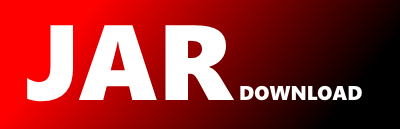
org.cdk8s.plus22.Job Maven / Gradle / Ivy
Show all versions of cdk8s-plus-22 Show documentation
package org.cdk8s.plus22;
/**
* A Job creates one or more Pods and ensures that a specified number of them successfully terminate.
*
* As pods successfully complete,
* the Job tracks the successful completions. When a specified number of successful completions is reached, the task (ie, Job) is complete.
* Deleting a Job will clean up the Pods it created. A simple case is to create one Job object in order to reliably run one Pod to completion.
* The Job object will start a new Pod if the first Pod fails or is deleted (for example due to a node hardware failure or a node reboot).
* You can also use a Job to run multiple Pods in parallel.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.47.0 (build 86d2c33)", date = "2021-12-08T00:16:55.104Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.cdk8s.plus22.$Module.class, fqn = "cdk8s-plus-22.Job")
public class Job extends org.cdk8s.plus22.Resource implements org.cdk8s.plus22.IPodTemplate {
protected Job(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Job(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Job(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable org.cdk8s.plus22.JobProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Job(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* Add a container to the pod.
*
* @param container This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public @org.jetbrains.annotations.NotNull org.cdk8s.plus22.Container addContainer(final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.ContainerProps container) {
return software.amazon.jsii.Kernel.call(this, "addContainer", software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.Container.class), new Object[] { java.util.Objects.requireNonNull(container, "container is required") });
}
/**
* Add a volume to the pod.
*
* @param volume This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void addVolume(final @org.jetbrains.annotations.NotNull org.cdk8s.plus22.Volume volume) {
software.amazon.jsii.Kernel.call(this, "addVolume", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(volume, "volume is required") });
}
/**
* The underlying cdk8s API object.
*
* @see base.Resource.apiObject
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull org.cdk8s.ApiObject getApiObject() {
return software.amazon.jsii.Kernel.get(this, "apiObject", software.amazon.jsii.NativeType.forClass(org.cdk8s.ApiObject.class));
}
/**
* The containers belonging to the pod.
*
* Use addContainer
to add containers.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getContainers() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "containers", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.Container.class))));
}
/**
* Provides read/write access to the underlying pod metadata of the resource.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull org.cdk8s.ApiObjectMetadataDefinition getPodMetadata() {
return software.amazon.jsii.Kernel.get(this, "podMetadata", software.amazon.jsii.NativeType.forClass(org.cdk8s.ApiObjectMetadataDefinition.class));
}
/**
* The volumes associated with this pod.
*
* Use addVolume
to add volumes.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getVolumes() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "volumes", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.Volume.class))));
}
/**
* Duration before job is terminated.
*
* If undefined, there is no deadline.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable org.cdk8s.Duration getActiveDeadline() {
return software.amazon.jsii.Kernel.get(this, "activeDeadline", software.amazon.jsii.NativeType.forClass(org.cdk8s.Duration.class));
}
/**
* Number of retries before marking failed.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Number getBackoffLimit() {
return software.amazon.jsii.Kernel.get(this, "backoffLimit", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Restart policy for all containers within the pod.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable org.cdk8s.plus22.RestartPolicy getRestartPolicy() {
return software.amazon.jsii.Kernel.get(this, "restartPolicy", software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.RestartPolicy.class));
}
/**
* The service account used to run this pod.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable org.cdk8s.plus22.IServiceAccount getServiceAccount() {
return software.amazon.jsii.Kernel.get(this, "serviceAccount", software.amazon.jsii.NativeType.forClass(org.cdk8s.plus22.IServiceAccount.class));
}
/**
* TTL before the job is deleted after it is finished.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable org.cdk8s.Duration getTtlAfterFinished() {
return software.amazon.jsii.Kernel.get(this, "ttlAfterFinished", software.amazon.jsii.NativeType.forClass(org.cdk8s.Duration.class));
}
/**
* A fluent builder for {@link org.cdk8s.plus22.Job}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private org.cdk8s.plus22.JobProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
}
/**
* Metadata that all persisted resources must have, which includes all objects users must create.
