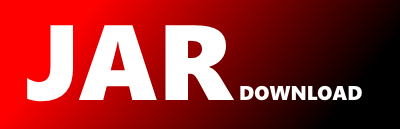
org.cdk8s.Size Maven / Gradle / Ivy
Show all versions of cdk8s Show documentation
package org.cdk8s;
/**
* Represents the amount of digital storage.
*
* The amount can be specified either as a literal value (e.g: 10
) which
* cannot be negative.
*
* When the amount is passed as a token, unit conversion is not possible.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.92.0 (build db7f27d)", date = "2023-11-17T12:32:48.504Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.cdk8s.$Module.class, fqn = "cdk8s.Size")
public class Size extends software.amazon.jsii.JsiiObject {
protected Size(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected Size(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* Create a Storage representing an amount gibibytes.
*
* 1 GiB = 1024 MiB
*
* @param amount This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Size gibibytes(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Size.class, "gibibytes", software.amazon.jsii.NativeType.forClass(org.cdk8s.Size.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Create a Storage representing an amount kibibytes.
*
* 1 KiB = 1024 bytes
*
* @param amount This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Size kibibytes(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Size.class, "kibibytes", software.amazon.jsii.NativeType.forClass(org.cdk8s.Size.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Create a Storage representing an amount mebibytes.
*
* 1 MiB = 1024 KiB
*
* @param amount This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Size mebibytes(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Size.class, "mebibytes", software.amazon.jsii.NativeType.forClass(org.cdk8s.Size.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Create a Storage representing an amount pebibytes.
*
* 1 PiB = 1024 TiB
*
* @param amount This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Size pebibyte(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Size.class, "pebibyte", software.amazon.jsii.NativeType.forClass(org.cdk8s.Size.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Create a Storage representing an amount tebibytes.
*
* 1 TiB = 1024 GiB
*
* @param amount This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull org.cdk8s.Size tebibytes(final @org.jetbrains.annotations.NotNull java.lang.Number amount) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(org.cdk8s.Size.class, "tebibytes", software.amazon.jsii.NativeType.forClass(org.cdk8s.Size.class), new Object[] { java.util.Objects.requireNonNull(amount, "amount is required") });
}
/**
* Return this storage as a total number of gibibytes.
*
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toGibibytes(final @org.jetbrains.annotations.Nullable org.cdk8s.SizeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toGibibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return this storage as a total number of gibibytes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toGibibytes() {
return software.amazon.jsii.Kernel.call(this, "toGibibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Return this storage as a total number of kibibytes.
*
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toKibibytes(final @org.jetbrains.annotations.Nullable org.cdk8s.SizeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toKibibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return this storage as a total number of kibibytes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toKibibytes() {
return software.amazon.jsii.Kernel.call(this, "toKibibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Return this storage as a total number of mebibytes.
*
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toMebibytes(final @org.jetbrains.annotations.Nullable org.cdk8s.SizeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toMebibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return this storage as a total number of mebibytes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toMebibytes() {
return software.amazon.jsii.Kernel.call(this, "toMebibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Return this storage as a total number of pebibytes.
*
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toPebibytes(final @org.jetbrains.annotations.Nullable org.cdk8s.SizeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toPebibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return this storage as a total number of pebibytes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toPebibytes() {
return software.amazon.jsii.Kernel.call(this, "toPebibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
/**
* Return this storage as a total number of tebibytes.
*
* @param opts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toTebibytes(final @org.jetbrains.annotations.Nullable org.cdk8s.SizeConversionOptions opts) {
return software.amazon.jsii.Kernel.call(this, "toTebibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class), new Object[] { opts });
}
/**
* Return this storage as a total number of tebibytes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Number toTebibytes() {
return software.amazon.jsii.Kernel.call(this, "toTebibytes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
}
}