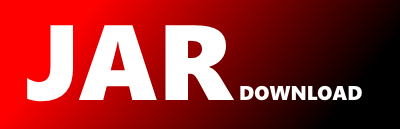
org.cip4.jdflib.util.net.URLProxySelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JDFLibJ Show documentation
Show all versions of JDFLibJ Show documentation
CIP4 JDF Library for JDF 1.x
/**
*
*/
package org.cip4.jdflib.util.net;
import java.io.IOException;
import java.net.InetAddress;
import java.net.Proxy;
import java.net.ProxySelector;
import java.net.SocketAddress;
import java.net.URI;
import java.net.UnknownHostException;
import java.util.List;
import java.util.Vector;
class URLProxySelector extends ProxySelector
{
/**
* @param p a proxy to add
*
*/
protected URLProxySelector(Proxy p)
{
super();
myproxies = new Vector();
if (p != null)
{
myproxies.add(p);
noproxies = new Vector();
noproxies.add(Proxy.NO_PROXY);
}
else
{
noproxies = myproxies;
}
myproxies.add(Proxy.NO_PROXY);
}
private final Vector myproxies;
private Vector noproxies;
/**
* @see java.net.ProxySelector#connectFailed(java.net.URI, java.net.SocketAddress, java.io.IOException)
* @param uri
* @param sa
* @param ioe
*/
@Override
public void connectFailed(URI uri, SocketAddress sa, IOException ioe)
{
//nop
}
/**
* @see java.net.ProxySelector#select(java.net.URI)
* @param uri
* @return
*/
@Override
public List select(URI uri)
{
String host = uri == null ? null : uri.getHost();
if (host != null)
host = host.toLowerCase();
boolean bLocal = false;
try
{
InetAddress a = InetAddress.getByName(host);
bLocal = a.isSiteLocalAddress();
}
catch (UnknownHostException x)
{
// nop
}
if (host == null || bLocal || "localhost".equals(host) || "127.0.0.1".equals(host))
return noproxies;
return myproxies;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy