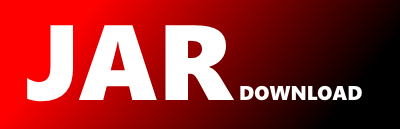
com.redhat.ceylon.model.loader.ClassFileUtil Maven / Gradle / Ivy
package com.redhat.ceylon.model.loader;
import com.redhat.ceylon.langtools.classfile.Annotation;
import com.redhat.ceylon.langtools.classfile.ClassFile;
import com.redhat.ceylon.langtools.classfile.ConstantPool;
import com.redhat.ceylon.langtools.classfile.ConstantPoolException;
import com.redhat.ceylon.langtools.classfile.RuntimeAnnotations_attribute;
import com.redhat.ceylon.langtools.classfile.Annotation.Annotation_element_value;
import com.redhat.ceylon.langtools.classfile.Annotation.Array_element_value;
import com.redhat.ceylon.langtools.classfile.Annotation.Class_element_value;
import com.redhat.ceylon.langtools.classfile.Annotation.Enum_element_value;
import com.redhat.ceylon.langtools.classfile.Annotation.Primitive_element_value;
import com.redhat.ceylon.langtools.classfile.Annotation.element_value;
import com.redhat.ceylon.langtools.classfile.Annotation.element_value_pair;
import com.redhat.ceylon.langtools.classfile.Attribute;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_Class_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_Double_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_Fieldref_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_Float_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_Integer_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_InterfaceMethodref_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_InvokeDynamic_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_Long_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_MethodHandle_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_MethodType_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_Methodref_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_NameAndType_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_String_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CONSTANT_Utf8_info;
import com.redhat.ceylon.langtools.classfile.ConstantPool.CPInfo;
import com.redhat.ceylon.langtools.classfile.ConstantPool.InvalidIndex;
import com.redhat.ceylon.langtools.classfile.ConstantPool.UnexpectedEntry;
public class ClassFileUtil {
private static final ConstantPool.Visitor
© 2015 - 2024 Weber Informatics LLC | Privacy Policy