending its Firebase ID Token (accessible via the getToken API in the Firebase
* Authentication client) with its request.
*
* The backend server can then use the verifyIdToken() method to verify the token is valid, meaning: the token is properly signed, has not expired, and it
* was issued for the project associated with this FirebaseAuth instance (which by default is extracted from your service account)
*
* If the token is valid, the returned {@link Task} will complete successfully and provide a parsed version of the token from which the UID and other claims
* in the token can be inspected. If the token is invalid, the Task will fail with an exception indicating the failure.
*
* @param token A Firebase ID Token to verify and parse.
* @return A {@link Task} which will complete successfully with the parsed token, or unsuccessfully with the failure Exception.
*/
public Task verifyIdToken(final String token);
/**
* Gets the user data corresponding to the specified user ID.
*
* @param uid A user ID string.
* @return A {@link Task} which will complete successfully with a {@link UserRecord} instance. If an error occurs while retrieving user data or if the
* specified user ID does not exist, the task fails with a FirebaseAuthException.
* @throws IllegalArgumentException If the user ID string is null or empty.
*/
public Task getUser(final String uid);
/**
* Gets the user data corresponding to the specified user email.
*
* @param email A user email address string.
* @return A {@link Task} which will complete successfully with a {@link UserRecord} instance. If an error occurs while retrieving user data or if the email
* address does not correspond to a user, the task fails with a FirebaseAuthException.
* @throws IllegalArgumentException If the email is null or empty.
*/
public Task getUserByEmail(final String email);
/**
* Creates a new user account with the attributes contained in the specified {@link CreateRequest}.
*
* @param request A non-null {@link CreateRequest} instance.
* @return A {@link Task} which will complete successfully with a {@link UserRecord} instance corresponding to the newly created account. If an error occurs
* while creating the user account, the task fails with a FirebaseAuthException.
* @throws NullPointerException if the provided request is null.
*/
public Task createUser(final CreateRequest request);
/**
* Create a new {@link CreateRequest} to be used in {@link FirebaseAuth#createUser(CreateRequest)}.
*
* @return a new {@code CreateRequest}, not null
*/
public CreateRequest getCreateRequest();
/**
* Updates an existing user account with the attributes contained in the specified {@link UpdateRequest}.
*
* @param request A non-null {@link UpdateRequest} instance.
* @return A {@link Task} which will complete successfully with a {@link UserRecord} instance corresponding to the updated user account. If an error occurs
* while updating the user account, the task fails with a FirebaseAuthException.
* @throws NullPointerException if the provided update request is null.
*/
public Task updateUser(final UpdateRequest request);
/**
* Deletes the user identified by the specified user ID.
*
* @param uid A user ID string.
* @return A {@link Task} which will complete successfully when the specified user account has been deleted. If an error occurs while deleting the user
* account, the task fails with a FirebaseAuthException.
* @throws IllegalArgumentException If the user ID string is null or empty.
*/
public Task deleteUser(final String uid);
}