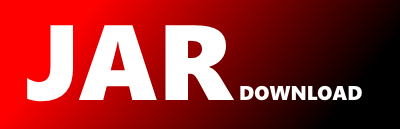
com.redhat.ceylon.langtools.tools.javac.tree.TreeMaker Maven / Gradle / Ivy
/*
* Copyright (c) 1999, 2011, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
* or visit www.oracle.com if you need additional information or have any
* questions.
*/
package com.redhat.ceylon.langtools.tools.javac.tree;
import static com.redhat.ceylon.langtools.tools.javac.code.Flags.*;
import static com.redhat.ceylon.langtools.tools.javac.code.Kinds.*;
import static com.redhat.ceylon.langtools.tools.javac.code.TypeTags.*;
import com.redhat.ceylon.langtools.tools.javac.code.*;
import com.redhat.ceylon.langtools.tools.javac.code.Symbol.*;
import com.redhat.ceylon.langtools.tools.javac.code.Type.*;
import com.redhat.ceylon.langtools.tools.javac.tree.JCTree.*;
import com.redhat.ceylon.langtools.tools.javac.util.*;
import com.redhat.ceylon.langtools.tools.javac.util.JCDiagnostic.DiagnosticPosition;
/** Factory class for trees.
*
* This is NOT part of any supported API.
* If you write code that depends on this, you do so at your own risk.
* This code and its internal interfaces are subject to change or
* deletion without notice.
*/
public class TreeMaker implements JCTree.Factory {
/** The context key for the tree factory. */
protected static final Context.Key treeMakerKey =
new Context.Key();
/** Get the TreeMaker instance. */
public static TreeMaker instance(Context context) {
TreeMaker instance = context.get(treeMakerKey);
if (instance == null)
instance = new TreeMaker(context);
return instance;
}
/** The position at which subsequent trees will be created.
*/
public int pos = Position.NOPOS;
/** The toplevel tree to which created trees belong.
*/
public JCCompilationUnit toplevel;
/** The current name table. */
Names names;
Types types;
/** The current symbol table. */
Symtab syms;
/** Create a tree maker with null toplevel and NOPOS as initial position.
*/
protected TreeMaker(Context context) {
context.put(treeMakerKey, this);
this.pos = Position.NOPOS;
this.toplevel = null;
this.names = Names.instance(context);
this.syms = Symtab.instance(context);
this.types = Types.instance(context);
}
/** Create a tree maker with a given toplevel and FIRSTPOS as initial position.
*/
TreeMaker(JCCompilationUnit toplevel, Names names, Types types, Symtab syms) {
this.pos = Position.FIRSTPOS;
this.toplevel = toplevel;
this.names = names;
this.types = types;
this.syms = syms;
}
/** Create a new tree maker for a given toplevel.
*/
public TreeMaker forToplevel(JCCompilationUnit toplevel) {
return new TreeMaker(toplevel, names, types, syms);
}
/** Reassign current position.
*/
public TreeMaker at(int pos) {
this.pos = pos;
return this;
}
/** Reassign current position.
*/
public TreeMaker at(DiagnosticPosition pos) {
this.pos = (pos == null ? Position.NOPOS : pos.getStartPosition());
return this;
}
/**
* Create given tree node at current position.
* @param defs a list of ClassDef, Import, and Skip
*/
public JCCompilationUnit TopLevel(List packageAnnotations,
JCExpression pid,
List defs) {
Assert.checkNonNull(packageAnnotations);
for (JCTree node : defs)
Assert.check(node instanceof JCClassDecl
|| node instanceof JCImport
|| node instanceof JCSkip
|| node instanceof JCErroneous
|| (node instanceof JCExpressionStatement
&& ((JCExpressionStatement)node).expr instanceof JCErroneous),
node.getClass().getSimpleName());
JCCompilationUnit tree = new JCCompilationUnit(packageAnnotations, pid, defs,
null, null, null, null);
tree.pos = pos;
return tree;
}
public JCImport Import(JCTree qualid, boolean importStatic) {
JCImport tree = new JCImport(qualid, importStatic);
tree.pos = pos;
return tree;
}
public JCClassDecl ClassDef(JCModifiers mods,
Name name,
List typarams,
JCExpression extending,
List implementing,
List defs)
{
JCClassDecl tree = new JCClassDecl(mods,
name,
typarams,
extending,
implementing,
defs,
null);
tree.pos = pos;
return tree;
}
public JCMethodDecl MethodDef(JCModifiers mods,
Name name,
JCExpression restype,
List typarams,
List params,
List thrown,
JCBlock body,
JCExpression defaultValue) {
JCMethodDecl tree = new JCMethodDecl(mods,
name,
restype,
typarams,
params,
thrown,
body,
defaultValue,
null);
tree.pos = pos;
return tree;
}
public JCVariableDecl VarDef(JCModifiers mods, Name name, JCExpression vartype, JCExpression init) {
JCVariableDecl tree = new JCVariableDecl(mods, name, vartype, init, null);
tree.pos = pos;
return tree;
}
public JCSkip Skip() {
JCSkip tree = new JCSkip();
tree.pos = pos;
return tree;
}
public JCBlock Block(long flags, List stats) {
JCBlock tree = new JCBlock(flags, stats);
tree.pos = pos;
return tree;
}
public JCDoWhileLoop DoLoop(JCStatement body, JCExpression cond) {
JCDoWhileLoop tree = new JCDoWhileLoop(body, cond);
tree.pos = pos;
return tree;
}
public JCWhileLoop WhileLoop(JCExpression cond, JCStatement body) {
JCWhileLoop tree = new JCWhileLoop(cond, body);
tree.pos = pos;
return tree;
}
public JCForLoop ForLoop(List init,
JCExpression cond,
List step,
JCStatement body)
{
JCForLoop tree = new JCForLoop(init, cond, step, body);
tree.pos = pos;
return tree;
}
public JCEnhancedForLoop ForeachLoop(JCVariableDecl var, JCExpression expr, JCStatement body) {
JCEnhancedForLoop tree = new JCEnhancedForLoop(var, expr, body);
tree.pos = pos;
return tree;
}
public JCLabeledStatement Labelled(Name label, JCStatement body) {
JCLabeledStatement tree = new JCLabeledStatement(label, body);
tree.pos = pos;
return tree;
}
public JCSwitch Switch(JCExpression selector, List cases) {
JCSwitch tree = new JCSwitch(selector, cases);
tree.pos = pos;
return tree;
}
public JCCase Case(JCExpression pat, List stats) {
JCCase tree = new JCCase(pat, stats);
tree.pos = pos;
return tree;
}
public JCSynchronized Synchronized(JCExpression lock, JCBlock body) {
JCSynchronized tree = new JCSynchronized(lock, body);
tree.pos = pos;
return tree;
}
public JCTry Try(JCBlock body, List catchers, JCBlock finalizer) {
return Try(List.nil(), body, catchers, finalizer);
}
public JCTry Try(List resources,
JCBlock body,
List catchers,
JCBlock finalizer) {
JCTry tree = new JCTry(resources, body, catchers, finalizer);
tree.pos = pos;
return tree;
}
public JCCatch Catch(JCVariableDecl param, JCBlock body) {
JCCatch tree = new JCCatch(param, body);
tree.pos = pos;
return tree;
}
public JCConditional Conditional(JCExpression cond,
JCExpression thenpart,
JCExpression elsepart)
{
JCConditional tree = new JCConditional(cond, thenpart, elsepart);
tree.pos = pos;
return tree;
}
public JCIf If(JCExpression cond, JCStatement thenpart, JCStatement elsepart) {
JCIf tree = new JCIf(cond, thenpart, elsepart);
tree.pos = pos;
return tree;
}
public JCExpressionStatement Exec(JCExpression expr) {
JCExpressionStatement tree = new JCExpressionStatement(expr);
tree.pos = pos;
return tree;
}
public JCBreak Break(Name label) {
JCBreak tree = new JCBreak(label, null);
tree.pos = pos;
return tree;
}
public JCContinue Continue(Name label) {
JCContinue tree = new JCContinue(label, null);
tree.pos = pos;
return tree;
}
public JCReturn Return(JCExpression expr) {
JCReturn tree = new JCReturn(expr);
tree.pos = pos;
return tree;
}
public JCThrow Throw(JCTree expr) {
JCThrow tree = new JCThrow(expr);
tree.pos = pos;
return tree;
}
public JCAssert Assert(JCExpression cond, JCExpression detail) {
JCAssert tree = new JCAssert(cond, detail);
tree.pos = pos;
return tree;
}
public JCMethodInvocation Apply(List typeargs,
JCExpression fn,
List args)
{
JCMethodInvocation tree = new JCMethodInvocation(typeargs, fn, args);
tree.pos = pos;
return tree;
}
public JCNewClass NewClass(JCExpression encl,
List typeargs,
JCExpression clazz,
List args,
JCClassDecl def)
{
JCNewClass tree = new JCNewClass(encl, typeargs, clazz, args, def);
tree.pos = pos;
return tree;
}
public JCNewArray NewArray(JCExpression elemtype,
List dims,
List elems)
{
JCNewArray tree = new JCNewArray(elemtype, dims, elems);
tree.pos = pos;
return tree;
}
public JCParens Parens(JCExpression expr) {
JCParens tree = new JCParens(expr);
tree.pos = pos;
return tree;
}
public JCAssign Assign(JCExpression lhs, JCExpression rhs) {
JCAssign tree = new JCAssign(lhs, rhs);
tree.pos = pos;
return tree;
}
public JCAssignOp Assignop(int opcode, JCTree lhs, JCTree rhs) {
JCAssignOp tree = new JCAssignOp(opcode, lhs, rhs, null);
tree.pos = pos;
return tree;
}
public JCUnary Unary(int opcode, JCExpression arg) {
JCUnary tree = new JCUnary(opcode, arg);
tree.pos = pos;
return tree;
}
public JCBinary Binary(int opcode, JCExpression lhs, JCExpression rhs) {
JCBinary tree = new JCBinary(opcode, lhs, rhs, null);
tree.pos = pos;
return tree;
}
public JCTypeCast TypeCast(JCTree clazz, JCExpression expr) {
JCTypeCast tree = new JCTypeCast(clazz, expr);
tree.pos = pos;
return tree;
}
public JCInstanceOf TypeTest(JCExpression expr, JCTree clazz) {
JCInstanceOf tree = new JCInstanceOf(expr, clazz);
tree.pos = pos;
return tree;
}
public JCArrayAccess Indexed(JCExpression indexed, JCExpression index) {
JCArrayAccess tree = new JCArrayAccess(indexed, index);
tree.pos = pos;
return tree;
}
public JCFieldAccess Select(JCExpression selected, Name selector) {
JCFieldAccess tree = new JCFieldAccess(selected, selector, null);
tree.pos = pos;
return tree;
}
public JCIdent Ident(Name name) {
JCIdent tree = new JCIdent(name, null);
tree.pos = pos;
return tree;
}
// Added by Ceylon
public JCIndyIdent IndyIdent(Name name,
JCExpression indyReturnType, List indyParameterTypes,
JCExpression bsmType, Name bsmName,
List