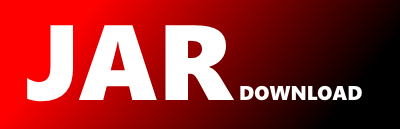
org.cdecode.firebase.api.database.OnDisconnect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.cdecode.firebase.api Show documentation
Show all versions of org.cdecode.firebase.api Show documentation
Provides an API for the Firebase Admin SDK without dependency.
The newest version!
/*
* Copyright 2017 Google Inc. Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License
* for the specific language governing permissions and limitations under the License.
*/
package org.cdecode.firebase.api.database;
import java.util.Map;
import org.cdecode.firebase.api.database.DatabaseReference.CompletionListener;
import org.cdecode.firebase.api.tasks.Task;
/**
* The OnDisconnect interface is used to manage operations that will be run on the server when this client disconnects. It can be used to add or remove data
* based on a client's connection status. It is very useful in applications looking for 'presence' functionality.
*
* Instances of this class are obtained by calling {@link DatabaseReference#onDisconnect() onDisconnect} on a Firebase Database ref.
*/
@SuppressWarnings("rawtypes")
public interface OnDisconnect {
/**
* Ensure the data at this location is set to the specified value when the client is disconnected (due to closing the browser, navigating to a new page, or
* network issues).
*
* This method is especially useful for implementing "presence" systems, where a value should be changed or cleared when a user disconnects so that they
* appear "offline" to other users.
*
* @param value The value to be set when a disconnect occurs
* @return The {@link Task} for this operation.
*/
public Task setValue(Object value);
/**
* Ensure the data at this location is set to the specified value and priority when the client is disconnected (due to closing the browser, navigating to a
* new page, or network issues).
*
* This method is especially useful for implementing "presence" systems, where a value should be changed or cleared when a user disconnects so that they
* appear "offline" to other users.
*
* @param value The value to be set when a disconnect occurs
* @param priority The priority to be set when a disconnect occurs
* @return The {@link Task} for this operation.
*/
public Task setValue(Object value, String priority);
/**
* Ensure the data at this location is set to the specified value and priority when the client is disconnected (due to closing the browser, navigating to a
* new page, or network issues).
*
* This method is especially useful for implementing "presence" systems, where a value should be changed or cleared when a user disconnects so that they
* appear "offline" to other users.
*
* @param value The value to be set when a disconnect occurs
* @param priority The priority to be set when a disconnect occurs
* @return The {@link Task} for this operation.
*/
public Task setValue(Object value, double priority);
/**
* Ensure the data at this location is set to the specified value when the client is disconnected (due to closing the browser, navigating to a new page, or
* network issues).
*
* This method is especially useful for implementing "presence" systems, where a value should be changed or cleared when a user disconnects so that they
* appear "offline" to other users.
*
* @param value The value to be set when a disconnect occurs
* @param listener A listener that will be triggered once the server has queued up the operation
*/
public void setValue(Object value, CompletionListener listener);
/**
* Ensure the data at this location is set to the specified value and priority when the client is disconnected (due to closing the browser, navigating to a
* new page, or network issues).
*
* This method is especially useful for implementing "presence" systems, where a value should be changed or cleared when a user disconnects so that they
* appear "offline" to other users.
*
* @param value The value to be set when a disconnect occurs
* @param priority The priority to be set when a disconnect occurs
* @param listener A listener that will be triggered once the server has queued up the operation
*/
public void setValue(Object value, String priority, CompletionListener listener);
/**
* Ensure the data at this location is set to the specified value and priority when the client is disconnected (due to closing the browser, navigating to a
* new page, or network issues).
*
* This method is especially useful for implementing "presence" systems, where a value should be changed or cleared when a user disconnects so that they
* appear "offline" to other users.
*
* @param value The value to be set when a disconnect occurs
* @param priority The priority to be set when a disconnect occurs
* @param listener A listener that will be triggered once the server has queued up the operation
*/
public void setValue(Object value, double priority, CompletionListener listener);
/**
* Ensure the data at this location is set to the specified value and priority when the client is disconnected (due to closing the browser, navigating to a
* new page, or network issues).
*
* This method is especially useful for implementing "presence" systems, where a value should be changed or cleared when a user disconnects so that they
* appear "offline" to other users.
*
* @param value The value to be set when a disconnect occurs
* @param priority The priority to be set when a disconnect occurs
* @param listener A listener that will be triggered once the server has queued up the operation
*/
public void setValue(Object value, Map priority, CompletionListener listener);
// Update
/**
* Ensure the data has the specified child values updated when the client is disconnected
*
* @param update The paths to update, along with their desired values
* @return The {@link Task} for this operation.
*/
public Task updateChildren(Map update);
/**
* Ensure the data has the specified child values updated when the client is disconnected
*
* @param update The paths to update, along with their desired values
* @param listener A listener that will be triggered once the server has queued up the operation
*/
public void updateChildren(final Map update, final CompletionListener listener);
// Remove
/**
* Remove the value at this location when the client disconnects
*
* @return The {@link Task} for this operation.
*/
public Task removeValue();
/**
* Remove the value at this location when the client disconnects
*
* @param listener A listener that will be triggered once the server has queued up the operation
*/
public void removeValue(CompletionListener listener);
// Cancel the operation
/**
* Cancel any disconnect operations that are queued up at this location
*
* @return The {@link Task} for this operation.
*/
public Task cancel();
/**
* Cancel any disconnect operations that are queued up at this location
*
* @param listener A listener that will be triggered once the server has cancelled the operations
*/
public void cancel(final CompletionListener listener);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy