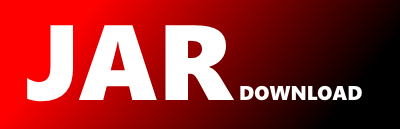
org.cdk8s.plus22.DeploymentProps Maven / Gradle / Ivy
Show all versions of cdk8s-plus-22 Show documentation
package org.cdk8s.plus22;
/**
* Properties for initialization of `Deployment`.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.47.0 (build 86d2c33)", date = "2021-12-08T00:16:55.089Z")
@software.amazon.jsii.Jsii(module = org.cdk8s.plus22.$Module.class, fqn = "cdk8s-plus-22.DeploymentProps")
@software.amazon.jsii.Jsii.Proxy(DeploymentProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface DeploymentProps extends software.amazon.jsii.JsiiSerializable, org.cdk8s.plus22.ResourceProps, org.cdk8s.plus22.PodTemplateProps {
/**
* Automatically allocates a pod selector for this deployment.
*
* If this is set to false
you must define your selector through
* deployment.podMetadata.addLabel()
and deployment.selectByLabel()
.
*
* Default: true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getDefaultSelector() {
return null;
}
/**
* Number of desired pods.
*
* Default: 1
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getReplicas() {
return null;
}
/**
* @return a {@link Builder} of {@link DeploymentProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DeploymentProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Boolean defaultSelector;
java.lang.Number replicas;
org.cdk8s.ApiObjectMetadata metadata;
org.cdk8s.ApiObjectMetadata podMetadata;
java.util.List containers;
org.cdk8s.plus22.RestartPolicy restartPolicy;
org.cdk8s.plus22.IServiceAccount serviceAccount;
java.util.List volumes;
/**
* Sets the value of {@link DeploymentProps#getDefaultSelector}
* @param defaultSelector Automatically allocates a pod selector for this deployment.
* If this is set to false
you must define your selector through
* deployment.podMetadata.addLabel()
and deployment.selectByLabel()
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder defaultSelector(java.lang.Boolean defaultSelector) {
this.defaultSelector = defaultSelector;
return this;
}
/**
* Sets the value of {@link DeploymentProps#getReplicas}
* @param replicas Number of desired pods.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder replicas(java.lang.Number replicas) {
this.replicas = replicas;
return this;
}
/**
* Sets the value of {@link DeploymentProps#getMetadata}
* @param metadata Metadata that all persisted resources must have, which includes all objects users must create.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metadata(org.cdk8s.ApiObjectMetadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Sets the value of {@link DeploymentProps#getPodMetadata}
* @param podMetadata The pod metadata.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder podMetadata(org.cdk8s.ApiObjectMetadata podMetadata) {
this.podMetadata = podMetadata;
return this;
}
/**
* Sets the value of {@link DeploymentProps#getContainers}
* @param containers List of containers belonging to the pod.
* Containers cannot currently be
* added or removed. There must be at least one container in a Pod.
*
* You can add additionnal containers using podSpec.addContainer()
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder containers(java.util.List extends org.cdk8s.plus22.ContainerProps> containers) {
this.containers = (java.util.List)containers;
return this;
}
/**
* Sets the value of {@link DeploymentProps#getRestartPolicy}
* @param restartPolicy Restart policy for all containers within the pod.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder restartPolicy(org.cdk8s.plus22.RestartPolicy restartPolicy) {
this.restartPolicy = restartPolicy;
return this;
}
/**
* Sets the value of {@link DeploymentProps#getServiceAccount}
* @param serviceAccount A service account provides an identity for processes that run in a Pod.
* When you (a human) access the cluster (for example, using kubectl), you are
* authenticated by the apiserver as a particular User Account (currently this
* is usually admin, unless your cluster administrator has customized your
* cluster). Processes in containers inside pods can also contact the
* apiserver. When they do, they are authenticated as a particular Service
* Account (for example, default).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceAccount(org.cdk8s.plus22.IServiceAccount serviceAccount) {
this.serviceAccount = serviceAccount;
return this;
}
/**
* Sets the value of {@link DeploymentProps#getVolumes}
* @param volumes List of volumes that can be mounted by containers belonging to the pod.
* You can also add volumes later using podSpec.addVolume()
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder volumes(java.util.List extends org.cdk8s.plus22.Volume> volumes) {
this.volumes = (java.util.List)volumes;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DeploymentProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DeploymentProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link DeploymentProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DeploymentProps {
private final java.lang.Boolean defaultSelector;
private final java.lang.Number replicas;
private final org.cdk8s.ApiObjectMetadata metadata;
private final org.cdk8s.ApiObjectMetadata podMetadata;
private final java.util.List containers;
private final org.cdk8s.plus22.RestartPolicy restartPolicy;
private final org.cdk8s.plus22.IServiceAccount serviceAccount;
private final java.util.List volumes;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.defaultSelector = software.amazon.jsii.Kernel.get(this, "defaultSelector", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.replicas = software.amazon.jsii.Kernel.get(this, "replicas", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.metadata = software.amazon.jsii.Kernel.get(this, "metadata", software.amazon.jsii.NativeType.forClass(org.cdk8s.ApiObjectMetadata.class));
this.podMetadata = software.amazon.jsii.Kernel.get(this, "podMetadata", software.amazon.jsii.NativeType.forClass(org.cdk8s.ApiObjectMetadata.class));
this.containers = software.amazon.jsii.Kernel.get(this, "containers", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.ContainerProps.class)));
this.restartPolicy = software.amazon.jsii.Kernel.get(this, "restartPolicy", software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.RestartPolicy.class));
this.serviceAccount = software.amazon.jsii.Kernel.get(this, "serviceAccount", software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.IServiceAccount.class));
this.volumes = software.amazon.jsii.Kernel.get(this, "volumes", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.Volume.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.defaultSelector = builder.defaultSelector;
this.replicas = builder.replicas;
this.metadata = builder.metadata;
this.podMetadata = builder.podMetadata;
this.containers = (java.util.List)builder.containers;
this.restartPolicy = builder.restartPolicy;
this.serviceAccount = builder.serviceAccount;
this.volumes = (java.util.List)builder.volumes;
}
@Override
public final java.lang.Boolean getDefaultSelector() {
return this.defaultSelector;
}
@Override
public final java.lang.Number getReplicas() {
return this.replicas;
}
@Override
public final org.cdk8s.ApiObjectMetadata getMetadata() {
return this.metadata;
}
@Override
public final org.cdk8s.ApiObjectMetadata getPodMetadata() {
return this.podMetadata;
}
@Override
public final java.util.List getContainers() {
return this.containers;
}
@Override
public final org.cdk8s.plus22.RestartPolicy getRestartPolicy() {
return this.restartPolicy;
}
@Override
public final org.cdk8s.plus22.IServiceAccount getServiceAccount() {
return this.serviceAccount;
}
@Override
public final java.util.List getVolumes() {
return this.volumes;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDefaultSelector() != null) {
data.set("defaultSelector", om.valueToTree(this.getDefaultSelector()));
}
if (this.getReplicas() != null) {
data.set("replicas", om.valueToTree(this.getReplicas()));
}
if (this.getMetadata() != null) {
data.set("metadata", om.valueToTree(this.getMetadata()));
}
if (this.getPodMetadata() != null) {
data.set("podMetadata", om.valueToTree(this.getPodMetadata()));
}
if (this.getContainers() != null) {
data.set("containers", om.valueToTree(this.getContainers()));
}
if (this.getRestartPolicy() != null) {
data.set("restartPolicy", om.valueToTree(this.getRestartPolicy()));
}
if (this.getServiceAccount() != null) {
data.set("serviceAccount", om.valueToTree(this.getServiceAccount()));
}
if (this.getVolumes() != null) {
data.set("volumes", om.valueToTree(this.getVolumes()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("cdk8s-plus-22.DeploymentProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DeploymentProps.Jsii$Proxy that = (DeploymentProps.Jsii$Proxy) o;
if (this.defaultSelector != null ? !this.defaultSelector.equals(that.defaultSelector) : that.defaultSelector != null) return false;
if (this.replicas != null ? !this.replicas.equals(that.replicas) : that.replicas != null) return false;
if (this.metadata != null ? !this.metadata.equals(that.metadata) : that.metadata != null) return false;
if (this.podMetadata != null ? !this.podMetadata.equals(that.podMetadata) : that.podMetadata != null) return false;
if (this.containers != null ? !this.containers.equals(that.containers) : that.containers != null) return false;
if (this.restartPolicy != null ? !this.restartPolicy.equals(that.restartPolicy) : that.restartPolicy != null) return false;
if (this.serviceAccount != null ? !this.serviceAccount.equals(that.serviceAccount) : that.serviceAccount != null) return false;
return this.volumes != null ? this.volumes.equals(that.volumes) : that.volumes == null;
}
@Override
public final int hashCode() {
int result = this.defaultSelector != null ? this.defaultSelector.hashCode() : 0;
result = 31 * result + (this.replicas != null ? this.replicas.hashCode() : 0);
result = 31 * result + (this.metadata != null ? this.metadata.hashCode() : 0);
result = 31 * result + (this.podMetadata != null ? this.podMetadata.hashCode() : 0);
result = 31 * result + (this.containers != null ? this.containers.hashCode() : 0);
result = 31 * result + (this.restartPolicy != null ? this.restartPolicy.hashCode() : 0);
result = 31 * result + (this.serviceAccount != null ? this.serviceAccount.hashCode() : 0);
result = 31 * result + (this.volumes != null ? this.volumes.hashCode() : 0);
return result;
}
}
}