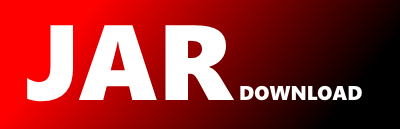
ceylon.language.meta.declaration.ValueDeclaration.ceylon Maven / Gradle / Ivy
import ceylon.language.meta.model {
Value,
Attribute,
AppliedType = Type,
IncompatibleTypeException,
StorageException
}
"""A value declaration.
### Usage sample for toplevel value
Getting a model requires applying type arguments to the
value declaration with [[apply]] in order to be able to read that value. For example, here is how you would
obtain a value model that you can read from a toplevel attribute declaration:
String foo = "Hello";
void test(){
// We need to apply the the foo declaration in order to get the foo value model
Value valueModel = `value foo`.apply();
// This will print: Hello
print(valueModel.get());
}
### Usage sample for attributes
For attributes it is a bit longer, because attributes need to be applied the containing type, so you should
use [[memberApply]] and start by giving the containing closed type:
class Outer(){
shared String foo => "Hello";
}
void test(){
// Apply the containing closed type `Outer` to the attribute declaration `Outer.foo`
Attribute valueModel = `value Outer.foo`.memberApply(`Outer`);
// We now have an Attribute, which needs to be applied to a containing instance in order to become a
// readable value:
Value boundValueModel = valueModel(Outer());
// This will print: Hello
print(boundValueModel.get());
}
"""
shared sealed interface ValueDeclaration
satisfies FunctionOrValueDeclaration & GettableDeclaration {
"True if this declaration is annotated with [[late|ceylon.language::late]]."
since("1.2.0")
shared formal Boolean late;
"True if this declaration is annotated with [[variable|ceylon.language::variable]]."
shared formal Boolean variable;
"True if this declaration is an `object` declaration, whose type is an anonymous class."
since("1.1.0")
shared formal Boolean objectValue;
"This value's anonymous class declaration if this value is an object declaration. `null` otherwise."
since("1.1.0")
shared formal ClassDeclaration? objectClass;
"Applies this value declaration in order to obtain a value model.
See [this code sample](#toplevel-sample) for an example on how to use this."
throws(`class IncompatibleTypeException`, "If the specified `Get` or `Set` type arguments are not compatible with the actual result.")
shared formal Value apply();
"Applies this `static` value declaration in order to obtain a value model."
throws(`class IncompatibleTypeException`, "If the specified `Get` or `Set` type arguments are not compatible with the actual result.")
shared formal Value staticApply(AppliedType
© 2015 - 2024 Weber Informatics LLC | Privacy Policy