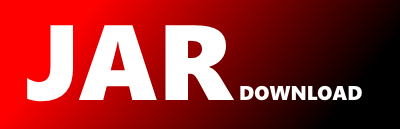
org.cleartk.classifier.viterbi.ViterbiClassifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cleartk-ml Show documentation
Show all versions of cleartk-ml Show documentation
ClearTK machine learning library and interfaces
/**
* Copyright (c) 2007-2008, Regents of the University of Colorado
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
* Neither the name of the University of Colorado at Boulder nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package org.cleartk.classifier.viterbi;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.uima.UimaContext;
import org.apache.uima.resource.ResourceInitializationException;
import org.cleartk.classifier.Classifier;
import org.cleartk.classifier.CleartkProcessingException;
import org.cleartk.classifier.Feature;
import org.cleartk.classifier.ScoredOutcome;
import org.cleartk.classifier.SequenceClassifier;
import org.cleartk.util.CleartkInitializationException;
import org.cleartk.util.ReflectionUtil;
import org.cleartk.util.ReflectionUtil.TypeArgumentDelegator;
import org.uimafit.component.initialize.ConfigurationParameterInitializer;
import org.uimafit.descriptor.ConfigurationParameter;
import org.uimafit.factory.ConfigurationParameterFactory;
import org.uimafit.factory.initializable.Initializable;
/**
*
* Copyright (c) 2007-2008, Regents of the University of Colorado
* All rights reserved.
*/
public class ViterbiClassifier implements SequenceClassifier,
Initializable, TypeArgumentDelegator {
protected Classifier delegatedClassifier;
protected OutcomeFeatureExtractor[] outcomeFeatureExtractors;
public static final String PARAM_STACK_SIZE = ConfigurationParameterFactory.createConfigurationParameterName(
ViterbiClassifier.class,
"stackSize");
@ConfigurationParameter(
description = "specifies the maximum number of candidate paths to "
+ "keep track of. In general, this number should be higher than the number "
+ "of possible classifications at any given point in the sequence. This "
+ "guarantees that highest-possible scoring sequence will be returned. If, "
+ "however, the number of possible classifications is quite high and/or you "
+ "are concerned about throughput performance, then you may want to reduce the number "
+ "of candidate paths to maintain. If Classifier.score is not implemented for the given delegated classifier, then "
+ "the value of this parameter must be 1. ",
defaultValue = "1")
protected int stackSize;
public static final String PARAM_ADD_SCORES = ConfigurationParameterFactory.createConfigurationParameterName(
ViterbiClassifier.class,
"addScores");
@ConfigurationParameter(
description = "specifies whether the scores of candidate sequence classifications should be "
+ "calculated by summing classfication scores for each member of the sequence or by multiplying them. A value of "
+ "true means that the scores will be summed. A value of false means that the scores will be multiplied. ",
defaultValue = "false")
protected boolean addScores = false;
public ViterbiClassifier(
Classifier delegatedClassifier,
OutcomeFeatureExtractor[] outcomeFeatureExtractors) {
this.delegatedClassifier = delegatedClassifier;
this.outcomeFeatureExtractors = outcomeFeatureExtractors;
}
public void initialize(UimaContext context) throws ResourceInitializationException {
ConfigurationParameterInitializer.initialize(this, context);
if (stackSize < 1) {
throw CleartkInitializationException.parameterLessThan(PARAM_STACK_SIZE, 1, stackSize);
}
}
public List classify(List> features)
throws CleartkProcessingException {
if (stackSize == 1) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy