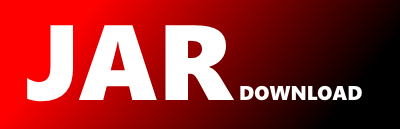
org.checkerframework.dataflow.expression.Unknown Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dataflow-shaded Show documentation
Show all versions of dataflow-shaded Show documentation
dataflow-shaded is a dataflow framework based on the javac compiler.
It differs from the org.checkerframework:dataflow artifact in two ways.
First, the packages in this artifact have been renamed to org.checkerframework.shaded.*.
Second, unlike the dataflow artifact, this artifact contains the dependencies it requires.
package org.checkerframework.dataflow.expression;
import com.sun.source.tree.Tree;
import javax.lang.model.type.TypeMirror;
import org.checkerframework.checker.interning.qual.UsesObjectEquals;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.checkerframework.dataflow.analysis.Store;
import org.checkerframework.dataflow.cfg.node.Node;
import org.checkerframework.javacutil.AnnotationProvider;
import org.checkerframework.javacutil.TreeUtils;
/** Stands for any expression that the Dataflow Framework lacks explicit support for. */
@UsesObjectEquals
public class Unknown extends JavaExpression {
/** String representation of the expression that has no corresponding {@code JavaExpression}. */
private final String originalExpression;
/**
* Create a new Unknown JavaExpression.
*
* @param type the Java type of this
*/
public Unknown(TypeMirror type) {
this(type, "?");
}
/**
* Create a new Unknown JavaExpression.
*
* @param type the Java type of this
* @param originalExpression a String representation of the expression that has no corresponding
* {@code JavaExpression}
*/
public Unknown(TypeMirror type, String originalExpression) {
super(type);
this.originalExpression = originalExpression;
}
/**
* Create a new Unknown JavaExpression.
*
* @param tree a tree that does not have a corresponding {@code JavaExpression}
*/
public Unknown(Tree tree) {
this(TreeUtils.typeOf(tree), TreeUtils.toStringTruncated(tree, 40));
}
/**
* Create a new Unknown JavaExpression.
*
* @param node a node that does not have a corresponding {@code JavaExpression}
*/
public Unknown(Node node) {
this(node.getType(), node.toString());
}
@Override
public boolean equals(@Nullable Object obj) {
return obj == this;
}
// Overridden to avoid an error "overrides equals, but does not override hashCode"
@Override
public int hashCode() {
return System.identityHashCode(this);
}
@Override
public String toString() {
return originalExpression;
}
@SuppressWarnings("unchecked") // generic cast
@Override
public @Nullable T containedOfClass(Class clazz) {
return getClass() == clazz ? (T) this : null;
}
@Override
public boolean isDeterministic(AnnotationProvider provider) {
return false;
}
@Override
public boolean isAssignableByOtherCode() {
return true;
}
@Override
public boolean isModifiableByOtherCode() {
return true;
}
@Override
public boolean syntacticEquals(JavaExpression je) {
return this == je;
}
@Override
public boolean containsSyntacticEqualJavaExpression(JavaExpression other) {
return this.syntacticEquals(other);
}
@Override
public boolean containsModifiableAliasOf(Store> store, JavaExpression other) {
return true;
}
@Override
public R accept(JavaExpressionVisitor visitor, P p) {
return visitor.visitUnknown(this, p);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy