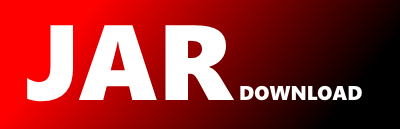
org.citrusframework.kafka.endpoint.KafkaEndpoint Maven / Gradle / Ivy
/*
* Copyright the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.citrusframework.kafka.endpoint;
import jakarta.annotation.Nullable;
import org.apache.commons.lang3.RandomStringUtils;
import org.citrusframework.actions.ReceiveMessageAction;
import org.citrusframework.common.ShutdownPhase;
import org.citrusframework.endpoint.AbstractEndpoint;
import java.time.Duration;
import static java.lang.Boolean.TRUE;
import static java.util.Objects.nonNull;
import static org.citrusframework.actions.ReceiveMessageAction.Builder.receive;
import static org.citrusframework.kafka.endpoint.selector.KafkaMessageByHeaderSelector.kafkaHeaderEquals;
import static org.citrusframework.kafka.message.KafkaMessageHeaders.KAFKA_PREFIX;
import static org.citrusframework.util.StringUtils.hasText;
/**
* Kafka message endpoint capable of sending/receiving messages from Kafka message destination.
* Either uses a Kafka connection factory or a Spring Kafka template to connect with Kafka
* destinations.
*
* @since 2.8
*/
public class KafkaEndpoint extends AbstractEndpoint implements ShutdownPhase {
/**
* Cached producer or consumer
*/
private @Nullable KafkaProducer kafkaProducer;
private @Nullable KafkaConsumer kafkaConsumer;
public static SimpleKafkaEndpointBuilder builder() {
return new SimpleKafkaEndpointBuilder();
}
/**
* Default constructor initializing endpoint configuration.
*/
public KafkaEndpoint() {
super(new KafkaEndpointConfiguration());
}
/**
* Constructor with endpoint configuration.
*/
public KafkaEndpoint(KafkaEndpointConfiguration endpointConfiguration) {
super(endpointConfiguration);
}
static KafkaEndpoint newKafkaEndpoint(
@Nullable org.apache.kafka.clients.consumer.KafkaConsumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy