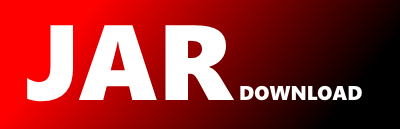
org.cdk8s.Duration Maven / Gradle / Ivy
Show all versions of cdk8s Show documentation
package org.cdk8s;
/**
* Represents a length of time.
*
* The amount can be specified either as a literal value (e.g: 10
) which
* cannot be negative.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.88.0 (build eaabd08)", date = "2023-09-11T12:14:52.871Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.cdk8s.$Module.class, fqn = "cdk8s.Duration")
public class Duration extends software.amazon.jsii.JsiiObject {
protected Duration(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Duration(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Create a Duration representing an amount of days.
*
* @return a new Duration
representing amount
Days.
* @param amount the amount of Days the Duration
will represent. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Duration days(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Duration.class, "days", software.amazon.jsii.NativeType.forClass(org.cdk8s.Duration.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Create a Duration representing an amount of hours.
*
* @return a new Duration
representing amount
Hours.
* @param amount the amount of Hours the Duration
will represent. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Duration hours(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Duration.class, "hours", software.amazon.jsii.NativeType.forClass(org.cdk8s.Duration.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Create a Duration representing an amount of milliseconds.
*
* @return a new Duration
representing amount
ms.
* @param amount the amount of Milliseconds the Duration
will represent. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Duration millis(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Duration.class, "millis", software.amazon.jsii.NativeType.forClass(org.cdk8s.Duration.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Create a Duration representing an amount of minutes.
*
* @return a new Duration
representing amount
Minutes.
* @param amount the amount of Minutes the Duration
will represent. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Duration minutes(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Duration.class, "minutes", software.amazon.jsii.NativeType.forClass(org.cdk8s.Duration.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Parse a period formatted according to the ISO 8601 standard.
*
* @return the parsed Duration
.
* @see https://www.iso.org/fr/standard/70907.html
* @param duration an ISO-formtted duration to be parsed. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Duration parse(final @org.jetbrains.annotations.NotNull java.lang.String duration) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Duration.class, "parse", software.amazon.jsii.NativeType.forClass(org.cdk8s.Duration.class), new Object[] { java.util.Objects.requireNonNull(duration, "duration is required") });
}
/**
* Create a Duration representing an amount of seconds.
*
* @return a new Duration
representing amount
Seconds.
* @param amount the amount of Seconds the Duration
will represent. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Duration seconds(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Duration.class, "seconds", software.amazon.jsii.NativeType.forClass(org.cdk8s.Duration.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Return the total number of days in this Duration.
*
* @return the value of this Duration
expressed in Days.
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toDays(final @org.jetbrains.annotations.Nullable org.cdk8s.TimeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toDays", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return the total number of days in this Duration.
*
* @return the value of this Duration
expressed in Days.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toDays() {
return software.amazon.jsii.Kernel.call(this, "toDays", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Return the total number of hours in this Duration.
*
* @return the value of this Duration
expressed in Hours.
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toHours(final @org.jetbrains.annotations.Nullable org.cdk8s.TimeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toHours", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return the total number of hours in this Duration.
*
* @return the value of this Duration
expressed in Hours.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toHours() {
return software.amazon.jsii.Kernel.call(this, "toHours", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Turn this duration into a human-readable string.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String toHumanString() {
return software.amazon.jsii.Kernel.call(this, "toHumanString", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Return an ISO 8601 representation of this period.
*
* @return a string starting with 'PT' describing the period
* @see https://www.iso.org/fr/standard/70907.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String toIsoString() {
return software.amazon.jsii.Kernel.call(this, "toIsoString", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Return the total number of milliseconds in this Duration.
*
* @return the value of this Duration
expressed in Milliseconds.
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toMilliseconds(final @org.jetbrains.annotations.Nullable org.cdk8s.TimeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toMilliseconds", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return the total number of milliseconds in this Duration.
*
* @return the value of this Duration
expressed in Milliseconds.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toMilliseconds() {
return software.amazon.jsii.Kernel.call(this, "toMilliseconds", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Return the total number of minutes in this Duration.
*
* @return the value of this Duration
expressed in Minutes.
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toMinutes(final @org.jetbrains.annotations.Nullable org.cdk8s.TimeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toMinutes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return the total number of minutes in this Duration.
*
* @return the value of this Duration
expressed in Minutes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toMinutes() {
return software.amazon.jsii.Kernel.call(this, "toMinutes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Return the total number of seconds in this Duration.
*
* @return the value of this Duration
expressed in Seconds.
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toSeconds(final @org.jetbrains.annotations.Nullable org.cdk8s.TimeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toSeconds", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return the total number of seconds in this Duration.
*
* @return the value of this Duration
expressed in Seconds.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toSeconds() {
return software.amazon.jsii.Kernel.call(this, "toSeconds", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Return unit of Duration.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String unitLabel() {
return software.amazon.jsii.Kernel.call(this, "unitLabel", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
}