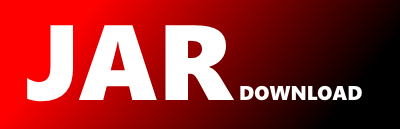
org.checkerframework.dataflow.busyexpr.BusyExprTransfer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dataflow-shaded Show documentation
Show all versions of dataflow-shaded Show documentation
dataflow-shaded is a dataflow framework based on the javac compiler.
It differs from the org.checkerframework:dataflow artifact in two ways.
First, the packages in this artifact have been renamed to org.checkerframework.shaded.*.
Second, unlike the dataflow artifact, this artifact contains the dependencies it requires.
package org.checkerframework.dataflow.busyexpr;
import java.util.List;
import org.checkerframework.dataflow.analysis.BackwardTransferFunction;
import org.checkerframework.dataflow.analysis.RegularTransferResult;
import org.checkerframework.dataflow.analysis.TransferInput;
import org.checkerframework.dataflow.analysis.TransferResult;
import org.checkerframework.dataflow.analysis.UnusedAbstractValue;
import org.checkerframework.dataflow.cfg.UnderlyingAST;
import org.checkerframework.dataflow.cfg.node.AbstractNodeVisitor;
import org.checkerframework.dataflow.cfg.node.AssignmentNode;
import org.checkerframework.dataflow.cfg.node.MethodInvocationNode;
import org.checkerframework.dataflow.cfg.node.Node;
import org.checkerframework.dataflow.cfg.node.ObjectCreationNode;
import org.checkerframework.dataflow.cfg.node.ReturnNode;
/** A busy expression transfer function */
public class BusyExprTransfer
extends AbstractNodeVisitor<
TransferResult,
TransferInput>
implements BackwardTransferFunction {
@Override
public BusyExprStore initialNormalExitStore(
UnderlyingAST underlyingAST, List returnNodes) {
return new BusyExprStore();
}
@Override
public BusyExprStore initialExceptionalExitStore(UnderlyingAST underlyingAST) {
return new BusyExprStore();
}
@Override
public RegularTransferResult visitNode(
Node n, TransferInput p) {
return new RegularTransferResult<>(null, p.getRegularStore());
}
@Override
public RegularTransferResult visitAssignment(
AssignmentNode n, TransferInput p) {
RegularTransferResult transferResult =
(RegularTransferResult) super.visitAssignment(n, p);
BusyExprStore store = transferResult.getRegularStore();
store.killBusyExpr(n.getTarget());
store.addUseInExpression(n.getExpression());
return transferResult;
}
@Override
public RegularTransferResult visitMethodInvocation(
MethodInvocationNode n, TransferInput p) {
RegularTransferResult transferResult =
(RegularTransferResult)
super.visitMethodInvocation(n, p);
BusyExprStore store = transferResult.getRegularStore();
for (Node arg : n.getArguments()) {
store.addUseInExpression(arg);
}
return transferResult;
}
@Override
public RegularTransferResult visitObjectCreation(
ObjectCreationNode n, TransferInput p) {
RegularTransferResult transferResult =
(RegularTransferResult) super.visitObjectCreation(n, p);
BusyExprStore store = transferResult.getRegularStore();
for (Node arg : n.getArguments()) {
store.addUseInExpression(arg);
}
return transferResult;
}
@Override
public RegularTransferResult visitReturn(
ReturnNode n, TransferInput p) {
RegularTransferResult transferResult =
(RegularTransferResult) super.visitReturn(n, p);
Node result = n.getResult();
if (result != null) {
BusyExprStore store = transferResult.getRegularStore();
store.addUseInExpression(result);
}
return transferResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy