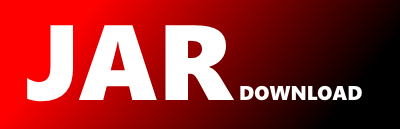
org.cdecode.firebase.api.tasks.Tasks Maven / Gradle / Ivy
/*
* Copyright 2017 Google Inc. Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License
* for the specific language governing permissions and limitations under the License.
*/
package org.cdecode.firebase.api.tasks;
import java.util.Collection;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Executor;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import org.osgi.annotation.versioning.ProviderType;
/**
* {@link Task} utility methods.
*/
@ProviderType
public interface Tasks {
/**
* Returns a completed Task with the specified result.
*
* @param result the result
* @param the task type
* @return a Task that will be completed
*/
public Task forResult(final T result);
/**
* Returns a completed Task with the specified exception.
*
* @param exception the exception
* @param the task type
* @return a Task that will be completed
*/
public Task forException(final Exception exception);
/**
* Returns a Task that will be completed with the result of the specified Callable.
*
* The Callable will be called on a shared thread pool.
*
* @param callable the callable
* @param the task type
* @return a Task that will be completed
*/
public Task call(final Callable callable);
/**
* Returns a Task that will be completed with the result of the specified Callable.
*
* @param the task type
* @param executor the Executor to use to call the Callable
* @param callable the Callable to call
* @return a Task that will be completed
*/
public Task call(final Executor executor, final Callable callable);
/**
* Blocks until the specified Task is complete.
*
* @param task the task to wait
* @param the task type
* @return the Task's result
* @throws ExecutionException if the Task fails
* @throws InterruptedException if an interrupt occurs while waiting for the Task to complete
*/
public T await(final Task task)
throws ExecutionException, InterruptedException;
/**
* Blocks until the specified Task is complete.
*
* @param task the task to wait
* @param timeout the timeout
* @param unit unit of time
* @param the task type
* @return the Task's result
* @throws ExecutionException if the Task fails
* @throws InterruptedException if an interrupt occurs while waiting for the Task to complete
* @throws TimeoutException if the specified timeout is reached before the Task completes
*/
public T await(final Task task, final long timeout, final TimeUnit unit)
throws ExecutionException, InterruptedException, TimeoutException;
/**
* Returns a Task that completes successfully when all of the specified Tasks complete successfully. Does not accept nulls.
*
* @param tasks the non null tasks
* @return a Task that completes successfully when all of the specified Tasks complete successfully. Does not accept nulls.
* @throws NullPointerException if any of the provided Tasks are null
*/
public Task whenAll(final Collection extends Task>> tasks);
/**
* Returns a Task that completes successfully when all of the specified Tasks complete successfully. Does not accept nulls.
*
* @param tasks the non null tasks
* @return a Task that completes successfully when all of the specified Tasks complete successfully. Does not accept nulls.
* @throws NullPointerException if any of the provided Tasks are null
*/
public Task whenAll(final Task>... tasks);
}