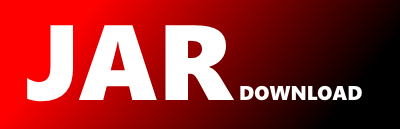
org.cdecode.firebase.api.tasks.Task Maven / Gradle / Ivy
/*
* Copyright 2017 Google Inc. Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License
* for the specific language governing permissions and limitations under the License.
*/
package org.cdecode.firebase.api.tasks;
import java.util.concurrent.Executor;
/**
* Represents an asynchronous operation.
*
* @param the type of the result of the operation
*/
public interface Task {
/**
* @return {@code true} if the Task is complete; {@code false} otherwise
*/
public boolean isComplete();
/**
* @return {@code true} if the Task has completed successfully; {@code false} otherwise
*/
public boolean isSuccessful();
/**
* @return the result of the Task, if it has already completed
* @throws IllegalStateException if the Task is not yet complete
* @throws RuntimeExecutionException if the Task failed with an exception
*/
public T getResult();
/**
* @param exceptionType the {@link Throwable} type to throw
* @param the {@link Throwable} type to throw
* @return the result of the Task, if it has already completed
* @throws IllegalStateException if the Task is not yet complete
* @throws X if the Task failed with an exception of type X
* @throws RuntimeExecutionException if the Task failed with an exception that was not of type X
*/
public T getResult(Class exceptionType)
throws X;
/**
* @return the exception that caused the Task to fail. Returns {@code null} if the Task is not yet complete, or completed successfully
*/
public Exception getException();
/**
* Adds a listener that is called if the Task completes successfully.
*
* The listener will be called on a shared thread pool. If the Task has already completed successfully, a call to the listener will be immediately
* scheduled. If multiple listeners are added, they will be called in the order in which they were added.
*
* @param listener the listener to call
* @return this Task
*/
public Task addOnSuccessListener(OnSuccessListener super T> listener);
/**
* Adds a listener that is called if the Task completes successfully.
*
* If multiple listeners are added, they will be called in the order in which they were added. If the Task has already completed successfully, a call to the
* listener will be immediately scheduled.
*
* @param executor the executor to use to call the listener
* @param listener the listener to call
* @return this Task
*/
public Task addOnSuccessListener(Executor executor, OnSuccessListener super T> listener);
/**
* Adds a listener that is called if the Task fails.
*
* The listener will be called on a shared thread pool. If the Task has already failed, a call to the listener will be immediately scheduled. If multiple
* listeners are added, they will be called in the order in which they were added.
*
* @param listener the listener to call
* @return this Task
*/
public Task addOnFailureListener(OnFailureListener listener);
/**
* Adds a listener that is called if the Task fails.
*
* If the Task has already failed, a call to the listener will be immediately scheduled. If multiple listeners are added, they will be called in the order
* in which they were added.
*
* @param executor the executor to use to call the listener
* @param listener the listener to call
* @return this Task
*/
public Task addOnFailureListener(Executor executor, OnFailureListener listener);
/**
* Adds a listener that is called when the Task completes.
*
* The listener will be called on a shared thread pool. If the Task is already complete, a call to the listener will be immediately scheduled. If multiple
* listeners are added, they will be called in the order in which they were added.
*
* @param listener the listener to call
* @return this Task
*/
default public Task addOnCompleteListener(final OnCompleteListener listener) {
throw new UnsupportedOperationException("addOnCompleteListener is not implemented");
}
/**
* Adds a listener that is called when the Task completes.
*
* If the Task is already complete, a call to the listener will be immediately scheduled. If multiple listeners are added, they will be called in the order
* in which they were added.
*
* @param executor the executor to use to call the listener
* @param listener the listener to call
* @return this Task
*/
default public Task addOnCompleteListener(final Executor executor, final OnCompleteListener listener) {
throw new UnsupportedOperationException("addOnCompleteListener is not implemented");
}
/**
* Returns a new Task that will be completed with the result of applying the specified Continuation to this Task.
*
* If the Continuation throws an exception, the returned Task will fail with that exception.
*
* The Continuation will be called on a shared thread pool.
*
* @param the task type
* @param continuation the continuation
* @return a new Task that will be completed with the result
*/
default public Task continueWith(final Continuation continuation) {
throw new UnsupportedOperationException("continueWith is not implemented");
}
/**
* Returns a new Task that will be completed with the result of applying the specified Continuation to this Task.
*
* If the Continuation throws an exception, the returned Task will fail with that exception.
*
* @param the task type
* @param executor the executor to use to call the Continuation
* @param continuation the continuation
* @return a new Task that will be completed
* @see Continuation#then(Task)
*/
default public Task continueWith(final Executor executor, final Continuation continuation) {
throw new UnsupportedOperationException("continueWith is not implemented");
}
/**
* Returns a new Task that will be completed with the result of applying the specified Continuation to this Task.
*
* If the Continuation throws an exception, the returned Task will fail with that exception.
*
* The Continuation will be called on a shared thread pool.
*
* @param the task type
* @param continuation the continuation
* @return a new Task that will be completed
*/
default public Task continueWithTask(final Continuation> continuation) {
throw new UnsupportedOperationException("continueWithTask is not implemented");
}
/**
* Returns a new Task that will be completed with the result of applying the specified Continuation to this Task.
*
* If the Continuation throws an exception, the returned Task will fail with that exception.
*
* @param the task type
* @param executor the executor to use to call the Continuation
* @param continuation the continuation
* @return a new Task that will be completed
* @see Continuation#then(Task)
*/
default public Task continueWithTask(final Executor executor, final Continuation> continuation) {
throw new UnsupportedOperationException("continueWithTask is not implemented");
}
}