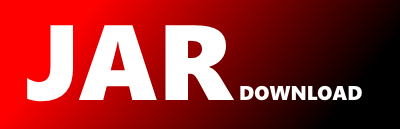
org.cinchapi.concourse.config.ConcourseClientPreferences Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of concourse-config Show documentation
Show all versions of concourse-config Show documentation
A framework for reading and editing Concourse configuration files
The newest version!
/*
* The MIT License (MIT)
*
* Copyright (c) 2014 Jeff Nelson, Cinchapi Software Collective
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.cinchapi.concourse.config;
/**
* A wrapper around the {@code concourse_client.prefs} file that is used to
* configure a client connection.
*
* Instantiate using {@link ConcourseClientPreferences#load(String)}
*
*
* @author jnelson
*/
public class ConcourseClientPreferences extends AbstractPreferences {
/**
* Return a {@link ConcourseClientPreferences} wrapper that is backed by the
* configuration information in {@code file}.
*
* @param file
* @return the preferences
*/
public static ConcourseClientPreferences load(String file) {
return new ConcourseClientPreferences(file);
}
// Defaults
private static final String DEFAULT_HOST = "localhost";
private static final int DEFAULT_PORT = 1717;
private static final String DEFAULT_USERNAME = "admin";
private static final String DEFAULT_PASSWORD = "admin";
private static final String DEFAULT_ENVIRONMENT = "";
/**
* Construct a new instance.
*
* @param file
*/
protected ConcourseClientPreferences(String file) {
super(file);
}
/**
* Return the value associated with the {@code environment} key.
*
* @return the environment
*/
public String getEnvironment() {
return getHandler().getString("environment", DEFAULT_ENVIRONMENT);
}
/**
* Return the value associated with the {@code host} key.
*
* @return the host
*/
public String getHost() {
return getHandler().getString("host", DEFAULT_HOST);
}
/**
* Return the value associated with the {@code password} key.
*
* NOTE: This method returns the password as a char array
* so that the caller can null out the data immediately after use. This is
* generally advised to limit the amount of time that the sensitive data
* remains in memory.
*
*
* @return the password
*/
public char[] getPassword() {
return getHandler().getString("password", DEFAULT_PASSWORD)
.toCharArray();
}
/**
* Return the value associated with the {@code port} key.
*
* @return the port
*/
public int getPort() {
return getHandler().getInt("port", DEFAULT_PORT);
}
/**
* Return the value associated with the {@code username} key.
*
* @return the username
*/
public String getUsername() {
return getHandler().getString("username", DEFAULT_USERNAME);
}
/**
* Set the value associated with the {@code environment} key.
*
* @param environment
*/
public void setEnvironment(String environment) {
set("environment", environment);
}
/**
* Set the value associated with the {@code host} key.
*
* @param host
*/
public void setHost(String host) {
set("host", host);
}
/**
* Set the value associated with the {@code password} key.
*
* @param password
*/
public void setPassword(char[] password) {
set("password", new String(password));
}
/**
* Set the value associated with the {@code port} key.
*
* @param port
*/
public void setPort(int port) {
set("port", port);
}
/**
* Set the value associated with the {@code username} key.
*
* @param username
*/
public void setUsername(String username) {
set("username", username);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy