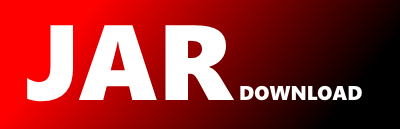
org.clapper.util.misc.SemaphoreException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javautil Show documentation
Show all versions of javautil Show documentation
A general-purpose Java utility library
The newest version!
package org.clapper.util.misc;
/**
* Thrown by classes that implement the {@link Semaphore} interface, to
* indicate an error.
*
* @deprecated J2SE 5.0 now provides a java.util.concurrent.Semaphore class
*/
public class SemaphoreException extends NestedException
{
/*----------------------------------------------------------------------*\
Private Static Variables
\*----------------------------------------------------------------------*/
/**
* See JDK 1.5 version of java.io.Serializable
*/
private static final long serialVersionUID = 1L;
/*----------------------------------------------------------------------*\
Constructors
\*----------------------------------------------------------------------*/
/**
* Default constructor, for an exception with no nested exception and
* no message.
*/
public SemaphoreException()
{
super();
}
/**
* Constructs an exception containing another exception, but no message
* of its own.
*
* @param exception the exception to contain
*/
public SemaphoreException (Throwable exception)
{
super (exception);
}
/**
* Constructs an exception containing an error message, but no
* nested exception.
*
* @param message the message to associate with this exception
*/
public SemaphoreException (String message)
{
super (message);
}
/**
* Constructs an exception containing another exception and a message.
*
* @param message the message to associate with this exception
* @param exception the exception to contain
*/
public SemaphoreException (String message, Throwable exception)
{
super (message, exception);
}
/**
* Constructs an exception containing a resource bundle name, a message
* key, and a default message (in case the resource bundle can't be
* found). Using this constructor is equivalent to calling the
* {@link #SemaphoreException(String,String,String,Object[])} constructor,
* with a null pointer for the Object[] parameter.
* Calls to {@link NestedException#getMessage(Locale)} will attempt to
* retrieve the top-most message (i.e., the message from this exception,
* not from nested exceptions) by querying the named resource bundle.
* Calls to {@link NestedException#printStackTrace(PrintWriter,Locale)}
* will do the same, where applicable. The message is not retrieved
* until one of those methods is called, because the desired locale is
* passed into getMessage() and printStackTrace(),
* not this constructor.
*
* @param bundleName resource bundle name
* @param messageKey the key to the message to find in the bundle
* @param defaultMsg the default message
*
* @see #SemaphoreException(String,String,String,Object[])
* @see NestedException#getLocalizedMessage
*/
public SemaphoreException (String bundleName,
String messageKey,
String defaultMsg)
{
super (bundleName, messageKey, defaultMsg);
}
/**
* Constructs an exception containing a resource bundle name, a message
* key, and a default message (in case the resource bundle can't be
* found). Using this constructor is equivalent to calling the
* {@link #SemaphoreException(String,String,String,Object[])} constructor,
* with a null pointer for the Object[] parameter.
* Calls to {@link NestedException#getMessage(Locale)} will attempt to
* retrieve the top-most message (i.e., the message from this exception,
* not from nested exceptions) by querying the named resource bundle.
* Calls to {@link NestedException#printStackTrace(PrintWriter,Locale)}
* will do the same, where applicable. The message is not retrieved
* until one of those methods is called, because the desired locale is
* passed into getMessage() and printStackTrace(),
* not this constructor.
*
* @param bundleName resource bundle name
* @param messageKey the key to the message to find in the bundle
* @param defaultMsg the default message
* @param msgParams parameters to the message, if any, or null
*
* @see #SemaphoreException(String,String,String,Object[])
* @see NestedException#getLocalizedMessage
*/
public SemaphoreException (String bundleName,
String messageKey,
String defaultMsg,
Object[] msgParams)
{
super (bundleName, messageKey, defaultMsg, msgParams);
}
/**
* Constructs an exception containing a resource bundle name, a message
* key, a default message (in case the resource bundle can't be found),
* and another exception. Using this constructor is equivalent to
* calling the {@link #SemaphoreException(String,String,String,Object[])}
* constructor, with a null pointer for the Object[]
* parameter. Calls to {@link #getMessage(Locale)} will attempt to
* retrieve the top-most message (i.e., the message from this
* exception, not from nested exceptions) by querying the named
* resource bundle. Calls to
* {@link #printStackTrace(PrintWriter,Locale)} will do the same, where
* applicable. The message is not retrieved until one of those methods
* is called, because the desired locale is passed into
* getMessage() and printStackTrace(), not this
* constructor.
*
* @param bundleName resource bundle name
* @param messageKey the key to the message to find in the bundle
* @param defaultMsg the default message
* @param exception the exception to nest
*
* @see #SemaphoreException(String,String,String,Object[])
* @see NestedException#getMessage(Locale)
*/
public SemaphoreException (String bundleName,
String messageKey,
String defaultMsg,
Throwable exception)
{
this (bundleName, messageKey, defaultMsg, null, exception);
}
/**
* Constructs an exception containing a resource bundle name, a message
* key, a default message format (in case the resource bundle can't be
* found), arguments to be incorporated in the message via
* java.text.MessageFormat, and another exception.
* Calls to {@link #getMessage(Locale)} will attempt to retrieve the
* top-most message (i.e., the message from this exception, not from
* nested exceptions) by querying the named resource bundle. Calls to
* {@link #printStackTrace(PrintWriter,Locale)} will do the same, where
* applicable. The message is not retrieved until one of those methods
* is called, because the desired locale is passed into
* getMessage() and printStackTrace(), not this
* constructor.
*
* @param bundleName resource bundle name
* @param messageKey the key to the message to find in the bundle
* @param defaultMsg the default message
* @param msgParams parameters to the message, if any, or null
* @param exception exception to be nested
*
* @see #SemaphoreException(String,String,String,Object[])
* @see NestedException#getMessage(Locale)
*/
public SemaphoreException (String bundleName,
String messageKey,
String defaultMsg,
Object[] msgParams,
Throwable exception)
{
super (bundleName, messageKey, defaultMsg, msgParams, exception);
}
}