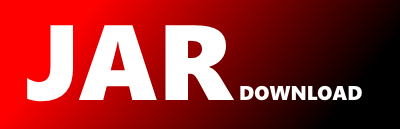
org.cleartk.classifier.CleartkAnnotatorDescriptionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cleartk-ml Show documentation
Show all versions of cleartk-ml Show documentation
ClearTK machine learning library and interfaces
/**
* Copyright (c) 2009, Regents of the University of Colorado
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
* Neither the name of the University of Colorado at Boulder nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package org.cleartk.classifier;
import org.apache.uima.analysis_engine.AnalysisEngineDescription;
import org.apache.uima.resource.ResourceInitializationException;
import org.apache.uima.resource.metadata.TypeSystemDescription;
import org.cleartk.classifier.jar.DirectoryDataWriterFactory;
import org.cleartk.classifier.jar.GenericJarClassifierFactory;
import org.cleartk.classifier.viterbi.DefaultOutcomeFeatureExtractor;
import org.cleartk.classifier.viterbi.ViterbiClassifier;
import org.cleartk.classifier.viterbi.ViterbiDataWriterFactory;
import org.uimafit.factory.AnalysisEngineFactory;
import org.uimafit.factory.ConfigurationParameterFactory;
/**
* A factory class that simplifies the creation of descriptors for {@link CleartkAnnotator} and
* {@link CleartkSequenceAnnotator} classes. The factory methods here wrap common patterns of calls
* to {@link AnalysisEngineFactory#createPrimitiveDescription} and
* {@link ConfigurationParameterFactory#addConfigurationParameter} for setting classifier classes,
* output directories, etc. that are necessary when creating a CleartkAnnotator or
* CleartkSequenceAnnotator.
*
*
* Copyright (c) 2009, Regents of the University of Colorado
* All rights reserved.
*
* @author Steven Bethard
* @deprecated Use {@link AnalysisEngineFactory} directly.
*/
@Deprecated
public class CleartkAnnotatorDescriptionFactory {
/**
* Create an {@link AnalysisEngineDescription} for using a {@link CleartkSequenceAnnotator} to
* write training data for a {@link ViterbiClassifier}. Note that data is written with a
* {@link DataWriterFactory}, not a {@link SequenceDataWriterFactory} - A ViterbiClassifier wraps
* a non-sequence classifier to work as a sequence classifier.
*
* @param
* The outcome type of the classifier (must be the same for both the
* CleartkSequenceAnnotator and the DataWriterFactory).
* @param annotatorClass
* The main annotator for the AnalysisEngineDescription.
* @param typeSystemDescription
* The type system used by the annotator.
* @param delegatedDataWriterFactoryClass
* The non-sequence DataWriterFactory that will be used to write the training data (and
* indicate the type of classifier that will be trained from this data).
* @param outputDir
* The directory where the training data should be written.
* @return An AnalysisEngineDescription for the CleartkSequenceAnnotator.
* @deprecated Use {@link AnalysisEngineFactory} directly.
*/
@Deprecated
public static AnalysisEngineDescription createViterbiAnnotator(
Class extends CleartkSequenceAnnotator> annotatorClass,
TypeSystemDescription typeSystemDescription,
Class extends DataWriterFactory> delegatedDataWriterFactoryClass,
String outputDir) throws ResourceInitializationException {
return AnalysisEngineFactory.createPrimitiveDescription(
annotatorClass,
typeSystemDescription,
CleartkSequenceAnnotator.PARAM_DATA_WRITER_FACTORY_CLASS_NAME,
ViterbiDataWriterFactory.class.getName(),
DirectoryDataWriterFactory.PARAM_OUTPUT_DIRECTORY,
outputDir,
ViterbiDataWriterFactory.PARAM_DELEGATED_DATA_WRITER_FACTORY_CLASS,
delegatedDataWriterFactoryClass.getName(),
ViterbiDataWriterFactory.PARAM_OUTCOME_FEATURE_EXTRACTOR_NAMES,
new String[] { DefaultOutcomeFeatureExtractor.class.getName() });
}
/**
* Create an {@link AnalysisEngineDescription} for using a {@link CleartkAnnotator} to create new
* annotations based on the predictions of a classifier.
*
* @param cleartkAnnotatorClass
* The main annotator for the AnalysisEngineDescription.
* @param typeSystemDescription
* The type system used by the annotator.
* @param classifierJar
* The jar file containing the classifier that will make predictions.
* @return An AnalysisEngineDescription for the CleartkAnnotator.
* @deprecated Use {@link AnalysisEngineFactory} directly.
*/
@Deprecated
public static AnalysisEngineDescription createCleartkAnnotator(
Class extends CleartkAnnotator>> cleartkAnnotatorClass,
TypeSystemDescription typeSystemDescription,
String classifierJar) throws ResourceInitializationException {
AnalysisEngineDescription aed = AnalysisEngineFactory.createPrimitiveDescription(
cleartkAnnotatorClass,
typeSystemDescription);
ConfigurationParameterFactory.addConfigurationParameter(
aed,
GenericJarClassifierFactory.PARAM_CLASSIFIER_JAR_PATH,
classifierJar);
return aed;
}
/**
* Create an {@link AnalysisEngineDescription} for using a {@link CleartkAnnotator} to write
* training data.
*
* @param
* The outcome type of the classifier (must be the same for both the CleartkAnnotator and
* the DataWriterFactory).
* @param cleartkAnnotatorClass
* The main annotator for the AnalysisEngineDescription.
* @param typeSystemDescription
* The type system used by the annotator.
* @param dataWriterFactoryClass
* The DataWriterFactory that will be used to write the training data (and indicate the
* type of classifier that will be trained from this data).
* @param outputDir
* The directory where the training data should be written.
* @return An AnalysisEngineDescription for the CleartkAnnotator.
* @deprecated Use {@link AnalysisEngineFactory} directly.
*/
@Deprecated
public static AnalysisEngineDescription createCleartkAnnotator(
Class extends CleartkAnnotator> cleartkAnnotatorClass,
TypeSystemDescription typeSystemDescription,
Class extends DataWriterFactory> dataWriterFactoryClass,
String outputDir) throws ResourceInitializationException {
return AnalysisEngineFactory.createPrimitiveDescription(
cleartkAnnotatorClass,
typeSystemDescription,
CleartkAnnotator.PARAM_DATA_WRITER_FACTORY_CLASS_NAME,
dataWriterFactoryClass.getName(),
DirectoryDataWriterFactory.PARAM_OUTPUT_DIRECTORY,
outputDir);
}
/**
* Create an {@link AnalysisEngineDescription} for using a {@link CleartkSequenceAnnotator} to
* create new annotations based on the predictions of a classifier.
*
* @param sequenceClassifierAnnotatorClass
* The main annotator for the AnalysisEngineDescription.
* @param typeSystemDescription
* The type system used by the annotator.
* @param classifierJar
* The jar file containing the classifier that will make predictions.
* @return An AnalysisEngineDescription for the CleartkSequenceAnnotator.
* @deprecated Use {@link AnalysisEngineFactory} directly.
*/
@Deprecated
public static AnalysisEngineDescription createCleartkSequenceAnnotator(
Class extends CleartkSequenceAnnotator>> sequenceClassifierAnnotatorClass,
TypeSystemDescription typeSystemDescription,
String classifierJar) throws ResourceInitializationException {
AnalysisEngineDescription aed = AnalysisEngineFactory.createPrimitiveDescription(
sequenceClassifierAnnotatorClass,
typeSystemDescription);
ConfigurationParameterFactory.addConfigurationParameter(
aed,
GenericJarClassifierFactory.PARAM_CLASSIFIER_JAR_PATH,
classifierJar);
return aed;
}
/**
* Create an {@link AnalysisEngineDescription} for using a {@link CleartkSequenceAnnotator} to
* write training data.
*
* @param
* The outcome type of the classifier (must be the same for both the
* CleartkSequenceAnnotator and the SequenceDataWriterFactory).
* @param sequenceClassifierAnnotatorClass
* The main annotator for the AnalysisEngineDescription.
* @param typeSystemDescription
* The type system used by the annotator.
* @param dataWriterFactoryClass
* The SequenceDataWriterFactory that will be used to write the training data (and
* indicate the type of classifier that will be trained from this data).
* @param outputDir
* The directory where the training data should be written.
* @return An AnalysisEngineDescription for the CleartkSequenceAnnotator.
* @deprecated Use {@link AnalysisEngineFactory} directly.
*/
@Deprecated
public static AnalysisEngineDescription createCleartkSequenceAnnotator(
Class extends CleartkSequenceAnnotator> sequenceClassifierAnnotatorClass,
TypeSystemDescription typeSystemDescription,
Class extends SequenceDataWriterFactory> dataWriterFactoryClass,
String outputDir) throws ResourceInitializationException {
return AnalysisEngineFactory.createPrimitiveDescription(
sequenceClassifierAnnotatorClass,
typeSystemDescription,
CleartkSequenceAnnotator.PARAM_DATA_WRITER_FACTORY_CLASS_NAME,
dataWriterFactoryClass.getName(),
DirectoryDataWriterFactory.PARAM_OUTPUT_DIRECTORY,
outputDir);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy