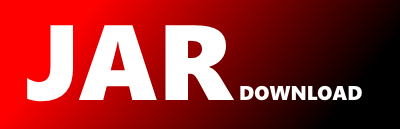
org.cloudfoundry.identity.uaa.user.UserInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudfoundry-identity-server Show documentation
Show all versions of cloudfoundry-identity-server Show documentation
Cloud Foundry User Account and Authentication
The newest version!
/*
* ****************************************************************************
* Cloud Foundry
* Copyright (c) [2009-2016] Pivotal Software, Inc. All Rights Reserved.
*
* This product is licensed to you under the Apache License, Version 2.0 (the "License").
* You may not use this product except in compliance with the License.
*
* This product includes a number of subcomponents with
* separate copyright notices and license terms. Your use of these
* subcomponents is subject to the terms and conditions of the
* subcomponent's license, as noted in the LICENSE file.
* ****************************************************************************
*/
package org.cloudfoundry.identity.uaa.user;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import java.util.List;
public class UserInfo {
@JsonProperty("roles")
private List roles;
@JsonProperty("user_attributes")
private LinkedMultiValueMap userAttributes;
public UserInfo(){}
@JsonIgnore
public UserInfo setRoles(List roles) {
this.roles = roles;
return this;
}
@JsonIgnore
public List getRoles() {
return roles;
}
@JsonIgnore
public UserInfo setUserAttributes(MultiValueMap userAttributes) {
this.userAttributes = new LinkedMultiValueMap<>(userAttributes);
return this;
}
@JsonIgnore
public MultiValueMap getUserAttributes() {
return userAttributes;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof UserInfo)) return false;
UserInfo userInfo = (UserInfo) o;
if (!compareRoles(getRoles(), ((UserInfo) o).getRoles())) return false;
return getUserAttributes() != null ? getUserAttributes().equals(userInfo.getUserAttributes()) : userInfo.getUserAttributes() == null;
}
protected boolean compareRoles(List l1, List l2) {
if (l1==null && l2==null) {
return true;
} else if (l1==null || l2==null) {
return false;
}
return l1.containsAll(l2) && l2.containsAll(l1);
}
@Override
public int hashCode() {
int result = getRoles() != null ? getRoles().hashCode() : 0;
result = 31 * result + (getUserAttributes() != null ? getUserAttributes().hashCode() : 0);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy