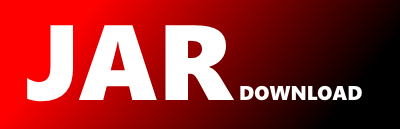
org.cdk8s.DependencyVertex Maven / Gradle / Ivy
Show all versions of cdk8s Show documentation
package org.cdk8s;
/**
* Represents a vertex in the graph.
*
* The value of each vertex is an IConstruct
that is accessible via the .value
getter.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.105.0 (build 0a2adcb)", date = "2024-11-20T06:18:11.265Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = org.cdk8s.$Module.class, fqn = "cdk8s.DependencyVertex")
public class DependencyVertex extends software.amazon.jsii.JsiiObject {
protected DependencyVertex(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected DependencyVertex(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param value
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public DependencyVertex(final @org.jetbrains.annotations.Nullable software.constructs.IConstruct value) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { value });
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public DependencyVertex() {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this);
}
/**
* Adds a vertex as a dependency of the current node.
*
* Also updates the parents of dep
, so that it contains this node as a parent.
*
* This operation will fail in case it creates a cycle in the graph.
*
* @param dep The dependency. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void addChild(final @org.jetbrains.annotations.NotNull org.cdk8s.DependencyVertex dep) {
software.amazon.jsii.Kernel.call(this, "addChild", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(dep, "dep is required") });
}
/**
* Returns a topologically sorted array of the constructs in the sub-graph.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List topology() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.call(this, "topology", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.constructs.IConstruct.class))));
}
/**
* Returns the parents of the vertex (i.e dependants).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getInbound() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "inbound", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.DependencyVertex.class))));
}
/**
* Returns the children of the vertex (i.e dependencies).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.util.List getOutbound() {
return java.util.Collections.unmodifiableList(software.amazon.jsii.Kernel.get(this, "outbound", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(org.cdk8s.DependencyVertex.class))));
}
/**
* Returns the IConstruct this graph vertex represents.
*
* null
in case this is the root of the graph.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable software.constructs.IConstruct getValue() {
return software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(software.constructs.IConstruct.class));
}
}