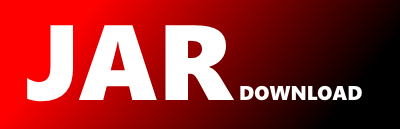
ceylon.modules.jboss.runtime.Graph Maven / Gradle / Ivy
/*
* Copyright 2011 Red Hat inc. and third party contributors as noted
* by the author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ceylon.modules.jboss.runtime;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* Simple graph impl.
*
* @param exact key type
* @param exact vertex value type
* @param exact edge cost type
* @author Ales Justin
*/
class Graph {
private ConcurrentMap> vertices = new ConcurrentHashMap>();
public Vertex createVertex(K key, V value) {
Vertex v = new Vertex(value);
Vertex previous = vertices.putIfAbsent(key, v);
return (previous != null) ? previous : v;
}
public Vertex getVertex(K key) {
return vertices.get(key);
}
public Collection> getVertices() {
return vertices != null ? Collections.unmodifiableCollection(vertices.values()) : Collections.>emptySet();
}
public static class Vertex {
private V value;
private Set> in;
private Set> out;
Vertex(V value) {
this.value = value;
}
public V getValue() {
return value;
}
private void addIn(Edge edge) {
if (in == null)
in = new HashSet>();
in.add(edge);
}
private void addOut(Edge edge) {
if (out == null)
out = new HashSet>();
out.add(edge);
}
public Set> getIn() {
return in != null ? Collections.unmodifiableSet(in) : Collections.>emptySet();
}
public Set> getOut() {
return out != null ? Collections.unmodifiableSet(out) : Collections.>emptySet();
}
public int hashCode() {
return value.hashCode();
}
@SuppressWarnings({"unchecked"})
public boolean equals(Object obj) {
if (obj instanceof Vertex == false)
return false;
Vertex other = (Vertex) obj;
return value.equals(other.value);
}
}
public static class Edge {
private E cost;
private Vertex from;
private Vertex to;
public static void create(E cost, Vertex from, Vertex to) {
new Edge(cost, from, to);
}
private Edge(E cost, Vertex from, Vertex to) {
if (from == null)
throw new IllegalArgumentException("Null from");
if (to == null)
throw new IllegalArgumentException("Null to");
this.cost = cost;
this.from = from;
this.to = to;
from.addOut(this);
to.addIn(this);
}
public E getCost() {
return cost;
}
public Vertex getFrom() {
return from;
}
public Vertex getTo() {
return to;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy