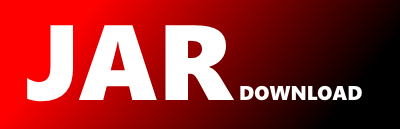
com.cloudbees.sdk.commands.config.ConfigParametersSet Maven / Gradle / Ivy
/*
* Copyright 2010-2013, CloudBees Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cloudbees.sdk.commands.config;
import com.cloudbees.api.BeesClient;
import com.cloudbees.api.ConfigurationParametersResponse;
import com.cloudbees.api.ConfigurationParametersUpdateResponse;
import com.cloudbees.sdk.cli.BeesCommand;
import com.cloudbees.sdk.cli.CLICommand;
import com.cloudbees.sdk.commands.config.model.ConfigParameters;
import com.cloudbees.sdk.commands.config.model.Environment;
import com.cloudbees.sdk.commands.config.model.ParameterSettings;
import com.cloudbees.sdk.commands.config.model.ResourceSettings;
import java.io.File;
import java.io.FileWriter;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @author Fabian Donze
*/
@BeesCommand(group="Configuration")
@CLICommand("config:set")
public class ConfigParametersSet extends ConfigParametersBase {
private String environment;
private String name;
private String type;
private Map runtimeParameters = new HashMap();
public ConfigParametersSet() {
}
public String getEnvironment() {
return environment;
}
public void setEnvironment(String environment) {
this.environment = environment;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public void setR(String rt) {
rt = rt.trim();
int idx = isParameter(rt);
if (idx > 0) {
runtimeParameters.put(rt.substring(0, idx), rt.substring(idx + 1));
}
}
public void setP(String rt) {
rt = rt.trim();
int idx = isParameter(rt);
if (idx > 0) {
addParameter(rt);
}
}
protected Map getRuntimeParameters() {
return runtimeParameters;
}
@Override
protected boolean preParseCommandLine() {
if (super.preParseCommandLine()) {
addOption( "n", "name", true, "Optional resource name to group parameters under one resource", true );
addOption( "e", "environment", true, "Optional environment scope (only for account parameters)", true);
addOption( "t", "type", true, "Optional resource type [jndi-env[:java_class_type] | system-property | context-param]", true);
addOption( "P", null, true, "Application config parameter name=value" );
addOption( "R", null, true, "Runtime config parameter name=value" );
return true;
}
return false;
}
@Override
protected boolean execute() throws Exception {
BeesClient client = getBeesClient(BeesClient.class);
String resourceId;
String resourceType;
if (isGlobal("set")) {
resourceId = getAccount();
resourceType = "global";
} else {
resourceId = getAppId();
resourceType = "application";
}
ConfigurationParametersResponse res = client.configurationParameters(resourceId, resourceType);
ConfigParameters configParameters = ConfigParameters.parse(res.getConfiguration());
List otherArgs = getParameters();
if (getName() != null) {
ResourceSettings resource = configParameters.getResource(getName());
if (resource == null) resource = new ResourceSettings(getName(), type);
for (String str : otherArgs) {
int idx = isParameter(str);
if (idx > 0) {
resource.setParameter(str.substring(0, idx), str.substring(idx + 1));
}
}
if (resource.getParameters().size() > 0) {
setResource(configParameters, resource, resourceType);
}
} else {
for (String str : otherArgs) {
int idx = isParameter(str);
if (idx > 0) {
if (getType() != null) {
ResourceSettings resource = new ResourceSettings(str.substring(0, idx), getType(), str.substring(idx + 1));
setResource(configParameters, resource, resourceType);
} else {
ParameterSettings parameter = new ParameterSettings(str.substring(0, idx), str.substring(idx + 1));
setParameter(configParameters, parameter, resourceType);
}
}
}
for (Map.Entry entry: getRuntimeParameters().entrySet()) {
ParameterSettings parameter = new ParameterSettings(entry.getKey(), entry.getValue());
setRuntimeParameter(configParameters, parameter, resourceType);
}
}
File xmlFile = File.createTempFile("conf", "xml");
xmlFile.deleteOnExit();
FileWriter fos = null;
try {
fos = new FileWriter(xmlFile);
fos.write(configParameters.toXML());
} finally {
if (fos != null)
fos.close();
}
ConfigurationParametersUpdateResponse res2 = client.configurationParametersUpdate(resourceId, resourceType, xmlFile);
if (isTextOutput()) {
displayStatus(resourceType, resourceId, res2.getStatus());
} else {
printOutput(res2, ConfigurationParametersUpdateResponse.class);
}
printConfigParameters(configParameters);
return true;
}
private void setResource(ConfigParameters configParameters, ResourceSettings resource, String resourceType) {
if (getEnvironment() != null && !resourceType.equalsIgnoreCase("application")) {
Environment environment = configParameters.getEnvironment(getEnvironment());
if (environment == null) {
environment = new Environment(getEnvironment());
configParameters.setEnvironment(environment);
}
environment.setResource(resource);
} else {
configParameters.setResource(resource);
}
}
private void setParameter(ConfigParameters configParameters, ParameterSettings parameter, String resourceType) {
if (getEnvironment() != null && !resourceType.equalsIgnoreCase("application")) {
Environment environment = configParameters.getEnvironment(getEnvironment());
if (environment == null) {
environment = new Environment(getEnvironment());
configParameters.setEnvironment(environment);
}
environment.setParameter(parameter);
} else {
configParameters.setParameter(parameter);
}
}
private void setRuntimeParameter(ConfigParameters configParameters, ParameterSettings parameter, String resourceType) {
if (getEnvironment() != null && !resourceType.equalsIgnoreCase("application")) {
Environment environment = configParameters.getEnvironment(getEnvironment());
if (environment == null) {
environment = new Environment(getEnvironment());
configParameters.setEnvironment(environment);
}
environment.setRuntimeParameter(parameter);
} else {
configParameters.setRuntimeParameter(parameter);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy