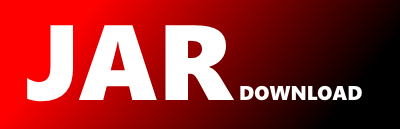
com.redhat.ceylon.compiler.java.language.AbstractArrayIterable Maven / Gradle / Ivy
package com.redhat.ceylon.compiler.java.language;
import ceylon.language.AssertionError;
import ceylon.language.Boolean;
import ceylon.language.Callable;
import ceylon.language.Iterator;
import ceylon.language.Null;
import ceylon.language.emptyIterator_;
import ceylon.language.impl.BaseIterable;
import com.redhat.ceylon.compiler.java.metadata.Ignore;
import com.redhat.ceylon.compiler.java.runtime.model.TypeDescriptor;
/**
* Abstract implementation of {@link ceylon.language.Iterable}
* which iterates over an array (it could be an array of
* primitives, or an array of references, we don't know).
*
* Various methods (such as {@link #take(long)},
* {@link #skip(long)} and {@link #by(long)} are overridden
* so that they iterate over the same array instance.
*
* @param The element type
* @param The array type
*/
public abstract class AbstractArrayIterable
extends BaseIterable {
private final TypeDescriptor $reified$Element;
/** The array (could be an array or primitives or references) */
private final ArrayType array;
/** The index where iteration starts */
protected final int start;
/** The step size of iteration */
protected final int step;
/** The number of elements in the iteration */
protected final int len;
@Override
public TypeDescriptor $getType$() {
return TypeDescriptor.klass(AbstractArrayIterable.class,
this.$reified$Element);
}
@Ignore
public AbstractArrayIterable(TypeDescriptor $reified$Element,
ArrayType array, int length) {
this($reified$Element, array, 0, length, 1);
}
/**
* Constructor used internally via
* {@link #newInstance(Object, int, int, int)}
*/
@Ignore
protected AbstractArrayIterable(TypeDescriptor $reified$Element,
ArrayType array, int start, int len, int step) {
super($reified$Element, Null.$TypeDescriptor$);
if (start < 0) {
throw new AssertionError("start must be non-negative");
}
if (len < 0) {
throw new AssertionError("len must be non-negative");
}
if (step <= 0) {
throw new AssertionError("step size must be greater than zero");
}
this.array = array;
this.$reified$Element = $reified$Element;
this.start = start;
this.len = len;
this.step = step;
}
/** Factory for creating a new instance */
protected abstract AbstractArrayIterable
newInstance(ArrayType array, int start, int len, int step);
protected abstract Element get(ArrayType array, int index);
@Ignore
public ArrayType arrayValue() {
return array;
}
@Override
public boolean getEmpty() {
return this.len == 0;
}
@Override
public Element getFirst() {
return this.getEmpty() ?
null :
get(this.array, this.start);
}
@Override
public Element getLast() {
return this.getEmpty() ?
null :
get(this.array, this.start+this.step*this.len-1);
}
@Override
public AbstractArrayIterable getRest() {
return getEmpty() ?
this :
newInstance(this.array,
this.start+this.step,
this.len-1,
this.step);
}
@Ignore
@Override
public long getSize() {
return Math.max(0, this.len);
}
@SuppressWarnings("unchecked")
@Override
public Iterator extends Element> iterator() {
if (this.getEmpty()) {
return (Iterator extends Element>)
emptyIterator_.get_();
}
return new AbstractArrayIterator($reified$Element,
start, len, step) {
protected Element get(int index) {
return AbstractArrayIterable.this.get(array, index);
}
};
}
@Override
public boolean longerThan(long length) {
return this.getSize() > length;
}
@Override
public boolean shorterThan(long length) {
return this.getSize() < length;
}
@Override
public AbstractArrayIterable
skip(long skip) {
if (skip <= 0) {
return this;
}
return newInstance(this.array,
this.start+(int)skip*this.step,
this.len-(int)skip,
this.step);
}
@Override
public AbstractArrayIterable
take(long take) {
if (take >= this.getSize()) {
return this;
}
return newInstance(this.array,
this.start,
(int)take,
this.step);
}
@Override
public AbstractArrayIterable
by(long step) {
return newInstance(this.array,
this.start,
(int)((this.len+step-1)/step), //ceiling division
this.step*(int)step);
}
@Override
public java.lang.Object each(
Callable extends java.lang.Object> step) {
int end = start+len;
for (int i=start; i selecting) {
// FIXME Very inefficient for primitive types due to boxing
int count=0;
int end = start+len;
for (int i=start; i selecting) {
int end = start+len;
for (int i=start; i selecting) {
int end = start+len;
for (int i=start; i selecting) {
int end = start+len;
for (int i=start; i selecting) {
int end = start+len;
for (int i=start; i java.lang.Object
reduce(@Ignore TypeDescriptor $reifiedResult,
Callable extends Result> accumulating) {
if (len==0) {
return null;
}
java.lang.Object partial = get(this.array,start);
int end = start+len;
for (int i=start+1; i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy