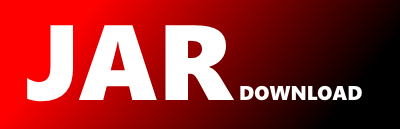
org.clapper.util.misc.ArrayIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javautil Show documentation
Show all versions of javautil Show documentation
A general-purpose Java utility library
The newest version!
package org.clapper.util.misc;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* The ArrayIterator class provides a bridge between an array of
* objects and an Iterator. It's useful in cases where you have an
* array, but you need an Iterator; using an instance of
* ArrayIterator saves copying the array's contents into a
* Collection, just to get an Iterator.
*
* @see java.util.Iterator
*/
public class ArrayIterator implements Iterator
{
/*----------------------------------------------------------------------*\
Private Data Elements
\*----------------------------------------------------------------------*/
/**
* The underlying Array.
*/
private T array[] = null;
/**
* The next array index.
*/
private int nextIndex = 0;
/*----------------------------------------------------------------------*\
Constructor
\*----------------------------------------------------------------------*/
/**
* Allocate a new ArrayIterator object that will
* iterate over the specified array of objects.
*
* @param array The array over which to iterate
*/
public ArrayIterator (T array[])
{
this.array = array;
}
/**
* Allocate a new ArrayIterator object that will iterate
* over the specified array of objects, starting at a particular index.
* The index isn't checked for validity until next() is called.
*
* @param array The array over which to iterate
* @param index The index at which to start
*/
public ArrayIterator (T array[], int index)
{
this.array = array;
this.nextIndex = index;
}
/*----------------------------------------------------------------------*\
Public Methods
\*----------------------------------------------------------------------*/
/**
* Get the index of the next element to be retrieved. This index value
* might be past the end of the array.
*
* @return the index
*/
public int getNextIndex()
{
return nextIndex;
}
/**
* Determine whether the underlying Iterator has more
* elements.
*
* @return true if and only if a call to
* next() will return an element,
* false otherwise.
*
* @see #next
*/
public boolean hasNext()
{
return (array != null) && (nextIndex < array.length);
}
/**
* Get the next element from the underlying array.
*
* @return the next element from the underlying array
*
* @exception java.util.NoSuchElementException
* No more elements exist
*
* @see #previous()
* @see java.util.Iterator#next
*/
public T next() throws NoSuchElementException
{
T result = null;
try
{
if (array == null)
throw new NoSuchElementException(); // NOPMD
result = array[nextIndex++];
}
catch (ArrayIndexOutOfBoundsException ex)
{
throw new NoSuchElementException(); // NOPMD
}
return result;
}
/**
* Get the previous element from the underlying array. This method
* decrements the iterator's internal index by one, and returns the
* corresponding element.
*
* @return the previous element from the underlying array
*
* @exception java.util.NoSuchElementException
* Attempt to move internal index before the first array element
*
* @see #next()
*/
public T previous() throws NoSuchElementException
{
T result = null;
try
{
result = array[--nextIndex];
}
catch (ArrayIndexOutOfBoundsException ex)
{
throw new NoSuchElementException(); // NOPMD
}
return result;
}
/**
* Required by the Iterator interface, but not supported by
* this class.
*
* @throws UnsupportedOperationException unconditionally
*/
public void remove()
{
throw new UnsupportedOperationException();
}
}