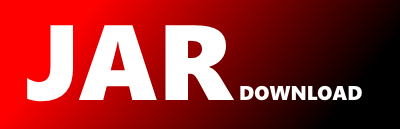
com.redhat.ceylon.common.tool.CeylonArgumentParsers Maven / Gradle / Ivy
package com.redhat.ceylon.common.tool;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
public class CeylonArgumentParsers extends ArgumentParserFactory {
private static Class> ceylonString;
private static Class> ceylonInteger;
private static Class> ceylonBoolean;
private static Method stringInstance;
private static Method integerInstance;
private static Method booleanInstance;
static {
try {
ceylonString = Class.forName("ceylon.language.String");
stringInstance = ceylonString.getDeclaredMethod("instance", String.class);
} catch (Exception e) {
ceylonString = null;
stringInstance = null;
}
try {
ceylonInteger = Class.forName("ceylon.language.Integer");
integerInstance = ceylonInteger.getDeclaredMethod("instance", long.class);
} catch (Exception e) {
ceylonInteger = null;
}
try {
ceylonBoolean = Class.forName("ceylon.language.Boolean");
booleanInstance = ceylonBoolean.getDeclaredMethod("instance", boolean.class);
} catch (Exception e) {
ceylonBoolean = null;
}
}
public static final ArgumentParser
© 2015 - 2024 Weber Informatics LLC | Privacy Policy