*
* @return {@code this}
* @param metadata Metadata that all persisted resources must have, which includes all objects users must create. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metadata(final org.cdk8s.ApiObjectMetadata metadata) {
this.props().metadata(metadata);
return this;
}
/**
* List of containers belonging to the pod.
*
* Containers cannot currently be
* added or removed. There must be at least one container in a Pod.
*
* You can add additionnal containers using podSpec.addContainer()
*
* Default: - No containers. Note that a pod spec must include at least one container.
*
* @return {@code this}
* @param containers List of containers belonging to the pod. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder containers(final java.util.List extends org.cdk8s.plus22.ContainerProps> containers) {
this.props().containers(containers);
return this;
}
/**
* Restart policy for all containers within the pod.
*
* Default: RestartPolicy.ALWAYS
*
* @return {@code this}
* @see https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle/#restart-policy
* @param restartPolicy Restart policy for all containers within the pod. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder restartPolicy(final org.cdk8s.plus22.RestartPolicy restartPolicy) {
this.props().restartPolicy(restartPolicy);
return this;
}
/**
* A service account provides an identity for processes that run in a Pod.
*
* When you (a human) access the cluster (for example, using kubectl), you are
* authenticated by the apiserver as a particular User Account (currently this
* is usually admin, unless your cluster administrator has customized your
* cluster). Processes in containers inside pods can also contact the
* apiserver. When they do, they are authenticated as a particular Service
* Account (for example, default).
*
* Default: - No service account.
*
* @return {@code this}
* @see https://kubernetes.io/docs/tasks/configure-pod-container/configure-service-account/
* @param serviceAccount A service account provides an identity for processes that run in a Pod. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceAccount(final org.cdk8s.plus22.IServiceAccount serviceAccount) {
this.props().serviceAccount(serviceAccount);
return this;
}
/**
* List of volumes that can be mounted by containers belonging to the pod.
*
* You can also add volumes later using podSpec.addVolume()
*
* Default: - No volumes.
*
* @return {@code this}
* @see https://kubernetes.io/docs/concepts/storage/volumes
* @param volumes List of volumes that can be mounted by containers belonging to the pod. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder volumes(final java.util.List extends org.cdk8s.plus22.Volume> volumes) {
this.props().volumes(volumes);
return this;
}
/**
* The pod metadata.
*
* @return {@code this}
* @param podMetadata The pod metadata. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder podMetadata(final org.cdk8s.ApiObjectMetadata podMetadata) {
this.props().podMetadata(podMetadata);
return this;
}
/**
* Specifies the duration the job may be active before the system tries to terminate it.
*
* Default: - If unset, then there is no deadline.
*
* @return {@code this}
* @param activeDeadline Specifies the duration the job may be active before the system tries to terminate it. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder activeDeadline(final org.cdk8s.Duration activeDeadline) {
this.props().activeDeadline(activeDeadline);
return this;
}
/**
* Specifies the number of retries before marking this job failed.
*
* Default: - If not set, system defaults to 6.
*
* @return {@code this}
* @param backoffLimit Specifies the number of retries before marking this job failed. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder backoffLimit(final java.lang.Number backoffLimit) {
this.props().backoffLimit(backoffLimit);
return this;
}
/**
* Limits the lifetime of a Job that has finished execution (either Complete or Failed).
*
* If this field is set, after the Job finishes, it is eligible to
* be automatically deleted. When the Job is being deleted, its lifecycle
* guarantees (e.g. finalizers) will be honored. If this field is set to zero,
* the Job becomes eligible to be deleted immediately after it finishes. This
* field is alpha-level and is only honored by servers that enable the
* TTLAfterFinished
feature.
*
* Default: - If this field is unset, the Job won't be automatically deleted.
*
* @return {@code this}
* @param ttlAfterFinished Limits the lifetime of a Job that has finished execution (either Complete or Failed). This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ttlAfterFinished(final org.cdk8s.Duration ttlAfterFinished) {
this.props().ttlAfterFinished(ttlAfterFinished);
return this;
}
/**
* @returns a newly built instance of {@link org.cdk8s.plus22.Job}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public org.cdk8s.plus22.Job build() {
return new org.cdk8s.plus22.Job(
this.scope,
this.id,
this.props != null ? this.props.build() : null
);
}
private org.cdk8s.plus22.JobProps.Builder props() {
if (this.props == null) {
this.props = new org.cdk8s.plus22.JobProps.Builder();
}
return this.props;
}
}
}