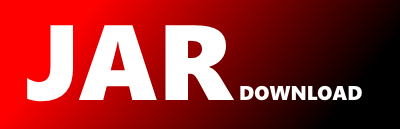
ceylon.language.meta.declaration.ClassDeclaration.ceylon Maven / Gradle / Ivy
import ceylon.language { AnnotationType = Annotation }
import ceylon.language.meta.model {
Class,
MemberClass,
AppliedType = Type,
IncompatibleTypeException,
TypeApplicationException
}
"""Class declaration.
### Callable classes
Since Ceylon 1.2 classes are not always directly invokable
(if the class has constructors, but not a default constructor). Thus
members of `ClassDeclaration` which depend on the class parameter
list typically have optional type, but are refined on
[[ClassWithInitializerDeclaration]] to be non-optional. The exceptions to
this are [[instantiate]] and [[memberInstantiate]],
which throw exceptions.
### Usage sample for toplevel classes
Because some classes have type parameters, getting a model requires applying type arguments to the
class declaration with [[classApply]] in order to be able to instantiate that class. For example, here is how you would
obtain a class model that you can instantiate from a toplevel class declaration:
class Foo(){
string => "Hello, our T is: ``typeLiteral()``";
}
void test(){
// We need to apply the Integer closed type to the Foo declaration in order to get the Foo closed type
Class,[]> classModel = `class Foo`.classApply,[]>(`Integer`);
// This will print: Hello, our T is: ceylon.language::Integer
print(classModel());
}
### Usage sample for member classes
For member classes it is a bit longer, because member classes need to be applied not only their type arguments but also
the containing type, so you should use [[memberClassApply]] and start by giving the containing closed type:
class Outer(){
shared class Inner(){
string => "Hello";
}
}
void test(){
// apply the containing closed type `Outer` to the member class declaration `Outer.Inner`
MemberClass memberClassModel = `class Outer.Inner`.memberClassApply(`Outer`);
// We now have a MemberClass, which needs to be applied to a containing instance in order to become an
// invokable class model:
Class boundMemberClassModel = memberClassModel(Outer());
// This will print: Hello
print(boundMemberClassModel());
}
"""
shared sealed interface ClassDeclaration
of ClassWithInitializerDeclaration | ClassWithConstructorsDeclaration
satisfies ClassOrInterfaceDeclaration {
"A constructor declaration representing the class initializer
(for a class with a parameter list) or
the default constructor, or null if the class lacks
both a parameter list and a default constructor."
since("1.2.0")
shared formal CallableConstructorDeclaration? defaultConstructor;
"The list of parameter declarations for this class.
Returns `null` if the class lacks both a parameter list and a
default constructor."
since("1.2.0")
shared formal FunctionOrValueDeclaration[]? parameterDeclarations;
"Gets a parameter declaration by name.
Returns `null` if this class lacks both a parameter list and a
default constructor,
or if no such parameter exists in the parameter list."
since("1.2.0")
shared formal FunctionOrValueDeclaration? getParameterDeclaration(String name);
"True if the current declaration is an annotation class or function."
since("1.2.0")
shared formal Boolean annotation;
"True if the class has an [[abstract|ceylon.language::abstract]] annotation."
shared formal Boolean abstract;
"True if the class is serializable class."
since("1.2.0")
shared formal Boolean serializable;
"True if the class is an anonymous class, as is the case for the class of object value declarations."
shared formal Boolean anonymous;
"This anonymous class' object value declaration if this class is an anonymous class. `null` otherwise."
since("1.1.0")
shared formal ValueDeclaration? objectValue;
"True if the class has a [[final|ceylon.language::final]] annotation."
shared formal Boolean final;
"Applies the given closed type arguments to this toplevel class declaration in order to obtain a class model.
See [this code sample](#toplevel-sample) for an example on how to use this."
throws(`class IncompatibleTypeException`,
"If the specified `Type` or `Arguments` type arguments are not
compatible with the actual result.")
throws(`class TypeApplicationException`,
"If the specified closed type argument values are not compatible
with the actual result's type parameters.")
shared formal Class classApply
(AppliedType<>* typeArguments)
given Arguments satisfies Anything[];
"Applies the given closed type arguments to this `static` member class declaration
in order to obtain a class model."
throws(`class IncompatibleTypeException`,
"If the specified `Type` or `Arguments` type arguments are not
compatible with the actual result.")
throws(`class TypeApplicationException`,
"If the specified closed type argument values are not compatible
with the actual result's type parameters.")
shared formal Class staticClassApply
(AppliedType
© 2015 - 2024 Weber Informatics LLC | Privacy Policy