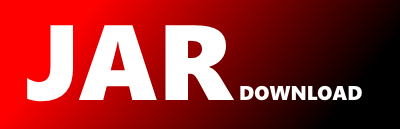
org.chartistjsf.model.chart.ChartModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ChartistJSF Show documentation
Show all versions of ChartistJSF Show documentation
Highly Customizable Responsive Charts for JSF based on PrimeFaces
/*
* Copyright 2015 ChartistJSF.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.chartistjsf.model.chart;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
*
* The main model for ChartistJSF
*
*
* @author Hatem Alimam
* @since 0.1
*/
public class ChartModel implements Serializable {
/**
*
*/
private static final long serialVersionUID = -8649635660286079308L;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy