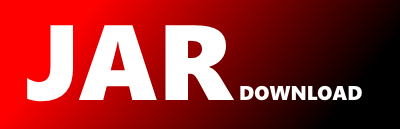
com.cloudbees.sdk.commands.config.ConfigParametersBase Maven / Gradle / Ivy
/*
* Copyright 2010-2013, CloudBees Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cloudbees.sdk.commands.config;
import com.cloudbees.sdk.commands.Command;
import com.cloudbees.sdk.commands.config.model.ConfigParameters;
import com.cloudbees.sdk.commands.config.model.ParameterSettings;
import com.cloudbees.sdk.utils.Helper;
import java.io.IOException;
/**
* @author Fabian Donze
*/
public abstract class ConfigParametersBase extends Command {
private Boolean global;
/**
* The id of the application.
*/
private String appid;
private String account;
public void setAppid(String appid) {
if (global == null) {
this.appid = appid;
global = Boolean.FALSE;
}
}
public void setAccount(String account) {
this.account = account;
global = Boolean.TRUE;
}
public void setGlobal(Boolean global) {
this.global = global;
}
protected boolean isGlobal(String action) throws IOException {
if (global == null) {
global = !Helper.promptMatches("Do you want to " + action + " global or application parameters: (g/a) ", "[aA].*");
}
return global;
}
@Override
protected boolean postParseCommandLine() {
if (!super.postParseCommandLine()) return false;
if (getParameters().size() > 0 && appid == null) {
String str = getParameters().get(0);
if (isParameter(str) < 0)
setAppid(str);
}
return true;
}
protected String getAccount() throws IOException {
if (account == null) account = getConfigProperties().getProperty("bees.project.app.domain");
if (account == null) account = Helper.promptFor("Account name: ", true);
return account;
}
protected boolean isAppIdSet() {
return appid != null;
}
protected String getAppId() throws IOException
{
if (appid == null || appid.equals("")) {
appid = AppHelper.getArchiveApplicationId();
}
if (appid == null || appid.equals(""))
appid = Helper.promptForAppId();
if (appid == null || appid.equals(""))
throw new IllegalArgumentException("No application id specified");
String[] appIdParts = appid.split("/");
if (appIdParts.length < 2) {
String defaultAppDomain = getAccount();
if (defaultAppDomain != null && !defaultAppDomain.equals("")) {
appid = defaultAppDomain + "/" + appid;
} else {
throw new RuntimeException("default app account could not be determined, appid needs to be fully-qualified ");
}
}
return appid;
}
@Override
protected boolean preParseCommandLine() {
addOption( "a", "appid", true, "CloudBees application ID");
addOption( "ac", "account", true, "CloudBees account name" );
addOption( "g", "global", false, "Global account parameters" );
return true;
}
@Override
protected String getUsageMessage() {
return "";
}
protected void printConfigParameters(ConfigParameters configParameters) {
if (isTextOutput()) {
if (configParameters.getEnvironments().size() > 0 || configParameters.getResources().size() > 0) {
System.out.println(configParameters.toXML());
} else {
// System.out.println("CONFIG PARAMETERS");
if (configParameters.getParameters().size() > 0) {
System.out.println("Application Parameters:");
for (ParameterSettings parameterSettings : configParameters.getParameters()) {
System.out.println(" " + parameterSettings.getName() + "=" + parameterSettings.getValue());
}
}
if (configParameters.getRuntimeParameters().size() > 0) {
System.out.println("Runtime Parameters:");
for (ParameterSettings parameterSettings : configParameters.getRuntimeParameters()) {
System.out.println(" " + parameterSettings.getName() + "=" + parameterSettings.getValue());
}
}
}
} else {
printOutput(configParameters, ConfigParameters.class);
}
}
protected void displayStatus(String resourceType, String resourceId, String status) {
if (resourceType == null || resourceType.length() == 0)
resourceType = "Unknown";
else
resourceType = Character.toUpperCase(resourceType.charAt(0)) + resourceType.substring(1);
System.out.println(resourceType + " config parameters for " + resourceId + ": " + status);
System.out.println();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy