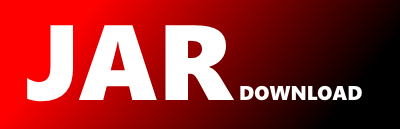
clojure.java.api.package.html Maven / Gradle / Ivy
Clojure interop from Java.
The clojure.java.api package provides a minimal interface to bootstrap
Clojure access from other JVM languages. It does this by providing:
- The ability to use Clojure's namespaces to locate an arbitrary
var, returning the
var's
clojure.lang.IFn
interface.
- A convenience method
read
for reading data using
Clojure's edn reader
IFn
s provide complete access to
Clojure's APIs.
You can also access any other library written in Clojure, after adding
either its source or compiled form to the classpath.
The public Java API for Clojure consists of the following classes
and interfaces:
- clojure.java.api.Clojure
- clojure.lang.IFn
All other Java classes should be treated as implementation details,
and applications should avoid relying on them.
To lookup and call a Clojure function:
IFn plus = Clojure.var("clojure.core", "+");
plus.invoke(1, 2);
Functions in clojure.core
are automatically loaded. Other
namespaces can be loaded via require
:
IFn require = Clojure.var("clojure.core", "require");
require.invoke(Clojure.read("clojure.set"));
IFn
s can be passed to higher order functions, e.g. the
example below passes inc
to map
:
IFn map = Clojure.var("clojure.core", "map");
IFn inc = Clojure.var("clojure.core", "inc");
map.invoke(inc, Clojure.read("[1 2 3]"));
Most IFns in Clojure refer to functions. A few, however, refer to
non-function data values. To access these, use deref
instead of fn
:
IFn printLength = Clojure.var("clojure.core", "*print-length*");
IFn deref = Clojure.var("clojure.core", "deref");
deref.invoke(printLength);