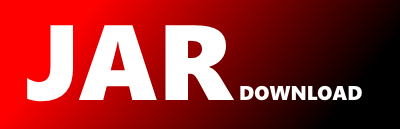
org.cdk8s.ApiObjectMetadata Maven / Gradle / Ivy
Show all versions of cdk8s Show documentation
package org.cdk8s;
/**
* Metadata associated with this object.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.95.0 (build f1ff514)", date = "2024-03-07T12:12:05.993Z")
@software.amazon.jsii.Jsii(module = org.cdk8s.$Module.class, fqn = "cdk8s.ApiObjectMetadata")
@software.amazon.jsii.Jsii.Proxy(ApiObjectMetadata.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface ApiObjectMetadata extends software.amazon.jsii.JsiiSerializable {
/**
* Annotations is an unstructured key value map stored with a resource that may be set by external tools to store and retrieve arbitrary metadata.
*
* They are not queryable and should be
* preserved when modifying objects.
*
* Default: - No annotations.
*
* @see http://kubernetes.io/docs/user-guide/annotations
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getAnnotations() {
return null;
}
/**
* Namespaced keys that tell Kubernetes to wait until specific conditions are met before it fully deletes resources marked for deletion.
*
* Must be empty before the object is deleted from the registry. Each entry is
* an identifier for the responsible component that will remove the entry from
* the list. If the deletionTimestamp of the object is non-nil, entries in
* this list can only be removed. Finalizers may be processed and removed in
* any order. Order is NOT enforced because it introduces significant risk of
* stuck finalizers. finalizers is a shared field, any actor with permission
* can reorder it. If the finalizer list is processed in order, then this can
* lead to a situation in which the component responsible for the first
* finalizer in the list is waiting for a signal (field value, external
* system, or other) produced by a component responsible for a finalizer later
* in the list, resulting in a deadlock. Without enforced ordering finalizers
* are free to order amongst themselves and are not vulnerable to ordering
* changes in the list.
*
* Default: - No finalizers.
*
* @see https://kubernetes.io/docs/concepts/overview/working-with-objects/finalizers/
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getFinalizers() {
return null;
}
/**
* Map of string keys and values that can be used to organize and categorize (scope and select) objects.
*
* May match selectors of replication controllers and services.
*
* Default: - No labels.
*
* @see http://kubernetes.io/docs/user-guide/labels
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getLabels() {
return null;
}
/**
* The unique, namespace-global, name of this object inside the Kubernetes cluster.
*
* Normally, you shouldn't specify names for objects and let the CDK generate
* a name for you that is application-unique. The names CDK generates are
* composed from the construct path components, separated by dots and a suffix
* that is based on a hash of the entire path, to ensure uniqueness.
*
* You can supply custom name allocation logic by overriding the
* chart.generateObjectName
method.
*
* If you use an explicit name here, bear in mind that this reduces the
* composability of your construct because it won't be possible to include
* more than one instance in any app. Therefore it is highly recommended to
* leave this unspecified.
*
* Default: - an app-unique name generated by the chart
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getName() {
return null;
}
/**
* Namespace defines the space within each name must be unique.
*
* An empty namespace is equivalent to the "default" namespace, but "default" is the canonical representation.
* Not all objects are required to be scoped to a namespace - the value of this field for those objects will be empty. Must be a DNS_LABEL. Cannot be updated. More info: http://kubernetes.io/docs/user-guide/namespaces
*
* Default: undefined (will be assigned to the 'default' namespace)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getNamespace() {
return null;
}
/**
* List of objects depended by this object.
*
* If ALL objects in the list have
* been deleted, this object will be garbage collected. If this object is
* managed by a controller, then an entry in this list will point to this
* controller, with the controller field set to true. There cannot be more
* than one managing controller.
*
* Kubernetes sets the value of this field automatically for objects that are
* dependents of other objects like ReplicaSets, DaemonSets, Deployments, Jobs
* and CronJobs, and ReplicationControllers. You can also configure these
* relationships manually by changing the value of this field. However, you
* usually don't need to and can allow Kubernetes to automatically manage the
* relationships.
*
* Default: - automatically set by Kubernetes
*
* @see https://kubernetes.io/docs/concepts/overview/working-with-objects/owners-dependents/
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getOwnerReferences() {
return null;
}
/**
* @return a {@link Builder} of {@link ApiObjectMetadata}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ApiObjectMetadata}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.util.Map annotations;
java.util.List finalizers;
java.util.Map labels;
java.lang.String name;
java.lang.String namespace;
java.util.List ownerReferences;
/**
* Sets the value of {@link ApiObjectMetadata#getAnnotations}
* @param annotations Annotations is an unstructured key value map stored with a resource that may be set by external tools to store and retrieve arbitrary metadata.
* They are not queryable and should be
* preserved when modifying objects.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder annotations(java.util.Map annotations) {
this.annotations = annotations;
return this;
}
/**
* Sets the value of {@link ApiObjectMetadata#getFinalizers}
* @param finalizers Namespaced keys that tell Kubernetes to wait until specific conditions are met before it fully deletes resources marked for deletion.
* Must be empty before the object is deleted from the registry. Each entry is
* an identifier for the responsible component that will remove the entry from
* the list. If the deletionTimestamp of the object is non-nil, entries in
* this list can only be removed. Finalizers may be processed and removed in
* any order. Order is NOT enforced because it introduces significant risk of
* stuck finalizers. finalizers is a shared field, any actor with permission
* can reorder it. If the finalizer list is processed in order, then this can
* lead to a situation in which the component responsible for the first
* finalizer in the list is waiting for a signal (field value, external
* system, or other) produced by a component responsible for a finalizer later
* in the list, resulting in a deadlock. Without enforced ordering finalizers
* are free to order amongst themselves and are not vulnerable to ordering
* changes in the list.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder finalizers(java.util.List finalizers) {
this.finalizers = finalizers;
return this;
}
/**
* Sets the value of {@link ApiObjectMetadata#getLabels}
* @param labels Map of string keys and values that can be used to organize and categorize (scope and select) objects.
* May match selectors of replication controllers and services.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder labels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Sets the value of {@link ApiObjectMetadata#getName}
* @param name The unique, namespace-global, name of this object inside the Kubernetes cluster.
* Normally, you shouldn't specify names for objects and let the CDK generate
* a name for you that is application-unique. The names CDK generates are
* composed from the construct path components, separated by dots and a suffix
* that is based on a hash of the entire path, to ensure uniqueness.
*
* You can supply custom name allocation logic by overriding the
* chart.generateObjectName
method.
*
* If you use an explicit name here, bear in mind that this reduces the
* composability of your construct because it won't be possible to include
* more than one instance in any app. Therefore it is highly recommended to
* leave this unspecified.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link ApiObjectMetadata#getNamespace}
* @param namespace Namespace defines the space within each name must be unique.
* An empty namespace is equivalent to the "default" namespace, but "default" is the canonical representation.
* Not all objects are required to be scoped to a namespace - the value of this field for those objects will be empty. Must be a DNS_LABEL. Cannot be updated. More info: http://kubernetes.io/docs/user-guide/namespaces
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder namespace(java.lang.String namespace) {
this.namespace = namespace;
return this;
}
/**
* Sets the value of {@link ApiObjectMetadata#getOwnerReferences}
* @param ownerReferences List of objects depended by this object.
* If ALL objects in the list have
* been deleted, this object will be garbage collected. If this object is
* managed by a controller, then an entry in this list will point to this
* controller, with the controller field set to true. There cannot be more
* than one managing controller.
*
* Kubernetes sets the value of this field automatically for objects that are
* dependents of other objects like ReplicaSets, DaemonSets, Deployments, Jobs
* and CronJobs, and ReplicationControllers. You can also configure these
* relationships manually by changing the value of this field. However, you
* usually don't need to and can allow Kubernetes to automatically manage the
* relationships.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder ownerReferences(java.util.List extends org.cdk8s.OwnerReference> ownerReferences) {
this.ownerReferences = (java.util.List)ownerReferences;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ApiObjectMetadata}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ApiObjectMetadata build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ApiObjectMetadata}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ApiObjectMetadata {
private final java.util.Map annotations;
private final java.util.List finalizers;
private final java.util.Map labels;
private final java.lang.String name;
private final java.lang.String namespace;
private final java.util.List ownerReferences;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.annotations = software.amazon.jsii.Kernel.get(this, "annotations", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.finalizers = software.amazon.jsii.Kernel.get(this, "finalizers", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.labels = software.amazon.jsii.Kernel.get(this, "labels", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.namespace = software.amazon.jsii.Kernel.get(this, "namespace", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.ownerReferences = software.amazon.jsii.Kernel.get(this, "ownerReferences", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.OwnerReference.class)));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.annotations = builder.annotations;
this.finalizers = builder.finalizers;
this.labels = builder.labels;
this.name = builder.name;
this.namespace = builder.namespace;
this.ownerReferences = (java.util.List)builder.ownerReferences;
}
@Override
public final java.util.Map getAnnotations() {
return this.annotations;
}
@Override
public final java.util.List getFinalizers() {
return this.finalizers;
}
@Override
public final java.util.Map getLabels() {
return this.labels;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final java.lang.String getNamespace() {
return this.namespace;
}
@Override
public final java.util.List getOwnerReferences() {
return this.ownerReferences;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAnnotations() != null) {
data.set("annotations", om.valueToTree(this.getAnnotations()));
}
if (this.getFinalizers() != null) {
data.set("finalizers", om.valueToTree(this.getFinalizers()));
}
if (this.getLabels() != null) {
data.set("labels", om.valueToTree(this.getLabels()));
}
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
if (this.getNamespace() != null) {
data.set("namespace", om.valueToTree(this.getNamespace()));
}
if (this.getOwnerReferences() != null) {
data.set("ownerReferences", om.valueToTree(this.getOwnerReferences()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("cdk8s.ApiObjectMetadata"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ApiObjectMetadata.Jsii$Proxy that = (ApiObjectMetadata.Jsii$Proxy) o;
if (this.annotations != null ? !this.annotations.equals(that.annotations) : that.annotations != null) return false;
if (this.finalizers != null ? !this.finalizers.equals(that.finalizers) : that.finalizers != null) return false;
if (this.labels != null ? !this.labels.equals(that.labels) : that.labels != null) return false;
if (this.name != null ? !this.name.equals(that.name) : that.name != null) return false;
if (this.namespace != null ? !this.namespace.equals(that.namespace) : that.namespace != null) return false;
return this.ownerReferences != null ? this.ownerReferences.equals(that.ownerReferences) : that.ownerReferences == null;
}
@Override
public final int hashCode() {
int result = this.annotations != null ? this.annotations.hashCode() : 0;
result = 31 * result + (this.finalizers != null ? this.finalizers.hashCode() : 0);
result = 31 * result + (this.labels != null ? this.labels.hashCode() : 0);
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
result = 31 * result + (this.namespace != null ? this.namespace.hashCode() : 0);
result = 31 * result + (this.ownerReferences != null ? this.ownerReferences.hashCode() : 0);
return result;
}
}
}