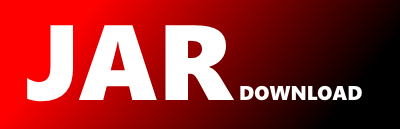
org.jboss.logmanager.config.LogContextConfigurationImpl Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source.
*
* Copyright 2014 Red Hat, Inc., and individual contributors
* as indicated by the @author tags.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jboss.logmanager.config;
import java.nio.charset.Charset;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Deque;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TimeZone;
import org.jboss.logmanager.LogContext;
import org.jboss.logmanager.Logger;
import org.jboss.logmanager.filters.AcceptAllFilter;
import org.jboss.logmanager.filters.AllFilter;
import org.jboss.logmanager.filters.AnyFilter;
import org.jboss.logmanager.filters.DenyAllFilter;
import org.jboss.logmanager.filters.InvertFilter;
import org.jboss.logmanager.filters.LevelChangingFilter;
import org.jboss.logmanager.filters.LevelFilter;
import org.jboss.logmanager.filters.LevelRangeFilter;
import org.jboss.logmanager.filters.RegexFilter;
import org.jboss.logmanager.filters.SubstituteFilter;
import java.util.logging.ErrorManager;
import java.util.logging.Filter;
import java.util.logging.Formatter;
import java.util.logging.Handler;
import java.util.logging.Level;
import static java.lang.Character.isJavaIdentifierPart;
import static java.lang.Character.isJavaIdentifierStart;
import static java.lang.Character.isWhitespace;
/**
* @author David M. Lloyd
*/
final class LogContextConfigurationImpl implements LogContextConfiguration {
private final LogContext logContext;
private final Map loggers = new HashMap();
private final Map handlers = new HashMap();
private final Map formatters = new HashMap();
private final Map filters = new HashMap();
private final Map errorManagers = new HashMap();
private final Map pojos = new HashMap();
private final Map loggerRefs = new HashMap();
private final Map handlerRefs = new HashMap();
private final Map filterRefs = new HashMap();
private final Map formatterRefs = new HashMap();
private final Map errorManagerRefs = new HashMap();
private final Map pojoRefs = new HashMap();
private final Deque> transactionState = new ArrayDeque>();
private final Map>> postConfigurationTransactionState = new LinkedHashMap>>();
private final Deque> preparedTransactions = new ArrayDeque>();
private boolean prepared = false;
private static final ObjectProducer ACCEPT_PRODUCER = new SimpleObjectProducer(AcceptAllFilter.getInstance());
private static final ObjectProducer DENY_PRODUCER = new SimpleObjectProducer(DenyAllFilter.getInstance());
LogContextConfigurationImpl(final LogContext logContext) {
this.logContext = logContext;
}
public LogContext getLogContext() {
return logContext;
}
public LoggerConfiguration addLoggerConfiguration(final String loggerName) {
if (loggers.containsKey(loggerName)) {
throw new IllegalArgumentException(String.format("Logger \"%s\" already exists", loggerName));
}
final LoggerConfigurationImpl loggerConfiguration = new LoggerConfigurationImpl(loggerName, this);
loggers.put(loggerName, loggerConfiguration);
transactionState.addLast(new ConfigAction() {
public Logger validate() throws IllegalArgumentException {
return logContext.getLogger(loggerName);
}
public void applyPreCreate(final Logger param) {
loggerRefs.put(loggerName, param);
}
public void applyPostCreate(Logger param) {
}
public void rollback() {
loggers.remove(loggerName);
}
});
return loggerConfiguration;
}
public boolean removeLoggerConfiguration(final String loggerName) {
final LoggerConfigurationImpl removed = loggers.remove(loggerName);
if (removed != null) {
transactionState.addLast(removed.getRemoveAction());
removed.setRemoved();
return true;
} else {
return false;
}
}
public LoggerConfiguration getLoggerConfiguration(final String loggerName) {
return loggers.get(loggerName);
}
public List getLoggerNames() {
return new ArrayList(loggers.keySet());
}
public HandlerConfiguration addHandlerConfiguration(final String moduleName, final String className, final String handlerName, final String... constructorProperties) {
if (handlers.containsKey(handlerName)) {
throw new IllegalArgumentException(String.format("Handler \"%s\" already exists", handlerName));
}
final HandlerConfigurationImpl handlerConfiguration = new HandlerConfigurationImpl(this, handlerName, moduleName, className, constructorProperties);
handlers.put(handlerName, handlerConfiguration);
addAction(handlerConfiguration.getConstructAction());
return handlerConfiguration;
}
public boolean removeHandlerConfiguration(final String handlerName) {
final HandlerConfigurationImpl removed = handlers.remove(handlerName);
if (removed != null) {
transactionState.addLast(removed.getRemoveAction());
removed.setRemoved();
return true;
} else {
return false;
}
}
public HandlerConfiguration getHandlerConfiguration(final String handlerName) {
return handlers.get(handlerName);
}
public List getHandlerNames() {
return new ArrayList(handlers.keySet());
}
public FormatterConfiguration addFormatterConfiguration(final String moduleName, final String className, final String formatterName, final String... constructorProperties) {
if (formatters.containsKey(formatterName)) {
