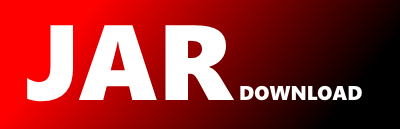
org.jboss.jandex.CompositeIndex Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source.
* Copyright 2013 Red Hat, Inc., and individual contributors
* as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.jandex;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
/**
* Composite annotation index. Represents an aggregation of multiple Index
instances.
* An example application is a Java EE deployment, which can contain multiple nested jars, each with
* their own index.
*
* @author John Bailey
* @author Stuart Douglas
* @author Jason T Greene
*/
public class CompositeIndex implements IndexView {
final Collection indexes;
private CompositeIndex(final Collection indexes) {
this.indexes = indexes;
}
public static CompositeIndex create(Collection indexes) {
return new CompositeIndex(indexes);
}
public static CompositeIndex create(final IndexView... indexes) {
return new CompositeIndex(Arrays.asList(indexes));
}
public static CompositeIndex createMerged(final CompositeIndex... indexes) {
ArrayList list = new ArrayList();
for (CompositeIndex index : indexes) {
list.addAll(index.indexes);
}
return create(list);
}
/**
* {@inheritDoc}
*/
public List getAnnotations(final DotName annotationName) {
final List allInstances = new ArrayList();
for (IndexView index : indexes) {
final Collection list = index.getAnnotations(annotationName);
if (list != null) {
allInstances.addAll(list);
}
}
return Collections.unmodifiableList(allInstances);
}
/**
* {@inheritDoc}
*/
public Set getKnownDirectSubclasses(final DotName className) {
final Set allKnown = new HashSet();
for (IndexView index : indexes) {
final Collection list = index.getKnownDirectSubclasses(className);
if (list != null) {
allKnown.addAll(list);
}
}
return Collections.unmodifiableSet(allKnown);
}
/**
* {@inheritDoc}
*/
public Set getAllKnownSubclasses(final DotName className) {
final Set allKnown = new HashSet();
final Set processedClasses = new HashSet();
getAllKnownSubClasses(className, allKnown, processedClasses);
return allKnown;
}
private void getAllKnownSubClasses(DotName className, Set allKnown, Set processedClasses) {
final Set subClassesToProcess = new HashSet();
subClassesToProcess.add(className);
while (!subClassesToProcess.isEmpty()) {
final Iterator toProcess = subClassesToProcess.iterator();
DotName name = toProcess.next();
toProcess.remove();
processedClasses.add(name);
getAllKnownSubClasses(name, allKnown, subClassesToProcess, processedClasses);
}
}
private void getAllKnownSubClasses(DotName name, Set allKnown, Set subClassesToProcess,
Set processedClasses) {
for (IndexView index : indexes) {
final Collection list = index.getKnownDirectSubclasses(name);
if (list != null) {
for (final ClassInfo clazz : list) {
final DotName className = clazz.name();
if (!processedClasses.contains(className)) {
allKnown.add(clazz);
subClassesToProcess.add(className);
}
}
}
}
}
/**
* {@inheritDoc}
*/
public Collection getKnownDirectImplementors(final DotName className) {
final Set allKnown = new HashSet();
for (IndexView index : indexes) {
final Collection list = index.getKnownDirectImplementors(className);
if (list != null) {
allKnown.addAll(list);
}
}
return Collections.unmodifiableSet(allKnown);
}
/**
* {@inheritDoc}
*/
public Set getAllKnownImplementors(final DotName interfaceName) {
final Set allKnown = new HashSet();
final Set subInterfacesToProcess = new HashSet();
final Set processedClasses = new HashSet();
subInterfacesToProcess.add(interfaceName);
while (!subInterfacesToProcess.isEmpty()) {
final Iterator toProcess = subInterfacesToProcess.iterator();
DotName name = toProcess.next();
toProcess.remove();
processedClasses.add(name);
getKnownImplementors(name, allKnown, subInterfacesToProcess, processedClasses);
}
return allKnown;
}
private void getKnownImplementors(DotName name, Set allKnown, Set subInterfacesToProcess,
Set processedClasses) {
for (IndexView index : indexes) {
final Collection list = index.getKnownDirectImplementors(name);
if (list != null) {
for (final ClassInfo clazz : list) {
final DotName className = clazz.name();
if (!processedClasses.contains(className)) {
if (Modifier.isInterface(clazz.flags())) {
subInterfacesToProcess.add(className);
} else {
if (!allKnown.contains(clazz)) {
allKnown.add(clazz);
processedClasses.add(className);
getAllKnownSubClasses(className, allKnown, processedClasses);
}
}
}
}
}
}
}
/**
* {@inheritDoc}
*/
public ClassInfo getClassByName(final DotName className) {
for (IndexView index : indexes) {
final ClassInfo info = index.getClassByName(className);
if (info != null) {
return info;
}
}
return null;
}
/**
* {@inheritDoc}
*/
public Collection getKnownClasses() {
final List allKnown = new ArrayList();
for (IndexView index : indexes) {
final Collection list = index.getKnownClasses();
if (list != null) {
allKnown.addAll(list);
}
}
return Collections.unmodifiableCollection(allKnown);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy