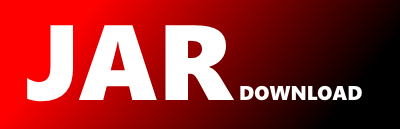
org.cdk8s.plus22.Ingress Maven / Gradle / Ivy
Show all versions of cdk8s-plus-22 Show documentation
package org.cdk8s.plus22;
/**
* Ingress is a collection of rules that allow inbound connections to reach the endpoints defined by a backend.
*
* An Ingress can be configured to give services
* externally-reachable urls, load balance traffic, terminate SSL, offer name
* based virtual hosting etc.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.47.0 (build 86d2c33)", date = "2021-12-08T00:16:55.100Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.cdk8s.plus22.$Module.class, fqn = "cdk8s-plus-22.Ingress")
public class Ingress extends org.cdk8s.plus22.Resource {
protected Ingress(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Ingress(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Ingress(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable org.cdk8s.plus22.IngressProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Ingress(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* Defines the default backend for this ingress.
*
* A default backend capable of
* servicing requests that don't match any rule.
*
* @param backend The backend to use for requests that do not match any rule. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addDefaultBackend(final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.IngressBackend backend) {
software.amazon.jsii.Kernel.call(this, "addDefaultBackend", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(backend, "backend is required") });
}
/**
* Specify a default backend for a specific host name.
*
* This backend will be used as a catch-all for requests
* targeted to this host name (the Host
header matches this value).
*
* @param host The host name to match. This parameter is required.
* @param backend The backend to route to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addHostDefaultBackend(final @org.jetbrains.annotations.NotNull java.lang.String host, final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.IngressBackend backend) {
software.amazon.jsii.Kernel.call(this, "addHostDefaultBackend", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(host, "host is required"), java.util.Objects.requireNonNull(backend, "backend is required") });
}
/**
* Adds an ingress rule applied to requests to a specific host and a specific HTTP path (the `Host` header matches this value).
*
* @param host The host name. This parameter is required.
* @param path The HTTP path. This parameter is required.
* @param backend The backend to route requests to. This parameter is required.
* @param pathType How the path is matched against request paths.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addHostRule(final @org.jetbrains.annotations.NotNull java.lang.String host, final @org.jetbrains.annotations.NotNull java.lang.String path, final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.IngressBackend backend, final @org.jetbrains.annotations.Nullable org.cdk8s.plus22.HttpIngressPathType pathType) {
software.amazon.jsii.Kernel.call(this, "addHostRule", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(host, "host is required"), java.util.Objects.requireNonNull(path, "path is required"), java.util.Objects.requireNonNull(backend, "backend is required"), pathType });
}
/**
* Adds an ingress rule applied to requests to a specific host and a specific HTTP path (the `Host` header matches this value).
*
* @param host The host name. This parameter is required.
* @param path The HTTP path. This parameter is required.
* @param backend The backend to route requests to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addHostRule(final @org.jetbrains.annotations.NotNull java.lang.String host, final @org.jetbrains.annotations.NotNull java.lang.String path, final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.IngressBackend backend) {
software.amazon.jsii.Kernel.call(this, "addHostRule", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(host, "host is required"), java.util.Objects.requireNonNull(path, "path is required"), java.util.Objects.requireNonNull(backend, "backend is required") });
}
/**
* Adds an ingress rule applied to requests sent to a specific HTTP path.
*
* @param path The HTTP path. This parameter is required.
* @param backend The backend to route requests to. This parameter is required.
* @param pathType How the path is matched against request paths.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addRule(final @org.jetbrains.annotations.NotNull java.lang.String path, final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.IngressBackend backend, final @org.jetbrains.annotations.Nullable org.cdk8s.plus22.HttpIngressPathType pathType) {
software.amazon.jsii.Kernel.call(this, "addRule", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(path, "path is required"), java.util.Objects.requireNonNull(backend, "backend is required"), pathType });
}
/**
* Adds an ingress rule applied to requests sent to a specific HTTP path.
*
* @param path The HTTP path. This parameter is required.
* @param backend The backend to route requests to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addRule(final @org.jetbrains.annotations.NotNull java.lang.String path, final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.IngressBackend backend) {
software.amazon.jsii.Kernel.call(this, "addRule", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(path, "path is required"), java.util.Objects.requireNonNull(backend, "backend is required") });
}
/**
* Adds rules to this ingress.
*
* @param rules The rules to add. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addRules(final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.IngressRule... rules) {
software.amazon.jsii.Kernel.call(this, "addRules", software.amazon.jsii.NativeType.VOID, java.util.Arrays.