throw new IllegalArgumentException(String.format("Formatter \"%s\" already exists", formatterName));
}
final FormatterConfigurationImpl formatterConfiguration = new FormatterConfigurationImpl(this, formatterName, moduleName, className, constructorProperties);
formatters.put(formatterName, formatterConfiguration);
addAction(formatterConfiguration.getConstructAction());
return formatterConfiguration;
}
public boolean removeFormatterConfiguration(final String formatterName) {
final FormatterConfigurationImpl removed = formatters.remove(formatterName);
if (removed != null) {
transactionState.addLast(removed.getRemoveAction());
removed.setRemoved();
return true;
} else {
return false;
}
}
public FormatterConfiguration getFormatterConfiguration(final String formatterName) {
return formatters.get(formatterName);
}
public List getFormatterNames() {
return new ArrayList(formatters.keySet());
}
public FilterConfiguration addFilterConfiguration(final String moduleName, final String className, final String filterName, final String... constructorProperties) {
if (filters.containsKey(filterName)) {
throw new IllegalArgumentException(String.format("Filter \"%s\" already exists", filterName));
}
final FilterConfigurationImpl filterConfiguration = new FilterConfigurationImpl(this, filterName, moduleName, className, constructorProperties);
filters.put(filterName, filterConfiguration);
addAction(filterConfiguration.getConstructAction());
return filterConfiguration;
}
public boolean removeFilterConfiguration(final String filterName) {
final FilterConfigurationImpl removed = filters.remove(filterName);
if (removed != null) {
transactionState.addLast(removed.getRemoveAction());
removed.setRemoved();
return true;
} else {
return false;
}
}
public FilterConfiguration getFilterConfiguration(final String filterName) {
return filters.get(filterName);
}
public List getFilterNames() {
return new ArrayList(filters.keySet());
}
public ErrorManagerConfiguration addErrorManagerConfiguration(final String moduleName, final String className, final String errorManagerName, final String... constructorProperties) {
if (errorManagers.containsKey(errorManagerName)) {
throw new IllegalArgumentException(String.format("ErrorManager \"%s\" already exists", errorManagerName));
}
final ErrorManagerConfigurationImpl errorManagerConfiguration = new ErrorManagerConfigurationImpl(this, errorManagerName, moduleName, className, constructorProperties);
errorManagers.put(errorManagerName, errorManagerConfiguration);
addAction(errorManagerConfiguration.getConstructAction());
return errorManagerConfiguration;
}
public boolean removeErrorManagerConfiguration(final String errorManagerName) {
final ErrorManagerConfigurationImpl removed = errorManagers.remove(errorManagerName);
if (removed != null) {
transactionState.addLast(removed.getRemoveAction());
removed.setRemoved();
return true;
} else {
return false;
}
}
public ErrorManagerConfiguration getErrorManagerConfiguration(final String errorManagerName) {
return errorManagers.get(errorManagerName);
}
public List getErrorManagerNames() {
return new ArrayList(errorManagers.keySet());
}
@Override
public PojoConfiguration addPojoConfiguration(final String moduleName, final String className, final String pojoName, final String... constructorProperties) {
if (pojos.containsKey(pojoName)) {
throw new IllegalArgumentException(String.format("POJO \"%s\" already exists", pojoName));
}
final PojoConfigurationImpl pojoConfiguration = new PojoConfigurationImpl(this, pojoName, moduleName, className, constructorProperties);
pojos.put(pojoName, pojoConfiguration);
transactionState.addLast(pojoConfiguration.getConstructAction());
return pojoConfiguration;
}
@Override
public boolean removePojoConfiguration(final String pojoName) {
final PojoConfigurationImpl removed = pojos.remove(pojoName);
if (removed != null) {
transactionState.addLast(removed.getRemoveAction());
removed.setRemoved();
return true;
}
return false;
}
@Override
public PojoConfiguration getPojoConfiguration(final String pojoName) {
return pojos.get(pojoName);
}
@Override
public List getPojoNames() {
return new ArrayList(pojos.keySet());
}
@Override
public void prepare() {
doPrepare(transactionState);
for (Deque> items : postConfigurationTransactionState.values()) {
doPrepare(items);
}
prepared = true;
}
public void commit() {
if (!prepared) {
prepare();
}
clear();
}
@SuppressWarnings("unchecked")
private static void doApplyPreCreate(ConfigAction action, Object arg) {
try {
action.applyPreCreate((T) arg);
} catch (Throwable ignored) {}
}
@SuppressWarnings("unchecked")
private static void doApplyPostCreate(ConfigAction action, Object arg) {
try {
action.applyPostCreate((T) arg);
} catch (Throwable ignored) {}
}
public void forget() {
doForget(transactionState);
doForget(preparedTransactions);
for (Deque> items : postConfigurationTransactionState.values()) {
doForget(items);
}
clear();
}
private void clear() {
prepared = false;
postConfigurationTransactionState.clear();
transactionState.clear();
preparedTransactions.clear();
}
private void doPrepare(final Deque> transactionState) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy