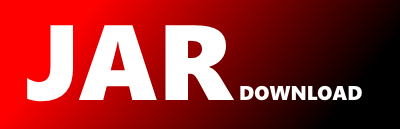
com.redhat.ceylon.tools.p2.ModuleInfo Maven / Gradle / Ivy
/*
* Copyright Red Hat Inc. and/or its affiliates and other contributors
* as indicated by the authors tag. All rights reserved.
*
* This copyrighted material is made available to anyone wishing to use,
* modify, copy, or redistribute it subject to the terms and conditions
* of the GNU General Public License version 2.
*
* This particular file is subject to the "Classpath" exception as provided in the
* LICENSE file that accompanied this code.
*
* This program is distributed in the hope that it will be useful, but WITHOUT A
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A
* PARTICULAR PURPOSE. See the GNU General Public License for more details.
* You should have received a copy of the GNU General Public License,
* along with this distribution; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*/
package com.redhat.ceylon.tools.p2;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.List;
import java.util.SortedMap;
import java.util.TreeMap;
import java.util.jar.Attributes;
import java.util.jar.JarFile;
import java.util.jar.Manifest;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import com.redhat.ceylon.common.ModuleSpec;
class ModuleInfo {
private static final String[] PropertyNames = new String[]{
"Bundle-Name",
"Bundle-Vendor",
"Bundle-Description",
"Bundle-DocUrl",
};
final String name, version;
final File jar;
Attributes osgiAttributes;
String osgiVersion;
private CeylonP2Tool tool;
public static class Dependency extends ModuleSpec {
private boolean optional;
public Dependency(String name, String version) {
this(name, version, false);
}
public Dependency(String name, String version, boolean optional) {
super(null, name, version);
this.optional = optional;
}
public boolean isOptional() {
return optional;
}
}
public ModuleInfo(CeylonP2Tool tool, String name, String version, File jar) throws IOException {
this.tool = tool;
this.name = name;
this.version = version;
this.jar = jar;
loadOsgiVersion();
}
private void loadOsgiVersion() throws IOException {
JarFile jarFile = new JarFile(jar);
Manifest manifest = jarFile.getManifest();
osgiVersion = version;
if(manifest != null){
osgiAttributes = manifest.getMainAttributes();
if(osgiAttributes != null){
String tmp = osgiAttributes.getValue("Bundle-Version");
if(tmp != null)
osgiVersion = tmp;
}
}
jarFile.close();
osgiVersion = CeylonP2Tool.fixOsgiVersion(osgiVersion);
}
public List getExportedPackages() {
String value = osgiAttributes.getValue("Export-Package");
List ret = new LinkedList<>();
if(value != null){
for(String pkg : split(value, ",")){
String[] details = split(pkg, ";");
String name = details[0];
String version = "";// GRRR: API of ModuleInfo
for(int i=1;i matchList = new ArrayList();
int start = 0;
String stringWithReplacedQuotes = "";
while (matcher.find(start)) {
String match= matcher.group(0);
char matchFirstChar = match.charAt(0);
char matchLastChar = match.charAt(match.length()-1);
if (matchFirstChar == '\'' && matchLastChar == '\'' ||
matchFirstChar == '\"' && matchLastChar == '\"') {
matchList.add(match);
stringWithReplacedQuotes += quoteTag;
} else {
stringWithReplacedQuotes += match;
}
start = matcher.end();
}
String[] splittedStringWithReplacedQuotes = stringWithReplacedQuotes.split(separator);
int quoteIndex = 0;
for (int i=0; i getImportedPackages() {
String value = osgiAttributes.getValue("Import-Package");
List ret = new LinkedList<>();
if(value != null){
for(String pkg : split(value, ",")){
String[] details = split(pkg, ";");
String name = details[0];
String version = "";// GRRR: API of ModuleInfo
boolean optional = false;
for(int i=1;i getImportedModules() {
String value = osgiAttributes.getValue("Require-Bundle");
List ret = new LinkedList<>();
if(value != null && !value.isEmpty()){
for(String pkg : split(value, ",")){
String[] details = split(pkg, ";");
String name = details[0];
boolean optional = false;
// skip some modules
if(tool.skipModule(name))
continue;
String version = "";// GRRR: API of ModuleInfo
for(int i=1;i getOsgiProperties() {
SortedMap ret = new TreeMap<>();
for(String name : PropertyNames){
String attribute = getAttribute(name);
if(attribute != null){
ret.put(name, attribute);
}
}
return ret;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy