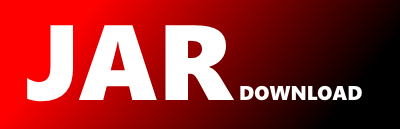
com.redhat.ceylon.cmr.impl.AbstractCeylonArtifactResult Maven / Gradle / Ivy
/*
* Copyright 2011 Red Hat inc. and third party contributors as noted
* by the author tags.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.redhat.ceylon.cmr.impl;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import com.redhat.ceylon.cmr.api.CmrRepository;
import com.redhat.ceylon.cmr.api.ModuleDependencyInfo;
import com.redhat.ceylon.cmr.api.ModuleInfo;
import com.redhat.ceylon.cmr.api.Overrides;
import com.redhat.ceylon.cmr.api.PathFilterParser;
import com.redhat.ceylon.cmr.api.RepositoryManager;
import com.redhat.ceylon.common.ModuleUtil;
import com.redhat.ceylon.model.cmr.ArtifactResult;
import com.redhat.ceylon.model.cmr.ArtifactResultType;
import com.redhat.ceylon.model.cmr.PathFilter;
import com.redhat.ceylon.model.cmr.Repository;
import com.redhat.ceylon.model.cmr.RepositoryException;
/**
* Abstract, use Jandex to read off Module info.
*
* @author Ales Justin
*/
public abstract class AbstractCeylonArtifactResult extends AbstractArtifactResult {
private RepositoryManager manager;
private ModuleInfo infos;
private boolean resolved = false;
protected AbstractCeylonArtifactResult(Repository repository, RepositoryManager manager,
String name, String version) {
super(repository, null, name, version);
this.manager = manager;
}
@Override
public ArtifactResultType type() {
return ArtifactResultType.CEYLON;
}
protected ModuleInfo resolve(){
if(!resolved){
Overrides overrides = ((CmrRepository)repository()).getRoot().getService(Overrides.class);
this.infos = Configuration.getResolvers(manager).resolve(this, overrides);
resolved = true;
}
return infos;
}
protected RepositoryManager getManager(){
return manager;
}
@Override
public PathFilter filter(){
ModuleInfo infos = resolve();
if(infos == null || infos.getFilter() == null)
return null;
try {
return PathFilterParser.parse(infos.getFilter());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public List dependencies() throws RepositoryException {
ModuleInfo infos = resolve();
// TODO -- perhaps null is not valid?
if (infos == null || infos.getDependencies().isEmpty())
return Collections.emptyList();
final List results = new ArrayList();
for (ModuleDependencyInfo mi : getOrderedDependencies(infos)) {
results.add(new LazyArtifactResult(manager,
mi.getNamespace(),
mi.getName(),
mi.getVersion(),
mi.isExport(),
mi.isOptional(),
mi.getModuleScope()));
}
return results;
}
/**
* {@code ModuleInfo} has dependencies as a {@code Set} (actually a
* {@code HashSet}), but {@link #dependencies()} needs to return a list.
* The order matter much except when several dependencies can't be found.
* That happens, in particular, when trying to run a module compiled with a
* more recent (but still BC) version of Ceylon. So for consistency
* on that case we will already return ceylon.language first.
*/
private List getOrderedDependencies(ModuleInfo infos) {
List dependencies = new ArrayList(infos.getDependencies());
for (int index = 0; index < dependencies.size(); index++) {
ModuleDependencyInfo dep = dependencies.get(index);
if ("ceylon.language".equals(dep.getName())) {
if (index != 0) {
dependencies.remove(index);
dependencies.add(0, dep);
}
break;
}
}
return dependencies;
}
@Override
public String artifactId() {
ModuleInfo info = resolve();
if(info != null){
String groupId = info.getGroupId();
String artifactId = info.getArtifactId();
if(groupId != null && !groupId.isEmpty()){
if(artifactId != null && !artifactId.isEmpty())
return artifactId;
// if we have a group but not artifact, default to the entire name
return name();
}
}
return ModuleUtil.getMavenCoordinates(name())[1];
}
@Override
public String groupId() {
ModuleInfo info = resolve();
if(info != null){
String groupId = info.getGroupId();
if(groupId != null && !groupId.isEmpty()){
return groupId;
}
}
return ModuleUtil.getMavenCoordinates(name())[0];
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy