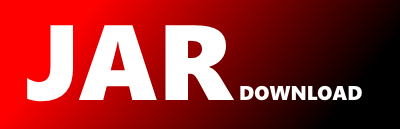
com.github.javaparser.ast.PackageDeclaration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stubparser Show documentation
Show all versions of stubparser Show documentation
This project contains a parser for the Checker Framework's stub files: https://checkerframework.org/manual/#stub . It is a fork of the JavaParser project.
The newest version!
/*
* Copyright (C) 2007-2010 Júlio Vilmar Gesser.
* Copyright (C) 2011, 2013-2024 The JavaParser Team.
*
* This file is part of JavaParser.
*
* JavaParser can be used either under the terms of
* a) the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* b) the terms of the Apache License
*
* You should have received a copy of both licenses in LICENCE.LGPL and
* LICENCE.APACHE. Please refer to those files for details.
*
* JavaParser is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package com.github.javaparser.ast;
import static com.github.javaparser.utils.Utils.assertNotNull;
import com.github.javaparser.TokenRange;
import com.github.javaparser.ast.expr.AnnotationExpr;
import com.github.javaparser.ast.expr.Name;
import com.github.javaparser.ast.nodeTypes.NodeWithAnnotations;
import com.github.javaparser.ast.nodeTypes.NodeWithName;
import com.github.javaparser.ast.observer.ObservableProperty;
import com.github.javaparser.ast.visitor.CloneVisitor;
import com.github.javaparser.ast.visitor.GenericVisitor;
import com.github.javaparser.ast.visitor.VoidVisitor;
import com.github.javaparser.metamodel.JavaParserMetaModel;
import com.github.javaparser.metamodel.PackageDeclarationMetaModel;
/**
* A package declaration.
*
{@code package com.github.javaparser.ast;}
*
{@code @Wonderful package anything.can.be.annotated.nowadays;}
*
* @author Julio Vilmar Gesser
*/
public class PackageDeclaration extends Node
implements NodeWithAnnotations, NodeWithName {
private NodeList annotations = new NodeList<>();
private Name name;
public PackageDeclaration() {
this(null, new NodeList<>(), new Name());
}
public PackageDeclaration(Name name) {
this(null, new NodeList<>(), name);
}
@AllFieldsConstructor
public PackageDeclaration(NodeList annotations, Name name) {
this(null, annotations, name);
}
/**
* This constructor is used by the parser and is considered private.
*/
@Generated("com.github.javaparser.generator.core.node.MainConstructorGenerator")
public PackageDeclaration(TokenRange tokenRange, NodeList annotations, Name name) {
super(tokenRange);
setAnnotations(annotations);
setName(name);
customInitialization();
}
@Override
@Generated("com.github.javaparser.generator.core.node.AcceptGenerator")
public R accept(final GenericVisitor v, final A arg) {
return v.visit(this, arg);
}
@Override
@Generated("com.github.javaparser.generator.core.node.AcceptGenerator")
public void accept(final VoidVisitor v, final A arg) {
v.visit(this, arg);
}
/**
* Retrieves the list of annotations declared before the package
* declaration. Return {@code null} if there are no annotations.
*
* @return list of annotations or {@code null}
*/
@Generated("com.github.javaparser.generator.core.node.PropertyGenerator")
public NodeList getAnnotations() {
return annotations;
}
/**
* Return the name expression of the package.
*
* @return the name of the package
*/
@Generated("com.github.javaparser.generator.core.node.PropertyGenerator")
public Name getName() {
return name;
}
/**
* @param annotations the annotations to set
*/
@Generated("com.github.javaparser.generator.core.node.PropertyGenerator")
public PackageDeclaration setAnnotations(final NodeList annotations) {
assertNotNull(annotations);
if (annotations == this.annotations) {
return this;
}
notifyPropertyChange(ObservableProperty.ANNOTATIONS, this.annotations, annotations);
if (this.annotations != null) this.annotations.setParentNode(null);
this.annotations = annotations;
setAsParentNodeOf(annotations);
return this;
}
/**
* Sets the name of this package declaration.
*
* @param name the name to set
*/
@Generated("com.github.javaparser.generator.core.node.PropertyGenerator")
public PackageDeclaration setName(final Name name) {
assertNotNull(name);
if (name == this.name) {
return this;
}
notifyPropertyChange(ObservableProperty.NAME, this.name, name);
if (this.name != null) this.name.setParentNode(null);
this.name = name;
setAsParentNodeOf(name);
return this;
}
@Override
@Generated("com.github.javaparser.generator.core.node.RemoveMethodGenerator")
public boolean remove(Node node) {
if (node == null) {
return false;
}
for (int i = 0; i < annotations.size(); i++) {
if (annotations.get(i) == node) {
annotations.remove(i);
return true;
}
}
return super.remove(node);
}
@Override
@Generated("com.github.javaparser.generator.core.node.CloneGenerator")
public PackageDeclaration clone() {
return (PackageDeclaration) accept(new CloneVisitor(), null);
}
@Override
@Generated("com.github.javaparser.generator.core.node.GetMetaModelGenerator")
public PackageDeclarationMetaModel getMetaModel() {
return JavaParserMetaModel.packageDeclarationMetaModel;
}
@Override
@Generated("com.github.javaparser.generator.core.node.ReplaceMethodGenerator")
public boolean replace(Node node, Node replacementNode) {
if (node == null) {
return false;
}
for (int i = 0; i < annotations.size(); i++) {
if (annotations.get(i) == node) {
annotations.set(i, (AnnotationExpr) replacementNode);
return true;
}
}
if (node == name) {
setName((Name) replacementNode);
return true;
}
return super.replace(node, replacementNode);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy