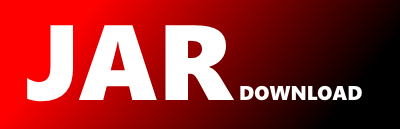
com.github.javaparser.ast.body.FieldDeclaration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stubparser Show documentation
Show all versions of stubparser Show documentation
This project contains a parser for the Checker Framework's stub files: https://checkerframework.org/manual/#stub . It is a fork of the JavaParser project.
The newest version!
/*
* Copyright (C) 2007-2010 Júlio Vilmar Gesser.
* Copyright (C) 2011, 2013-2024 The JavaParser Team.
*
* This file is part of JavaParser.
*
* JavaParser can be used either under the terms of
* a) the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
* b) the terms of the Apache License
*
* You should have received a copy of both licenses in LICENCE.LGPL and
* LICENCE.APACHE. Please refer to those files for details.
*
* JavaParser is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package com.github.javaparser.ast.body;
import static com.github.javaparser.ast.Modifier.Keyword.STATIC;
import static com.github.javaparser.ast.NodeList.nodeList;
import static com.github.javaparser.utils.Utils.assertNotNull;
import com.github.javaparser.TokenRange;
import com.github.javaparser.ast.*;
import com.github.javaparser.ast.Modifier.Keyword;
import com.github.javaparser.ast.expr.AnnotationExpr;
import com.github.javaparser.ast.expr.AssignExpr;
import com.github.javaparser.ast.expr.AssignExpr.Operator;
import com.github.javaparser.ast.expr.NameExpr;
import com.github.javaparser.ast.nodeTypes.NodeWithJavadoc;
import com.github.javaparser.ast.nodeTypes.NodeWithVariables;
import com.github.javaparser.ast.nodeTypes.modifiers.NodeWithAccessModifiers;
import com.github.javaparser.ast.nodeTypes.modifiers.NodeWithFinalModifier;
import com.github.javaparser.ast.nodeTypes.modifiers.NodeWithStaticModifier;
import com.github.javaparser.ast.observer.ObservableProperty;
import com.github.javaparser.ast.stmt.BlockStmt;
import com.github.javaparser.ast.stmt.ReturnStmt;
import com.github.javaparser.ast.type.Type;
import com.github.javaparser.ast.type.VoidType;
import com.github.javaparser.ast.visitor.CloneVisitor;
import com.github.javaparser.ast.visitor.GenericVisitor;
import com.github.javaparser.ast.visitor.VoidVisitor;
import com.github.javaparser.metamodel.FieldDeclarationMetaModel;
import com.github.javaparser.metamodel.JavaParserMetaModel;
import com.github.javaparser.metamodel.NonEmptyProperty;
import com.github.javaparser.resolution.Resolvable;
import com.github.javaparser.resolution.declarations.ResolvedFieldDeclaration;
import java.util.Optional;
import java.util.function.Consumer;
/**
* The declaration of a field in a class. "private static int a=15*15;" in this example: {@code class X { private static
* int a=15*15; }}
*
*
All annotations preceding the type will be set on this object, not on the type.
* JavaParser doesn't know if it they are applicable to the method or the type.
*
* @author Julio Vilmar Gesser
*/
public class FieldDeclaration extends BodyDeclaration
implements NodeWithJavadoc,
NodeWithVariables,
NodeWithAccessModifiers,
NodeWithStaticModifier,
NodeWithFinalModifier,
Resolvable {
private NodeList modifiers;
@NonEmptyProperty
private NodeList variables;
public FieldDeclaration() {
this(null, new NodeList<>(), new NodeList<>(), new NodeList<>());
}
public FieldDeclaration(NodeList modifiers, VariableDeclarator variable) {
this(null, modifiers, new NodeList<>(), nodeList(variable));
}
public FieldDeclaration(NodeList modifiers, NodeList variables) {
this(null, modifiers, new NodeList<>(), variables);
}
@AllFieldsConstructor
public FieldDeclaration(
NodeList modifiers,
NodeList annotations,
NodeList variables) {
this(null, modifiers, annotations, variables);
}
/**
* This constructor is used by the parser and is considered private.
*/
@Generated("com.github.javaparser.generator.core.node.MainConstructorGenerator")
public FieldDeclaration(
TokenRange tokenRange,
NodeList modifiers,
NodeList annotations,
NodeList variables) {
super(tokenRange, annotations);
setModifiers(modifiers);
setVariables(variables);
customInitialization();
}
/**
* Creates a {@link FieldDeclaration}.
*
* @param modifiers modifiers
* @param type type
* @param name field name
*/
public FieldDeclaration(NodeList modifiers, Type type, String name) {
this(assertNotNull(modifiers), new VariableDeclarator(type, assertNotNull(name)));
}
@Override
@Generated("com.github.javaparser.generator.core.node.AcceptGenerator")
public R accept(final GenericVisitor v, final A arg) {
return v.visit(this, arg);
}
@Override
@Generated("com.github.javaparser.generator.core.node.AcceptGenerator")
public void accept(final VoidVisitor v, final A arg) {
v.visit(this, arg);
}
/**
* Return the modifiers of this member declaration.
*
* @return modifiers
* @see Modifier
*/
@Generated("com.github.javaparser.generator.core.node.PropertyGenerator")
public NodeList getModifiers() {
return modifiers;
}
@Generated("com.github.javaparser.generator.core.node.PropertyGenerator")
public NodeList getVariables() {
return variables;
}
@Generated("com.github.javaparser.generator.core.node.PropertyGenerator")
public FieldDeclaration setModifiers(final NodeList modifiers) {
assertNotNull(modifiers);
if (modifiers == this.modifiers) {
return this;
}
notifyPropertyChange(ObservableProperty.MODIFIERS, this.modifiers, modifiers);
if (this.modifiers != null) this.modifiers.setParentNode(null);
this.modifiers = modifiers;
setAsParentNodeOf(modifiers);
return this;
}
@Generated("com.github.javaparser.generator.core.node.PropertyGenerator")
public FieldDeclaration setVariables(final NodeList variables) {
assertNotNull(variables);
if (variables == this.variables) {
return this;
}
notifyPropertyChange(ObservableProperty.VARIABLES, this.variables, variables);
if (this.variables != null) this.variables.setParentNode(null);
this.variables = variables;
setAsParentNodeOf(variables);
return this;
}
/**
* Create a getter for this field, will only work if this field declares only 1 identifier and if this field is
* already added to a ClassOrInterfaceDeclaration
*
* @return the {@link MethodDeclaration} created
* @throws IllegalStateException if there is more than 1 variable identifier or if this field isn't attached to a
* class or enum
*/
public MethodDeclaration createGetter() {
if (getVariables().size() != 1)
throw new IllegalStateException("You can use this only when the field declares only 1 variable name");
Optional parentClass = findAncestor(ClassOrInterfaceDeclaration.class);
Optional parentEnum = findAncestor(EnumDeclaration.class);
if (!(parentClass.isPresent() || parentEnum.isPresent())
|| (parentClass.isPresent() && parentClass.get().isInterface()))
throw new IllegalStateException("You can use this only when the field is attached to a class or an enum");
VariableDeclarator variable = getVariable(0);
String fieldName = variable.getNameAsString();
String fieldNameUpper = fieldName.toUpperCase().substring(0, 1) + fieldName.substring(1, fieldName.length());
final MethodDeclaration getter;
getter = parentClass
.map(clazz -> clazz.addMethod("get" + fieldNameUpper, Modifier.Keyword.PUBLIC))
.orElseGet(() -> parentEnum.get().addMethod("get" + fieldNameUpper, Modifier.Keyword.PUBLIC));
getter.setType(variable.getType());
BlockStmt blockStmt = new BlockStmt();
getter.setBody(blockStmt);
blockStmt.addStatement(new ReturnStmt(fieldName));
return getter;
}
/**
* Create a setter for this field, will only work if this field declares only 1 identifier and if this field is
* already added to a ClassOrInterfaceDeclaration
*
* @return the {@link MethodDeclaration} created
* @throws IllegalStateException if there is more than 1 variable identifier or if this field isn't attached to a
* class or enum
*/
public MethodDeclaration createSetter() {
if (getVariables().size() != 1)
throw new IllegalStateException("You can use this only when the field declares only 1 variable name");
Optional parentClass = findAncestor(ClassOrInterfaceDeclaration.class);
Optional parentEnum = findAncestor(EnumDeclaration.class);
if (!(parentClass.isPresent() || parentEnum.isPresent())
|| (parentClass.isPresent() && parentClass.get().isInterface()))
throw new IllegalStateException("You can use this only when the field is attached to a class or an enum");
VariableDeclarator variable = getVariable(0);
String fieldName = variable.getNameAsString();
String fieldNameUpper = fieldName.toUpperCase().substring(0, 1) + fieldName.substring(1, fieldName.length());
final MethodDeclaration setter;
setter = parentClass
.map(clazz -> clazz.addMethod("set" + fieldNameUpper, Modifier.Keyword.PUBLIC))
.orElseGet(() -> parentEnum.get().addMethod("set" + fieldNameUpper, Modifier.Keyword.PUBLIC));
setter.setType(new VoidType());
setter.getParameters().add(new Parameter(variable.getType(), fieldName));
BlockStmt blockStmt2 = new BlockStmt();
setter.setBody(blockStmt2);
blockStmt2.addStatement(
new AssignExpr(new NameExpr("this." + fieldName), new NameExpr(fieldName), Operator.ASSIGN));
return setter;
}
public boolean isTransient() {
return hasModifier(Modifier.Keyword.TRANSIENT);
}
public boolean isVolatile() {
return hasModifier(Modifier.Keyword.VOLATILE);
}
public FieldDeclaration setTransient(boolean set) {
return setModifier(Modifier.Keyword.TRANSIENT, set);
}
public FieldDeclaration setVolatile(boolean set) {
return setModifier(Modifier.Keyword.VOLATILE, set);
}
/*
* Every field declaration in the body of an interface is implicitly public, static, and final.
*/
@Override
public boolean isStatic() {
return hasModifier(STATIC) || isDeclaredInInterface();
}
/*
* Every field declaration in the body of an interface is implicitly public, static, and final.
*/
@Override
public boolean isFinal() {
return hasModifier(Keyword.FINAL) || isDeclaredInInterface();
}
/*
* Every field declaration in the body of an interface is implicitly public, static, and final.
*/
@Override
public boolean isPublic() {
return hasModifier(Keyword.PUBLIC) || isDeclaredInInterface();
}
/*
* Returns true if the field is declared in an interface
*/
private boolean isDeclaredInInterface() {
Optional parentType = findAncestor(TypeDeclaration.class);
return parentType
.filter(BodyDeclaration::isClassOrInterfaceDeclaration)
.map(BodyDeclaration::asClassOrInterfaceDeclaration)
.map(ClassOrInterfaceDeclaration::isInterface)
.orElse(false);
}
@Override
@Generated("com.github.javaparser.generator.core.node.RemoveMethodGenerator")
public boolean remove(Node node) {
if (node == null) {
return false;
}
for (int i = 0; i < modifiers.size(); i++) {
if (modifiers.get(i) == node) {
modifiers.remove(i);
return true;
}
}
for (int i = 0; i < variables.size(); i++) {
if (variables.get(i) == node) {
variables.remove(i);
return true;
}
}
return super.remove(node);
}
@Override
@Generated("com.github.javaparser.generator.core.node.CloneGenerator")
public FieldDeclaration clone() {
return (FieldDeclaration) accept(new CloneVisitor(), null);
}
@Override
@Generated("com.github.javaparser.generator.core.node.GetMetaModelGenerator")
public FieldDeclarationMetaModel getMetaModel() {
return JavaParserMetaModel.fieldDeclarationMetaModel;
}
@Override
@Generated("com.github.javaparser.generator.core.node.ReplaceMethodGenerator")
public boolean replace(Node node, Node replacementNode) {
if (node == null) {
return false;
}
for (int i = 0; i < modifiers.size(); i++) {
if (modifiers.get(i) == node) {
modifiers.set(i, (Modifier) replacementNode);
return true;
}
}
for (int i = 0; i < variables.size(); i++) {
if (variables.get(i) == node) {
variables.set(i, (VariableDeclarator) replacementNode);
return true;
}
}
return super.replace(node, replacementNode);
}
@Override
@Generated("com.github.javaparser.generator.core.node.TypeCastingGenerator")
public boolean isFieldDeclaration() {
return true;
}
@Override
@Generated("com.github.javaparser.generator.core.node.TypeCastingGenerator")
public FieldDeclaration asFieldDeclaration() {
return this;
}
@Override
@Generated("com.github.javaparser.generator.core.node.TypeCastingGenerator")
public void ifFieldDeclaration(Consumer action) {
action.accept(this);
}
@Override
public ResolvedFieldDeclaration resolve() {
return getSymbolResolver().resolveDeclaration(this, ResolvedFieldDeclaration.class);
}
@Override
@Generated("com.github.javaparser.generator.core.node.TypeCastingGenerator")
public Optional toFieldDeclaration() {
return Optional.of(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy