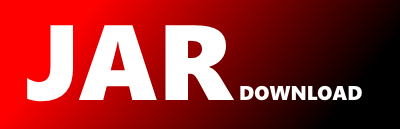
org.cdk8s.jenkins.JenkinsProps Maven / Gradle / Ivy
Show all versions of cdk8s-jenkins Show documentation
package org.cdk8s.jenkins;
/**
* Props for Jenkins
.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.105.0 (build 0a2adcb)", date = "2024-11-16T12:06:17.282Z")
@software.amazon.jsii.Jsii(module = org.cdk8s.jenkins.$Module.class, fqn = "cdk8s-jenkins.JenkinsProps")
@software.amazon.jsii.Jsii.Proxy(JenkinsProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface JenkinsProps extends software.amazon.jsii.JsiiSerializable {
/**
* List of plugins required by Jenkins operator.
*
* Default: - Default base plugins:
* { name: 'kubernetes', version: '1.31.3' },
* { name: 'workflow-job', version: '1145.v7f2433caa07f' },
* { name: 'workflow-aggregator', version: '2.6' },
* { name: 'git', version: '4.10.3' },
* { name: 'job-dsl', version: '1.78.1' },
* { name: 'configuration-as-code', version: '1414.v878271fc496f' },
* { name: 'kubernetes-credentials-provider', version: '0.20' }
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getBasePlugins() {
return null;
}
/**
* Toggle for CSRF Protection on Jenkins resource.
*
* Default: - false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getDisableCsrfProtection() {
return null;
}
/**
* Metadata associated with Jenkins resource.
*
* Default: : Default metadata values:
* {
* name: An app-unique name generated by the chart,
* annotations: No annotations,
* labels: { app: 'jenkins' },
* namespace: default,
* finalizers: No finalizers,
* ownerReferences: Automatically set by Kubernetes
* }
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable org.cdk8s.ApiObjectMetadata getMetadata() {
return null;
}
/**
* List of custom plugins applied to Jenkins resource.
*
* Default: - []
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getPlugins() {
return null;
}
/**
* List of seed job configuration for Jenkins resource.
*
* For more information about seed jobs, please take a look at { @link https://github.com/jenkinsci/job-dsl-plugin/wiki/Tutorial---Using-the-Jenkins-Job-DSL Jenkins Seed Jobs Documentation }.
*
* Default: - No seed jobs
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getSeedJobs() {
return null;
}
/**
* @return a {@link Builder} of {@link JenkinsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link JenkinsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.util.List basePlugins;
java.lang.Boolean disableCsrfProtection;
org.cdk8s.ApiObjectMetadata metadata;
java.util.List plugins;
java.util.List seedJobs;
/**
* Sets the value of {@link JenkinsProps#getBasePlugins}
* @param basePlugins List of plugins required by Jenkins operator.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder basePlugins(java.util.List extends org.cdk8s.jenkins.Plugin> basePlugins) {
this.basePlugins = (java.util.List)basePlugins;
return this;
}
/**
* Sets the value of {@link JenkinsProps#getDisableCsrfProtection}
* @param disableCsrfProtection Toggle for CSRF Protection on Jenkins resource.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder disableCsrfProtection(java.lang.Boolean disableCsrfProtection) {
this.disableCsrfProtection = disableCsrfProtection;
return this;
}
/**
* Sets the value of {@link JenkinsProps#getMetadata}
* @param metadata Metadata associated with Jenkins resource.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metadata(org.cdk8s.ApiObjectMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Sets the value of {@link JenkinsProps#getPlugins}
* @param plugins List of custom plugins applied to Jenkins resource.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder plugins(java.util.List extends org.cdk8s.jenkins.Plugin> plugins) {
this.plugins = (java.util.List)plugins;
return this;
}
/**
* Sets the value of {@link JenkinsProps#getSeedJobs}
* @param seedJobs List of seed job configuration for Jenkins resource.
* For more information about seed jobs, please take a look at { @link https://github.com/jenkinsci/job-dsl-plugin/wiki/Tutorial---Using-the-Jenkins-Job-DSL Jenkins Seed Jobs Documentation }.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder seedJobs(java.util.List extends org.cdk8s.jenkins.SeedJob> seedJobs) {
this.seedJobs = (java.util.List)seedJobs;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link JenkinsProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public JenkinsProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link JenkinsProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements JenkinsProps {
private final java.util.List basePlugins;
private final java.lang.Boolean disableCsrfProtection;
private final org.cdk8s.ApiObjectMetadata metadata;
private final java.util.List plugins;
private final java.util.List seedJobs;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.basePlugins = software.amazon.jsii.Kernel.get(this, "basePlugins", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.jenkins.Plugin.class)));
this.disableCsrfProtection = software.amazon.jsii.Kernel.get(this, "disableCsrfProtection", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.metadata = software.amazon.jsii.Kernel.get(this, "metadata", software.amazon.jsii.NativeType.forClass(org.cdk8s.ApiObjectMetadata.class));
this.plugins = software.amazon.jsii.Kernel.get(this, "plugins", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.jenkins.Plugin.class)));
this.seedJobs = software.amazon.jsii.Kernel.get(this, "seedJobs", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.jenkins.SeedJob.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.basePlugins = (java.util.List)builder.basePlugins;
this.disableCsrfProtection = builder.disableCsrfProtection;
this.metadata = builder.metadata;
this.plugins = (java.util.List)builder.plugins;
this.seedJobs = (java.util.List)builder.seedJobs;
}
@Override
public final java.util.List getBasePlugins() {
return this.basePlugins;
}
@Override
public final java.lang.Boolean getDisableCsrfProtection() {
return this.disableCsrfProtection;
}
@Override
public final org.cdk8s.ApiObjectMetadata getMetadata() {
return this.metadata;
}
@Override
public final java.util.List getPlugins() {
return this.plugins;
}
@Override
public final java.util.List getSeedJobs() {
return this.seedJobs;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getBasePlugins() != null) {
data.set("basePlugins", om.valueToTree(this.getBasePlugins()));
}
if (this.getDisableCsrfProtection() != null) {
data.set("disableCsrfProtection", om.valueToTree(this.getDisableCsrfProtection()));
}
if (this.getMetadata() != null) {
data.set("metadata", om.valueToTree(this.getMetadata()));
}
if (this.getPlugins() != null) {
data.set("plugins", om.valueToTree(this.getPlugins()));
}
if (this.getSeedJobs() != null) {
data.set("seedJobs", om.valueToTree(this.getSeedJobs()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("cdk8s-jenkins.JenkinsProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
JenkinsProps.Jsii$Proxy that = (JenkinsProps.Jsii$Proxy) o;
if (this.basePlugins != null ? !this.basePlugins.equals(that.basePlugins) : that.basePlugins != null) return false;
if (this.disableCsrfProtection != null ? !this.disableCsrfProtection.equals(that.disableCsrfProtection) : that.disableCsrfProtection != null) return false;
if (this.metadata != null ? !this.metadata.equals(that.metadata) : that.metadata != null) return false;
if (this.plugins != null ? !this.plugins.equals(that.plugins) : that.plugins != null) return false;
return this.seedJobs != null ? this.seedJobs.equals(that.seedJobs) : that.seedJobs == null;
}
@Override
public final int hashCode() {
int result = this.basePlugins != null ? this.basePlugins.hashCode() : 0;
result = 31 * result + (this.disableCsrfProtection != null ? this.disableCsrfProtection.hashCode() : 0);
result = 31 * result + (this.metadata != null ? this.metadata.hashCode() : 0);
result = 31 * result + (this.plugins != null ? this.plugins.hashCode() : 0);
result = 31 * result + (this.seedJobs != null ? this.seedJobs.hashCode() : 0);
return result;
}
}
}