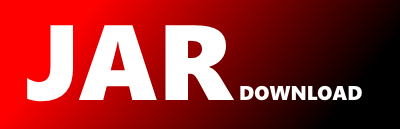
org.cdecode.firebase.api.database.FirebaseDatabase Maven / Gradle / Ivy
Show all versions of org.cdecode.firebase.api Show documentation
/*
* Copyright 2017 Google Inc. Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License
* for the specific language governing permissions and limitations under the License.
*/
package org.cdecode.firebase.api.database;
import org.osgi.annotation.versioning.ProviderType;
/**
* The entry point for accessing a Firebase Database.
*/
@ProviderType
public interface FirebaseDatabase {
/**
* Gets a DatabaseReference for the database root node.
*
* @return A DatabaseReference pointing to the root node.
*/
public DatabaseReference getReference();
/**
* Gets a DatabaseReference for the provided path.
*
* @param path Path to a location in your FirebaseDatabase.
* @return A DatabaseReference pointing to the specified path.
*/
public DatabaseReference getReference(final String path);
/**
* Gets a DatabaseReference for the provided URL. The URL must be a URL to a path within this FirebaseDatabase.
*
* @param url A URL to a path within your database.
* @return A DatabaseReference for the provided URL.
*/
public DatabaseReference getReferenceFromUrl(final String url);
/**
* The Firebase Database client automatically queues writes and sends them to the server at the earliest opportunity, depending on network connectivity. In
* some cases (e.g. offline usage) there may be a large number of writes waiting to be sent. Calling this method will purge all outstanding writes so they
* are abandoned.
*
* All writes will be purged, including transactions and {@link DatabaseReference#onDisconnect} writes. The writes will be rolled back locally, perhaps
* triggering events for affected event listeners, and the client will not (re-)send them to the Firebase backend.
*/
public void purgeOutstandingWrites();
/**
* Resumes our connection to the Firebase Database backend after a previous {@link #goOffline()} call.
*/
public void goOnline();
/**
* Shuts down our connection to the Firebase Database backend until {@link #goOnline()} is called.
*/
public void goOffline();
/**
* The Firebase Database client will cache synchronized data and keep track of all writes you've initiated while your application is running. It seamlessly
* handles intermittent network connections and re-sends write operations when the network connection is restored.
*
* However by default your write operations and cached data are only stored in-memory and will be lost when your app restarts. By setting this value to
* `true`, the data will be persisted to on-device (disk) storage and will thus be available again when the app is restarted (even when there is no network
* connectivity at that time). Note that this method must be called before creating your first Database reference and only needs to be called once per
* application.
*
* @param isEnabled Set to true to enable disk persistence, set to false to disable it.
*/
public void setPersistenceEnabled(final boolean isEnabled);
/**
* By default Firebase Database will use up to 10MB of disk space to cache data. If the cache grows beyond this size, Firebase Database will start removing
* data that hasn't been recently used. If you find that your application caches too little or too much data, call this method to change the cache size.
* This method must be called before creating your first Database reference and only needs to be called once per application.
*
* Note that the specified cache size is only an approximation and the size on disk may temporarily exceed it at times. Cache sizes smaller than 1 MB or
* greater than 100 MB are not supported.
*
* @param cacheSizeInBytes The new size of the cache in bytes.
*/
public void setPersistenceCacheSizeBytes(final long cacheSizeInBytes);
}